Musimatic Blog
Completion Of Phase 5 Project For Flatiron School Software Development Bootcamp
I have completed my Phase 5 project which can be found deployed here:
The related GitHub repo that's part of this project can be found here:
This is a full stack web application that is written with React for its frontend, and has a Ruby On Rails with Postgresql database backend. It can be deployed locally by using the following set of commands:
NOTE:
- You need to have 'postgresql' 15 installed on your local machine, so search online how to do this first before proceeding.
Then, run this command to install the project's components:
npm install
npm install --prefix client
bundle install
rails db:create
rails db:migrate
Its Rails backend can then be run locally with the following Bash command within the root of the project directory:
sudo service postgresql start
rails s
Its React frontend can be run locally with the following Bash command within the root of the project directory:
npm start --prefix client
The major goal of this project is to allow a user to create a party, add items to that party, add a location to that party, and also view all parties on its summary page. Each of the previously mentioned components are crud compatible meaning I can edit, and delete them via frontend actions to the backend database.
The 'View All Parties' or 'Summary' page features the Google Maps API in two ways. The data from all parties is aggregated via a fetch call made in the child 'Summary' component:
useEffect(() => {
fetch("/parties", {
method: "GET",
headers: {
"Content-Type": "application/json",
"Accept": "application/json",
},
})
.then((response) => response.json())
.then((data) => {
onFetchSummaryParties(data);
});
}, []);
The corresponding 'onFetchSummaryParties' function found within the parent App.js component then obtains the data in an asynchronous way:
async function handleFetchSummaryParties(fetchedParties) {
// Loop through each party and check to see if it has a location
// If it has a location, then run the 'get_coordinates' function to its actual 'lat' and 'lng' values accordingly so that we can
// later use them for the map on the summary page:
const promises = fetchedParties.map(async (party) => {
if (party.location) {
let position = await get_coordinates(party.location.name);
return {...party, location: {...party.location, position: position}};
}
else {
return party;
}
});
const modifiedParties = await Promise.all( promises );
setParties(modifiedParties);
}
The 'onFetchSummaryParties' function then calls the more specific function that is responsible for making the calls to the 'Geocoder' library which is the 'get_coordinates' function found within the parent App.js component. this function utilizes the 'process.env' method of retrieving the API key from the React frontend, which is fine since there is no other way to really secure the Google Maps API key as it is meant for the React frontend to directly access this information. It also then then goes through each of these parties and uses a process called 'Geocoding' via the 'Geocoder' library found within the Google Maps API to take a city address, and converts it into 'longitude' and 'latitude' points to be used by 'markers' later on:
async function get_coordinates(name) {
Geocode.setApiKey(process.env.REACT_APP_GOOGLE_API_KEY);
Geocode.setLanguage("en");
Geocode.setLocationType("ROOFTOP");
return await Geocode.fromAddress(name).then(
(response) => {
const { lat, lng } = response.results[0].geometry.location;
let position = { lat: lat, lng: lng }
// Related Stackoverflow post:
// https://stackoverflow.com/questions/38884522/why-is-my-asynchronous-function-returning-promise-pending-instead-of-a-val
return position
},
(error) => {
console.error(error);
}
).then((response) => {
return response;
})}
Within the child Summary component, each of the data sections are mapped through in order to create a list of markers, which are really just typical map pins, but relabeled to suit Google's naming preference. Here is the related section that is in the middle of the 'Summary.js' child component, and is way after the initial declaration of the 'markers' array:
if (party.location) {
if (Object.keys(party.location).length > 0) {
partyLocation = (
<li key={party.location.id}>{party.location.name}</li>
)
// Create new 'marker' object for the map here
// Provide the 'party.location.id' as the 'id' value
// Provide the 'party.location.name' for the 'name' value
// Use the 'Geocoder' fetch call to obtain the correct longitude and latitude results for each of the locations --> This might be tricky since they might not be received in time
let newMarker = { id: party.location.id, name: party.name + ": " + party.location.name, position: party.location.position }
markers.push(newMarker);
}
}
This is then rendered to the user as pins on the map that can be clicked to reveal the given party's information.
I also used the 'useContext' hook in order to create a simple 'hello' message. I started this process by creating a related context file called 'HelloContext.js' in the 'context' directory with the following:
import React, { createContext } from "react";
const HelloContext = createContext();
function HelloProvider({children}) {
return (
<HelloContext.Provider value="Hello">
{children}
</HelloContext.Provider>
)
}
export { HelloContext, HelloProvider }
I then imported the context provider within the App.js component:
import { HelloProvider } from "./context/HelloContext";
I then wrapped the context around the desired routing section for the 'About' component in the parent App.js component. I also passed down the 'user' as a separate 'props' value as well:
<Route
path="/"
element={
<HelloProvider>
<About user={user}/>
</HelloProvider>
}
/>
I then was able to utilize the context by simply creating a header on the 'About' page that contained the user's username:
import React, { useState, useContext, createContext } from "react";
import { HelloContext } from "./context/HelloContext";
function About({ user }) {
const value = useContext(HelloContext);
return (
<div>
<h2>About Page</h2>
<h3>{value}, {user.username}!</h3>
And that is my Phase 5 project.
Completion Of Phase 4 For Flatiron School Software Development Bootcamp
The Phase 4 section featured the 'Ruby On Rails' framework, and more specifically its ability to utilize 'Active Record' which can be found here as this guide helps explain this in more detail than I ever could:
'Rails', as it is commonly known, helps take the edge of from having to handle a lot of the 'backend' work that's required for a full stack web application. Remember, when someone mentions the 'frontend' of a 'full stack' web application, this means that they are referring to the user facing portion. Ex: Think of a typical modern website with forms, menus, etc. The 'backend' in this scenario handles all of the data fetch calls from the frontend. Ex: "I submitted this form with all of my user data and want to have something happen in the app". The 'Rails' backend in this scenario interprets what to do with this frontend data depending on the type of fetch call being made.
The process of how Rails handles routing for specific methods involves a few moving parts, so one related topic I would like to highlight in this blog post is the idea of how to use the 'Controller' methods provided by Rails. This to me is the heavy hitter reason why you would want to use 'Rails' in the first place since the 'Rails' framework dictates specific functions that can be used within the 'Controller' to help make your life easier. Reason being is that if you took the time to figure out what 'models' you want to be present in your app via the related 'migrations' you created that correspond to related database tables, then you will want some kind of easy way to access methods that interact with this data. The Rails specific methods for a 'Controller' allow you to interact with the project's 'model' data in more saner ways. Otherwise, you would be left to figure out the related SQL calls to your application's database on your own which is rarely fun in the first place. The fact that Rails does all of this SQL legwork for you is what makes it pretty awesome to use.
When you install Rails, you have the ability to use its associated bash terminal commands like the 'rails g' command in order to 'generate' certain project components for you. The 'rails g' command is basically equivalent to "Hey Rails, you go ahead and 'generate' something for me and place it in the correct directory structure as well." The beauty of this is that Rails will generate a project component for you, and magically place it in the correct directory structure of your project.
For example, we can create a new 'Controller' with Rails to interact with our 'cookouts' database table via the following command, NOTE: I used the '–no-test-framework' to prevent Rails from adding unnecessary testing components to the project:
rails g controller cookouts --no-test-framework
The above command then creates the associated 'app/controllers/cookouts_controller.rb' within the 'app/controllers' directory of the project.
From there, you are left to figure out what is necessary for your project's needs since this is dependent if your specific 'model' for your database table needs to be 'CRUD' (Create Read Update Delete) compatible for not. Reason being is that Rails has its own methods that handle CRUD capability, and you would need to use their specific function names if you want it to work properly.
A good example of a 'Controller' in action would be the 'CookoutsController' I used for my Phase 4 project which is shown in the following snippet which I will show first, and then later explain:
class CookoutsController < ApplicationController
def create
cookout = Cookout.create!(cookout_params)
render json: cookout, status: :created
end
def update
cookout = Cookout.find_by(id: params[:id])
if cookout
cookout.update(cookout_params)
render json: cookout
else
render json: { errors: [cookout.errors.full_messages] }, status: :unprocessable_entity
end
end
def index
cookouts = Cookout.all
if session[:user_id]
render json: cookouts
else
render json: { errors: ["Not authorized"] }, status: :unauthorized
end
end
def show
cookout = Cookout.find_by(id: params[:id])
if cookout
render json: cookout
else
render json: { error: "Cookout not found" }
end
end
def destroy
cookout = Cookout.find_by(id: params[:id])
if cookout
cookout.destroy
head :no_content
end
end
private
def cookout_params
# byebug
params.permit(:name, :start_time, :end_time)
end
end
Looks intimidating, right? However, if you read through each section and try to figure out what it might be doing, then you start to learn by example of what it's actually doing. This is especially useful to new developers since most of the learning process for learning development is just recognizing patterns and later just applying them at will.
Let's take the 'create' method above.
The 'create' method literally creates a new 'Cookout' on the Rails backend with the parameters that were provided from the frontend. In my frontend application, I provided the details of an individual 'cookout' which included the 'name', 'start_time' and 'end_time' for the given cookout. Rails then acknowledges this data by making a related call to the backend SQL database, and then 'renders' or 'displays' a 'JSON' (Javascript Object Notation) response that the frontend React portion of the application can use. The process of the 'frontend' receiving a JSON response from the 'backend' is useful in a full stack web application because you want to make sure that the backend acknowledges changes to the database, and you want to ensure that the frontend web application also updates its own data in real time. In a React project like this, I did this by updating the 'state' of the associated frontend data with the 'useState' React hook function accordingly.
Back to the backend again for more CRUD based methods provided by Rails. The 'update' function finds a cookout by its particular 'id', updates the 'cookout' in the backend database, and then gives the JSON response back to the frontend. The 'index' method shown above is handy as it allows the application to display ALL of the cookouts present, which was showcased on the 'View All Cookouts' React component of my project. The 'show' method would do a similar action to the 'index' method, but is done for a specific individual cookout. The 'destroy' method shown allows Rails to find a cookout by its 'id', and then use the 'destroy' method to remove it from the database.
Even though these Controller based methods provided by Rails might seem simple at first, the bigger picture is that Rails is preventing you from having to even touch a single line of SQL to make any of the database actions happen, and is super useful in any modern full stack web application.
For future reference, feel free to check out this Rails guide which goes over how 'Controllers' fit within the 'Model View Controller' (MVC) design paradigm:
Completion Of Phase 4 Project For Flatiron School Software Development Bootcamp
My Phase 4 project can be found here which includes the 'client' and 'server' components which you will have to run in two separate terminals with its own associated commands dicated in the 'README' of the project:
This particular project allowed me to utilize React with its frontend component, and 'Ruby On Rails' for its backend component.
The idea behind this app is that a user is allowed to create a cookout that other users can utilize. A user then creates foods which they will bring to this particular cookout.
One Rails specific feature that I learned for this particular app is the ability to authenticate users which is handle by the 'Users Controller' and 'Sessions Controllers' components from the Rails backend of the project. The way authentication works is that the user can sign up for an account. They can then provide their username and password and sign in.
The frontend portion of this login process is shown within this snippet here:
function LoginForm({ onLogin }) {
const [username, setUsername] = useState("");
const [password, setPassword] = useState("");
const [errors, setErrors] = useState([]);
const [isLoading, setIsLoading] = useState(false);
function handleSubmit(e) {
e.preventDefault();
setIsLoading(true);
fetch("/login", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({ username, password }),
}).then((r) => {
setIsLoading(false);
if (r.ok) {
r.json().then((user) => onLogin(user));
} else {
r.json().then((err) => setErrors(err.errors));
}
});
}
return (
<form onSubmit={handleSubmit}>
<FormField>
<Label htmlFor="username">Username</Label>
<Input
type="text"
id="username"
autoComplete="off"
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
</FormField>
<FormField>
<Label htmlFor="password">Password</Label>
<Input
type="password"
id="password"
autoComplete="current-password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
</FormField>
<FormField>
<Button variant="fill" color="primary" type="submit">
{isLoading ? "Loading..." : "Login"}
</Button>
</FormField>
<FormField>
{errors.map((err) => (
<Error key={err}>{err}</Error>
))}
</FormField>
</form>
);
}
The 'Ruby On Rails' components mentioned before help do a lot of the work to make sure that this happens smoothly. The 'UsersController' defines a 'create' method which creates a user based upon their username, and password. This is shown in this snippet found within the 'app/controllers/users_controller.rb' file of the project:
class UsersController < ApplicationController
skip_before_action :authorize, only: :create
def create
user = User.create!(user_params)
session[:user_id] = user.id
render json: user, status: :created
end
def show
render json: @current_user
end
private
def user_params
params.permit(:username, :password, :password_confirmation)
end
end
The 'SessionsController' does the heavy lifting of authenticating the same user we created earlier as it creates a 'session' only if it is able to find a user with that particular username, and if they authenticated successfully. Otherwise, it would not allow the user to proceed further. This is shown within this snippet of the 'app/controllers/sessions_controller.rb' file of the project:
class SessionsController < ApplicationController
skip_before_action :authorize, only: :create
def create
user = User.find_by(username: params[:username])
if user&.authenticate(params[:password])
session[:user_id] = user.id
render json: user
else
render json: { errors: ["Invalid username or password"] }, status: :unauthorized
end
end
def destroy
session.delete :user_id
head :no_content
end
end
Other than this portion of the application workflow, it is pretty much a React application that guides the user to create a cookout. The 'Cookout' component on the React frontend allows a user to fill out a related form to create a new Cookout. They can also edit or delete this cookout as well. A user can also create a new food that is associated with a particular cookout, and also edit or delete them as well. They are then greeted with a page that shows all of the cookouts that were made.
The better portion of the discussion of how Rails is useful in this scenario would be to discuss the workflow of how routing is done in this scenario. Whenever the user makes a React frontend call to the Rails backend, the related request is routed via the the routes present within 'config/routes.rb' file. The powerful tool that comes with Rails in this scenario would be the ability to create a 'resource' that automatically points to specific controllers and their associated methods.
In this scenario, I had to use nested routing, which is a bit complicated, but was required to fulfill the requirements for this project as it was highly dependent on the relationships present between the associated models. I mention this because the related routes file in question does feature this, so I figured I would at least mention this here before displaying the 'config/routes.rb' snippet:
Rails.application.routes.draw do
resources :cookouts do
resources :foods
end
# Login related routes:
post "/signup", to: "users#create"
get "/me", to: "users#show"
post "/login", to: "sessions#create"
delete "/logout", to: "sessions#destroy"
get "*path", to: "fallback#index", constraints: ->(req) { !req.xhr? && req.format.html? }
end
The 'CookoutsController' and 'FoodsController' act in similar ways since the workflow of making Rails method is just applying the 'convention' that matches the proper naming scheme dictated by the Rails framework itself. The idea is that as long as the proper names are kept, Rails will help you a ton with dealing with 'CRUD' capabilities on the backend. To boil it down in simpler terms, a lot of the work done to make Rails specific methods involves a workflow of finding a given Active Record object by its id, possibly manipulating it with the function, and then choosing whether or not to render it on screen for the user within its associated JSON response.
Here is the 'CookoutsController' related snippet within the 'app/controllers/cookouts_controller.rb' file for comparison:
class CookoutsController < ApplicationController
def create
cookout = Cookout.create!(cookout_params)
render json: cookout, status: :created
end
def update
cookout = Cookout.find_by(id: params[:id])
if cookout
cookout.update(cookout_params)
render json: cookout
else
render json: { errors: [cookout.errors.full_messages] }, status: :unprocessable_entity
end
end
def index
cookouts = Cookout.all
if session[:user_id]
render json: cookouts
else
render json: { errors: ["Not authorized"] }, status: :unauthorized
end
end
def show
cookout = Cookout.find_by(id: params[:id])
if cookout
render json: cookout
else
render json: { error: "Cookout not found" }
end
end
def destroy
cookout = Cookout.find_by(id: params[:id])
if cookout
cookout.destroy
head :no_content
end
end
private
def cookout_params
# byebug
params.permit(:name, :start_time, :end_time)
end
end
Here is the 'FoodsController' related snippet within the 'app/controllers/foods_controller.rb' file for comparison:
class FoodsController < ApplicationController
def create
food = @current_user.foods.create(food_params)
render json: food, status: :created
end
def update
food = @current_user.foods.find_by(id: params[:id])
if food.user_id == @current_user.id
food.update(food_params)
render json: food
else
render json: { errors: [food.errors.full_messages] }, status: :unprocessable_entity
end
end
def index
foods = @current_user.foods.all
if session[:user_id]
render json: foods
else
render json: { errors: ["Not authorized"] }, status: :unauthorized
end
end
def show
food = @current_user.foods.find_by(id: params[:id])
if food
render json: food
else
render json: { error: "Food not found" }
end
end
def destroy
food = @current_user.foods.find_by(id: params[:id])
if food.user_id == @current_user.id
food.destroy
head :no_content
end
end
private
def food_params
params.permit(:name, :cookout_id)
end
end
The authentication process for Rails involves the use of the '.authenticate' method which checks to see if the 'bcrypt' encrypted password provided to the backend is correct, and returns 'self' if this is correct. If it is not, then it returns false which then prevents the user from being able to sign into the application.
This is better explained in this reference pages:
- https://www.apidock.com/rails/ActiveModel/SecurePassword/InstanceMethodsOnActivation/authenticate
- https://api.rubyonrails.org/classes/ActionController/HttpAuthentication/Basic.html
- https://en.wikipedia.org/wiki/Bcrypt
This is also better represented in my code within the 'Sessions' controller of my project as it helps create a session for a given user only when they are authorized to do so:
class SessionsController < ApplicationController
skip_before_action :authorize, only: :create
def create
user = User.find_by(username: params[:username])
if user&.authenticate(params[:password])
session[:user_id] = user.id
render json: user
else
render json: { errors: ["Invalid username or password"] }, status: :unauthorized
end
end
def destroy
session.delete :user_id
head :no_content
end
end
After that, the overarching 'application' controller is then responsible for assigning the current user to the '@current_user' variable if a valid authenticated session is found. The '@current_user' variable is used throughout my project's Rails backend as it is useful to just use '@current_user' for various backend methods instead of having to repeat the process of finding the specific logged in user each time. This is shown here within the 'ApplicationController':
class ApplicationController < ActionController::API
include ActionController::Cookies
rescue_from ActiveRecord::RecordInvalid, with: :render_unprocessable_entity_response
before_action :authorize
private
def authorize
# NOTE: This is exactly how the /cookouts route knows what user has logged in since when the user logs in,
# This information is passed in via the params
@current_user = User.find_by(id: session[:user_id])
render json: { errors: ["Not authorized"] }, status: :unauthorized unless @current_user
end
def render_unprocessable_entity_response(exception)
render json: { errors: exception.record.errors.full_messages }, status: :unprocessable_entity
end
end
Though using Rails is slightly more complicated than what was presented earlier, it does a lot of the heavy lifting for you, and is very powerful. Honestly, I would come back to it later on to make an API as I believe this it's strong point, so I'm looking forward to potentially using it again for another side project in the future.
Completion Of Phase 3 For Flatiron School Software Development Bootcamp
I have recently completed the Phase 3 of the Flatiron School Software Development Bootcamp. As a result, I now know how to interact with the backend of a full stack web application.
Just for reminder purposes, the 'frontend' of a full stack web application is the beautiful client facing website that allows the user to interact with a given project to generate data. The 'backend' allows the user to then send the data from the 'frontend' to the 'backend', and process that data. The 'backend' of a full stack web application usually consists of some sort of database. In this case, I have learned how to utilize a SQLite3 database via a few different workflows.
At first, we learned how to deal with SQL commands directly made to a SQLite3 database. I then transitioned to learning how to use the 'Active Record' modules for the Ruby programming language. These methods do a lot of the heavy lifting of having to run SQL commands for you. The fantastic docs pages for the 'Active Record' module can be found here:
In this Ruby relatd blog post, I will discuss the topic of 'attribute accessors', which aid a Ruby developer by providing macros to allow for easier ways to generate methods for 'read' and 'write' accessor to instance variables.
For example, you can create a Ruby class called 'Food' in which you define an instance variable called 'name' that you assign a value to it when a user decides to create an instance of the 'Food' class itself:
class Food
def name=(value)
@name = value
end
end
# User actually creating an instance of the 'Food' class:
bread = Food.new
bread.name = "Salami"
What's neat about using macros in accessor macros in this scenario is that instead of having to manually define an instance variable upon creation of an instance of the 'Food' class, you can instead tap into Ruby's ability to utilize the 'attr_accessor' method that would automatically create a 'read' and 'write' method for the 'Food' class itself.
For comparison purposes, you could take the long way around by using both the 'attr_reader' and 'attr_writer' macros to allow you to create 'read' and 'write' methods automatically for the 'Food' class which span two extra lines of code:
class Food
attr_reader :name
attr_writer :name
end
# Person creating an instance of the 'Food' class:
salami = Food.new
salami.name = "Salami"
# Prints 'Salami' to console:
puts salami.name
Or, you can simply use 'attr_accessor' which allows you to create BOTH 'read' and 'write' macros for the 'Food' class within a single line:
class Food
attr_accessor :name
end
# Person creating an instance of the 'Food' class:
salami = Food.new
salami.name = "Salami"
# Prints 'Salami' to console:
puts salami.name
The end result is that by creating Ruby classes with attribute accessors gives you the flexibility in creating Ruby classes that have the ability to have read and write options for given instance variables, and also saves the user the hassle of having to create similar methods manually by hand.
The aspect of using 'attribute accessors' is better described in this article here for reference:
A great reference on Stack Overflow for this topic can also be found here:
Another great reference for Ruby classes can be found here:
Thanks for reading!
~ Sam
Completion Of Phase 3 Project For Flatiron School Software Development Bootcamp
I can easily say this web application has been quite a challenge to pull off. Not only is it using multiple React controlled forms, but it is using 'useState' and 'useEffect' hooks to make sure everything is in sync within certain parent and child components. Ontop of that, I have had to really make sure that the Ruby based models of the backend matched the requirements of the project as well, which was tricky without the ability of having a 'User' specific model to join a few of the models present.
You can start this app by navigating to the following repos, the first of which for the 'Frontend' component, and the second of which for the 'Backend' / Ruby / Sinatra backend:
- Frontend:
- https://github.com/SamuelBanya/SmoothMoves-Frontend
- Backend:
- https://github.com/SamuelBanya/SmoothMoves
I am assuming you know how to install npm and Ruby style projects, so I will leave you to install each of these components accordingly from the repos themselves. You can start the frontend component with this command:
npm start
You can start the backend component with this command:
bundle exec rake server
That being noted, this app is basically a checklist app that allows the user to specify a starting move location, and an ending move location. This was the app I wish I had before I started a huge move from the South to the Midwest a month or so ago.
The user is presented with three available routes which include the '/about', '/moves', and '/items' based components.
Within The '<About />' Component: Inside the '<About />' component, the user is presented with features that currently exist, as well as planned roadmap items via future edits.
Within The '<Move />' Component: The user is then able to progress to the '<Move />' component which allows them to create a new 'Move' which then gets sent to the backend via a POST based fetch() request.
The user is also able to edit an existing move so that they can change the 'pickup location' or the 'dropoff location' accordingly. Note that the moves are shown by their final 'dropoff location', since when you discuss a move from NYC to San Francisco, you would most likely call it your 'Move to San Francisco', hence it would be labeled as 'San Francisco' in the dropdown menu itself.
Users are able to also delete a move as well in this same menu.
By providing the user with this ability to 'create', 'read', 'update', and 'delete' moves, this makes this project's '/moves' route 'CRUD' or 'Create Read Update Delete' compatible, which fulfills the requirements of this project.
Within The '<Item />' Component: Users are able to select a dropoff location, and then select the amount of items they want to move.
This will create a corresponding carousel form of many forms, each of which contains an individual React controlled form.
The user then can submit the items to the checklist, which will then generate the corresponding 'Checkbox' Material UI components at the very bottom of the app itself.
Overall Workflow: The overall workflow of the entire app includes the following, which is included since it is a bit complicated underneath the hood, and is more for my own benefit if I want to revisit it later on to add the additional 'roadmap items' at another point:
Workflow: App.js: This is the parent component for all components in the app itself, which utilizes a 'useEffect' hook itself to match a 'GET' based fetch() request to the backend to grab all of the available moves which are used for dropdown items in various components include including the '<Moves />', and '<Items />' parent components respectfully.
This is the specific useEffect block responsible for this behavior:
const [moves, setMoves] = useState([]);
useEffect(() => {
fetch("http://localhost:9292/moves", {
method: "GET",
headers: {
"Content-Type": "application/json",
"Accept": "application/json",
},
})
.then((response) => response.json())
.then((data) => {
setMoves(data);
})
}, [moves]);
Under 'src/components/generalComponents': About.js: This just contains information about the app itself. NavBar.js: This contains the top navigation bar components of the app itself.
Under 'src/components/moveComponents': Moves.js: This is a parent component that is responsible to rendering the '<CreateMoveForm>' and '<EditMoveForm>' child components.
CreateMoveForm.js: This is a React controlled form that contains event handlers to help create moves with corresponding calls to the backend.
EditMoveForm.js: This is another React controlled form that allows the user to select a given move based upon the 'dropoff location' and to edit the individual 'pickup' and 'dropoff' locations respectively. It also allows the user to delete the given move. All of these actions help make the 'Moves' component CRUD compatible.
Under 'src/components/itemComponents': Items.js: This is a parent component that is responsible for rendering the '<ChooseMoveForm>' and '<ItemsForm>' child components. ChooseMoveForm.js: This is a component that contains a React controlled form that allows the user to select the individual move that they want to create items for. ItemsForm.js: This is a somewhat complicated section of the app itself that allows the user to determine the amount of items they want to move, as well as display a carousel based React controlled form that allows the user to enter details for each item they want to move. When the user is done filling out the information, they are then presented with a checklist at the very bottom of the page. ItemCard.js: This is the individual carousel React controlled form for each item that the user wants to ship.
Regarding The Backend: The relationships within the backend include the following ideas:
The 'Item' class has the 'belongs_to' Active Record based relationship to the 'Move' class, which is represented by the following code within the '/app/models/item.rb' file:
class Item < ActiveRecord::Base
belongs_to :move
end
The 'Move' class has the 'has_many' Active Record based relationship to the 'Item' class, which is represented by the following code within the '/app/models/move.rb' file:
class Move < ActiveRecord::Base
has_many :items
end
I then defined routes within 'application_controller' so that I can utilize various API endpoints to make calls from the frontend facing React web app to the backend facing Ruby / Sinatra instance which is utilizing a SQLite3 database, which can be observed in the following code found within the '/app/controllers/application_controller.rb' file:
class ApplicationController < Sinatra::Base
set :default_content_type, "application/json"
# Add your routes here
get "/moves" do
moves = Move.all()
moves.to_json(includes: :item)
end
post "/moves" do
move = Move.create(
pickup_location: params[:pickup_location],
dropoff_location: params[:dropoff_location]
)
move.to_json(includes: :item)
end
patch "/moves/:id" do
move = Move.find(params[:id])
move.update(
pickup_location: params[:pickup_location],
dropoff_location: params[:dropoff_location]
)
move.to_json(includes: :item)
end
delete "/moves/:id" do
move = Move.find(params[:id])
move.destroy()
move.to_json(includes: :item)
end
# NOTE: This is just to test if we are actually receiving any 'items' that are sent via POST requests from the frontend:
get "/moves/:id/items" do
move = Move.find(params[:id])
move.to_json(include: :items)
end
# NOTE: This is the original route before I looped through individual POST requests:
post "/moves/:id/items" do
# Related Docs page on 'collection.create' method:
# https://guides.rubyonrails.org/association_basics.html#methods-added-by-has-many-collection-create-attributes
item = Item.create(
move_id: params[:id],
name: params[:name],
length: params[:length],
width: params[:width],
height: params[:height],
weight: params[:weight]
)
item.to_json()
end
# NOTE: This is the modified route where you can provide the entire array:
# post "/test/:id" do
# post "/moves/:id/items" do
# move = Move.find(params[:id])
# newItems = move.items.create(params[:items])
# move.to_json(include: :items)
# end
end
The only other interesting thing to note is the cool aspects of the backend that are responsible for running the server on port 9292, which was already part of the forked project itself, and can be found within the 'Rakefile' of the project that is referred to when the user starts the backend with the 'bundle exec rake server' command:
require_relative "./config/environment"
require "sinatra/activerecord/rake"
desc "Start the server"
task :server do
if ActiveRecord::Base.connection.migration_context.needs_migration?
puts "Migrations are pending. Make sure to run `rake db:migrate` first."
return
end
# rackup -p PORT will run on the port specified (9292 by default)
ENV["PORT"] ||= "9292"
rackup = "rackup -p #{ENV['PORT']}"
# rerun allows auto-reloading of server when files are updated
# -b runs in the background (include it or binding.pry won't work)
exec "bundle exec rerun -b '#{rackup}'"
end
desc "Start the console"
task :console do
ActiveRecord::Base.logger = Logger.new(STDOUT)
Pry.start
end
As you can see from the above sections, this app is relatively complex on the backend, but is pretty simple in terms of the relationships and routes present on the backend. Overall, this was an interesting challenge to complete, and I'm very happy to say that at least I did something original with the requirements that I was given, and am happy with the results.
How To Deploy React Projects Using Netlify For The Frontend And Heroku For The Backend
In this blog post, I am going to go over how I deployed the Phase 2 project I created for Flatiron School Software Development Bootcamp, which is a React app that provides an image reference board for users. This app itself depends upon a 'db.json' database that needs a live 'json-server' instance running so that it can send related fetch() requests to it accordingly.
In terms of the technologies used for this deployment, I utilized Netlify (https://www.netlify.com/) for the 'frontend' portion, and Heroku (https://www.heroku.com/) for the 'backend' portion.
Just to give a bigger picture of what to expect, this overall method of deployment involves two separate repos for your given project:
- One repo that only contains the ‘db.json’ for the ‘backend’ database for the project which gets deployed to Heroku
- Your project's main repo that contains your project’s frontend which gets deployed to 'Netlify'
I first referenced this guide which shows you how to run 'json-server' via a Heroku backend:
I then forked the 'json-server-heroku' repo above by using the green 'Use this template' button, and named the forked repo, 'BackToTheDrawingBoardJsonServer’:
I git cloned the 'BackToTheDrawingBoardJsonServer' repo to my local machine and modified the ‘db.json’ accordingly to fit my project’s needs so that it was just a blank slate, NOTE: If you need your 'db.json' filled in with data ahead of time, you can also choose to fill it with data as well so this decision is up to you:
{
"photos": [
]
}
I then pushed my changes back to that repo mentioned above.
I then proceeded to navigate to the same directory’s project in a new terminal:
samuelbanya@Samuels-MBP ~/hub/Development/code/phase-2/BackToTheDrawingBoardJsonServer $ pwd
/Users/samuelbanya/hub/Development/code/phase-2/BackToTheDrawingBoardJsonServer
samuelbanya@Samuels-MBP ~/hub/Development/code/phase-2/BackToTheDrawingBoardJsonServer $ ls
README.md db.json package.json server.js yarn.lock
NOTE:
- If you haven't done this already, you will need to install 'Heroku CLI' using their guide found here, so do this step next if you need to:
- https://devcenter.heroku.com/articles/heroku-cli
I then 'logged in' to the 'Heroku CLI' by using the 'heroku login' terminal command which then opened up a separate web browser window to handle the authentication:
samuelbanya@Samuels-MBP ~/hub/Development/code/phase-2/BackToTheDrawingBoardJsonServer $ heroku login
After authenticating successfully, I then used the ‘heroku create’ command to create a new heroku app from the same project’s folder, and made sure to pass in the name of the project, 'drawingboardjsonserver', for the second parameter as well:
samuelbanya@Samuels-MBP ~/hub/Development/code/phase-2/BackToTheDrawingBoardJsonServer $ heroku create drawingboardjsonserver
NOTE: If you didn’t pass in the name parameter by mistake, you can reference a related guide from Heroku (https://devcenter.heroku.com/articles/renaming-apps) so that you can utilize the following Heroku CLI command sure to rename the Heroku app accordingly, which would update the app’s name on the Heroku Dashboard accordingly:
samuelbanya@Samuels-MBP ~/hub/Development/code/phase-2/BackToTheDrawingBoardJsonServer $ heroku apps:rename BackToTheDrawingBoardJsonServer
I then used the following git command to push the same Heroku app to the 'heroku master' branch:
samuelbanya@Samuels-MBP ~/hub/Development/code/phase-2/BackToTheDrawingBoardJsonServer $ git push heroku master
I then used the ‘heroku open’ command to open up the Heroku app in a new web browser window to check that it was working successfully:
samuelbanya@Samuels-MBP ~/hub/Development/code/phase-2/BackToTheDrawingBoardJsonServer $ heroku open
The previous command opened the running ‘json-server’ Heroku instance in a new browser tab:
I then went back to my original project's repo, aka the second repo in the context of this deployment, and modified each of the fetch() requests to point to the Heroku app’s actual API endpoint instead:
Example from ‘Board.js’ that was modified accordingly:
useEffect(() => {
fetch("https://drawingboardjsonserver.herokuapp.com/photos", {
method: "GET",
headers: {
"Content-Type": "application/json",
},
})
.then((response) => response.json())
.then((data) => {
setPhotos(data);
});
}, [photos]);
Modified ‘Upload.js’:
fetch("https://drawingboardjsonserver.herokuapp.com/photos", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
dataArray: dataArray,
}),
})
.then((response) => response.json())
.then((response) => {
console.log("response (from fetch request): ", response);
});
} else {
setErrors(["Name of photo is required!"]);
}
Modified ‘Photo.js’ fetch() request:
fetch(`https://drawingboardjsonserver.herokuapp.com/photos/${id}`, {
method: "DELETE",
})
.then((response) => response.json())
.then(() => {
console.log("Delete request success!");
onDeletePhoto(id);
});
I then logged into my Netlify account, and connected my GitHub account.
I then added the second repo to it, and deployed it via the Netlify web GUI:
This resulted in the live website being deployed here on Netlify:
Here's a summary of the involved components:
- End result of my Phase 2 Project:
- Live site on Netlify, which I basically just pointed the main GitHub repo to:
- https://backtothedrawingboard.netlify.app/board
Related repos:
- Frontend, deployed to ‘Netlify’:
- https://github.com/SamuelBanya/BackToTheDrawingBoard
- Backend for ‘json-server’, deployed to ‘Heroku’:
- https://github.com/SamuelBanya/BackToTheDrawingBoardJsonServer
Heroku app portion of ‘json-server’:
Hopefully you found this useful, since I could not find a single guide that even did remotely half of these steps in a nice and easy fashion.
Thankfully, I didn't find this process to be too hard, and was actually able to repeat this process for the rest of the projects so that I now have a nice looking portfolio site that has all my projects deployed to Netlify accordingly:
Thanks for reading, and have fun deploying your own projects with 'Netlify' and 'Heroku'!
~ Sam
Completion Of Phase 2 For Flatiron School Software Development Bootcamp
I am happy to note that I have successfully completed the requirements of Phase 2 for the Flatiron School Software Development Bootcamp.
Overall, it was not that easy to complete, but I am very proud that I did it. React itself was a very new topic for me, so unlike the previous phase where I completely knew what I was doing, I felt like I was thrown into the deep end with a floatable raft that comprises the React docs themselves.
React to me is a very neat framework that clearly has its reasons for its design choices. It aims to be a framework where you can really build an all-in-one page app that flows so nicely with parent and child components being able to pass down 'props' which contain related attributes or callback functions that can be used seamlessly throughout a project.
One particular topic I would like to focus on within this post is the 'useEffect' hook and why I find it to be one of the selling points for React as it shows just how powerful React is in terms of being able to update a page in real-time.
The 'useEffect' React hook allows you to call a load event when a page fully renders. This means that you can totally take advantage of using an API call to a local 'db.json' with a fetch() call when a page loads. This is awesome because you can show components such as images being updated in real time.
A good example of this would be from my own Phase 2 project mentioned in my earlier post. In this project, I had to grab the 'photos' object from the related '/photos' endpoint. In order to do this, I had to utilize 'useEffect' and call the endpoint accordingly, and make sure to pass in the 'photos' variable I created with the 'useState' hook so that I can ensure I was including the most up-to-date array, as shown by this snippet from my 'Board.js' component:
useEffect(() => {
fetch("http://localhost:3000/photos", {
method: "GET",
headers: {
"Content-Type": "application/json",
},
})
.then((response) => response.json())
.then((data) => {
setPhotos(data);
});
}, [photos]);
I even ended up using the 'useEffect' hook in the overarching parent component, 'App.js', to set the default theme of the project to be the light theme, 'App light', as shown with this 'useEffect' example from the 'App.js' component snippet here. You'll notice that in this example however, I am passing an empty dependency array as the second argument to 'useEffect' so that the function call to the 'setTheme' function is only called once:
useEffect(() => {
setTheme("App light");
}, []);
Overall, the 'useEffect' hook as seen by the two examples above has its importance for allowing a project to be dynamic to be able to continuously call a function if needed, or simply call it once.
The 'useEffect' hook itself's Docs page can be found here for reference, NOTE: Stick to the 'Function' components sections if you're not used to creating 'Class' components in React:
Thanks for reading my post!
~ Sam
Completion Of Phase 2 Project For Flatiron School Software Development Bootcamp
I am pretty stoked to have finished my Phase 2 project for the Flatiron School Software Development bootcamp called 'Back To The Drawing Board', which can be found here:
This project runs locally, so you will have to use the 'git clone' command to pull that project down to your local machine, and install necessary dependencies with 'npm install' in the root directory.
Also, this utilizes the 'json-server' dependency, so you would have to install it with 'npm install json-server', and then run the project accordingly with the following commands:
json-server --watch db.json
npm start
You can watch my YouTube demonstration video of this project here:
OVerall, this project was a bit more enjoyable to work on than the Phase 1 project since I had more of a cohesive and easier idea to implement instead of my previous project which started out as a clone of 'Rover.com'. This time around I decided to simply make an image reference board app in which you can upload art images with a name and hyperlink to a canvas. Once on the page, the user can then drag the images around, and resize them to suit the art you're working on.
I was able to get the last two functions through the 'Draggable' and 'Re-resizable' libraries found here respectively:
After a bit of research, I actually found the perfect live code sandbox demo that was similar to the functionality I wanted in my app, which is the following:
In order to get a similar functionality in my project, I had to build out my app with an app hierarchy that allowed me to be able to utilize 'props' to pass down attributes and callback functions to be able to control what's being shown on screen.
The relationships of the components present for the project include the following ideas:
- The 'App.js' parent component is responsible for the routing as well as the 'useState' section that controls the overall theme of the app
- The 'About.js' child component details the functionality of the app itself
- The 'Board.js' child component does a lot of the heavy lifting for the image board itself as it uses the 'useEffect' hook to grab images that were stored onto the 'db.json' database with a 'GET' request via 'json-server', and also handles deleting images via a callback function that is later passed to the 'Photo' component
- The 'Photo.js' component contains the individual photo card elements that contain the '<Draggable>' and '<Resizable>' components respectively which can be dragged across the screen and resized at will
- The 'Theme.js' component uses the 'onChange' event to grab the '<select>' tag's value and pass it up to the 'App.js' component to ultimately change the theme with an associated callback function
- The 'Upload.js' component uses a fetch() request to store the 'photoName' and 'photoLink' of the image that the user provided which is then displayed later onto the 'Board.js' component
As you can imagine, dealing with these components at times can be a bit rough mentally, but once you get the hang of being able to pass 'props' back and forth between parent and child components, it becomes more of a balancing act to make sure everything is working correctly.
Another major roadblock I faced was actually how to implement 'V6' style routing into the project as newer projects created with the 'create-react-app' template from React demand that you utilize the 'V6' style of routing. With a bit of research, I found out this particular page on 'react router' to help a ton for this issue:
The fix basically is to install the necessary 'v6' component accordingly and to adjust the routing given the ideas from the blog post above to utilize the '<Routes>' and '<Route>' component to conform to the 'V6' style:
npm install react-router-dom@6
The main part that threw me off for a bit was how to actually deal with individual 'photo' components I wanted to create. I figured out that this required the use of a '.map()' iteration so that I could iterate through the entire 'photos' object returned from 'db.json'. I placed this into the contents of a related variable, which is shown in the related snippet from the 'return' statement for the 'Board.js' component below. Even indexing into the specific sections of the 'photo' object to create the 'photoName' and 'photoLink' variables respectively was a slight challenge, but a bit fun since I got to work with slightly complicated data structures as a result:
const photoToDisplay = photos.map((photo) => {
return (
<div>
<Photo
key={photo["id"]}
id={photo["id"]}
photoName={photo["dataArray"][0]["photoName"]}
photoLink={photo["dataArray"][0]["photoLink"]}
onDeletePhoto={handleDeletePhoto}
/>
</div>
);
});
return <div>{photoToDisplay}</div>;
Inside the 'Photo.js' component, I had to then utilize a set of nested components so that both 'Draggable' and 'Resizable' components could be used, which is shown in this return statement snippet from the 'Photo.js' component:
return (
<Draggable>
<Resizable
id={id}
className="imgClass"
defaultSize={{
width: 100,
height: 100,
}}
style={{
background: `url(${photoLink})`,
backgroundSize: "contain",
backgroundRepeat: "no-repeat",
}}
lockAspectRatio={true}
>
<button onClick={handleDeletePhoto}>❌</button>
</Resizable>
</Draggable>
);
I was able to handle obtaining the photos themselves with a related fetch() call in the 'Board.js' component which calls 'db.json' via 'json-server' via the React based 'useEffect' hook. On this same note, the one thing that almost tripped me up was the use of the second parameter for the 'useEffect' call to literally utilize the same 'useState' variable called 'photos' so that I can store the contents of the filtered array into it. This was so that I could display the images in real time, even if I deleted one from the 'Board.js' component itself via the related callback function, which is shown in this snippet:
function Board() {
const [photos, setPhotos] = useState([]);
function handleDeletePhoto(id) {
const updatedPhotosArray = photos.filter((photo) => photo.id !== id);
setPhotos(updatedPhotosArray);
}
useEffect(() => {
fetch("http://localhost:3000/photos", {
method: "GET",
headers: {
"Content-Type": "application/json",
},
})
.then((response) => response.json())
.then((data) => {
setPhotos(data);
});
}, [photos]);
Overall, I think this is a great showcase of how much of React I've learned so far, and it really is a great simple, but effective web app written in React!
How I Created The 'morrowind-emacs-theme' For Emacs, And Pushed It To MELPA For Review
I have a thing for themes, especially in text editors. The way I see it is if you have to live inside a program all day doing something for work, like text editing for documents and todo lists or creating code for software development projects, you might as well be comfortable with the visual colors of the program you're using.
That being said, I recently created my own theme called 'morrowind-emacs-theme', which can be found here:
I utilized the excellent 'ThemeCreator' web app that basically allows you to make a new theme on the fly in your web browser, after which you can then export the same theme to a variety of text editors like IntelliJ, Textmate, Emacs, Vim, etc:
I based my Emacs theme upon the in-game menu from the game, 'The Elder Scrolls III: Morrowind', which is a game I grew up playing in my teens, and have very fond memories of. My short blurb on this game is that it is quite possibly one of the best role playing games that have ever existed in terms of the amount of freedom you could have within a single game. You literally could do anything you wanted, and however you wanted.
More so, I remember this Toonami video on TV when the game first came out which got me so excited about it in the first place:
- Toonami Reviews - Elder Scrolls III - Morrowind (https://www.youtube.com/watch?v=H-uh2CPlTx8)
You can imagine that I liked that game so much I figured it would be cool to make a color theme based upon the menu colors for that game, and the end results have been pretty good for the most part.
I also went ahead and have begun the process for my theme to get approved for 'MELPA' (https://melpa.org/#/) which is basically the largest package database for Emacs packages. It's the big leagues comparatively since if you can get your package on MELPA, you're going places, or at least will get noticed by someone using Emacs with 'M-x list packages' one way or another.
My related pull request to get approved my theme package recipe to be included on MELPA can be found here:
I'll be honest, I'm pretty excited about this, since if this works out, I'll create a ton more themes and become the themes guy for Emacs if I can help it :)
~ Sam
How I Created An 'apps.musimatic.xyz' Subdomain To House My Portfolio Projects
I thought about 'where' to host my portfolio projects, and despite there being some great alternatives like 'Netlify' and 'Heroku' that do a lot of the heavy lifting for you, I went ahead and took up the challenge to self-host my own projects subdomain.
I did some research and found this great blog post on the topic of how to host subdomains within the same website on a VPS running 'nginx':
I then proceeded to go to 'epik.com' which I use as my domain registrar, signed into my account, and modified the 'DNS Records' section accordingly for the 'musimatic.xyz' domain so that I had the following two domain records to handle 'AAAA' for 'IPv6' and 'A' for 'IPv4':
Host | Type | Points To | TTL |
apps | AAAA (IPv6) | 2604:a880:800:14::11:6000 | 30 |
apps | A (IPv4) | 104.131.2.109 | 30 |
I then created a related directory, '/var/www/apps' on the Digital Ocean VPS running the 'musimatic.xyz' website itself.
I then changed the ownership of this same directory with this command:
sudo chown -R www-data:www-data /var/www/apps/
I then created an 'index.html' file in '/var/www/apps':
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8"/>
<title>Document</title>
</head>
<body>
<h1>Welcome To 'apps.musimatic.xyz'</h1>
</body>
</html>
I then placed this into the related nginx config in '/etc/sites-available/apps':
server {
listen [::]:80;
listen 80;
# Path to default 'index.html' page for 'apps.musimatic.xyz/index.html' page:
root /var/www/apps;
# Define the index page to use:
index index.html index.html;
# Allow Nginx to use the empty name:
server_name apps.musimatic.xyz;
location / {
# Return a 404 error for instances when the server
# requests for untraceable files and directories.
try_files $uri $uri/ =404;
}
}
I then tried to test the config with this command:
root@musimatic:/var/www/apps# sudo nginx -t
nginx: the configuration file /etc/nginx/nginx.conf syntax is ok
nginx: configuration file /etc/nginx/nginx.conf test is successful
I then created this symbolic link accordingly:
sudo ln -s /etc/nginx/sites-available/apps /etc/nginx/sites-enabled/
I then restarted Nginx with this command:
sudo systemctl restart nginx
I then ran the following 'certbot' command as the 'root' user to generate a corresponding SSL certificate for the 'apps.musimatic.xyz' domain:
certbot
I then used the following prompts for the 'certbot' wizard:
- 2: apps.musimatic.xyz
- 2: Redirect - Make all requests redirect to secure HTTPS access.
I then was greeted with a message stating that this process was successful, and I am now able to see the basic page at 'apps.musimatic.xyz' without a problem:
Going forward, I plan on being able to deploy a 'React' website that hosts my projects site on that section of the site, so it should be pretty cool to see where it goes next. At least the heavy lifting of the networking side of this is complete, so that should be good going forward.
For now, I have created a corresponding GitHub repo for the 'apps' portion of my website which can be found here:
Also, if I plan on creating related '<a></a>' anchor tags on that '/var/www/apps' subdomain for 'index.html' for any specific projects, I noted the following as an example:
- Since I have 'root /var/www/apps;' present in my Nginx config, I could then use either of the following links in the future within related anchor tags so that I can refer to any specific project pages themselves
- Example future project anchor tag options to use within an anchor tag:
- https://apps.musimatic.xyz/jsprojectwebsites/project1/
- https://apps.musimatic.xyz/jsprojectwebsites/project1/index.html
- I can even limit it further to JUST be the specific subdomain due to link shortening that's possible within HTML links themselves to be a relative file path:
- 'jsprojectwebsites/project1/index.html'
One step at a time, but a definitely great (and somewhat involved) step forward has been taken today.
~ Sam
How I Modified My Emacs Config To Be Somewhat Useful For 'lsp-mode'
I have been consistently frustrated with trying to use Emacs for web development since 'lsp-mode' is really not that easy at all to set up.
The typical suggested workflow is to just use 'use-package' in your Emacs config to allow 'lsp-mode' to be installed, followed by a lot of configuration in terms of how you want 'IDE-like' qualities to be added to Emacs.
The problem that I found was that the language servers by default were automatically installed no matter what I did. The problem with this is that you really don't know what 'NodeJS' is going to install for you, nor any other terminal utility based package manager is going to pull in for you.
I went through so many iterations for the past few months to get this right, and really couldn't find any relief for this to the point where I was even debating checking out 'Spacemacs' or 'Doom Emacs' again just for sane defaults for 'lsp-mode'.
However, through a ton of research, I found these two very web development centered Emacs videos on how to configure it with 'lsp-mode', and it found a nice sweet spot in my Emacs config as a result:
- Setting up Emacs for Typescript React projects with lsp-mode and prettier (https://www.youtube.com/watch?v=ELOmzi0RW_8)
- Emacs - Updating init.el for Typescript React (https://www.youtube.com/watch?v=FIW31ivaxjg)
I also got rid of a few things in my config I just don't flat out use in my Emacs workflow as well.
Overall, getting closer to what I want. I even was able to modify fonts accordingly based upon the system in which Emacs runs as well, so that was cool to have too.
End results of my changes can be found here:
~ Sam
Helping Triage Bugs For Emacs Org Mode
I have been helping triaging a few bugs for Emacs Org Mode on the weekends, and honestly, its been kind of fun. I was able to reproduce a few personally, and am aiming to figure out how to fix them along with the help of the existing maintainer named Ihor.
He's been super helpful to a newbie like myself, and is a complete wizard when it comes to his own Emacs workflow. Seeing him be able to handle his emails all within Emacs by using 'notmuch' was pretty awesome to see, and makes me want to do the same as well one of these days if I can figure out how to make it work with Fastmail.
Anyway, here's to contributing to one of the greatest modes that Emacs ever has created (Org Mode), and to the best editor of all time (Emacs). I literally would not have been able to handle my last 3 jobs if it weren't for both of these two tools alone since Vim and Obsidian don't even come close to the power of Emacs and Org Mode.
~ Sam
How To Use 'rsync' To Sync Saves Between The Anbernic RG351MP And Anbernic RG351V
I finally figured out how to use 'rsync' so that I can sync battery saves between two handheld retro handheld devices I bought last year, which include the 'Anbernic RG351MP' and 'Anbernic RG351V':
- https://anbernic.com/products/anbernic-new-rg351mp-retro-games-built
- https://anbernic.com/products/anbernic-new-rg351v
'rsync' is basically a program that allows you to sync files between two Linux machines. You can use it for backing up files, entire harddrives, websites, you name it.
The cool application that I am going to explore in this blog post is how to make 'rsync' work for you so that you can seamlessly backup saves between your devices.
NOTE:
- This guide assumes you know how to create 'ssh' keys on different machines.
- Also, this guide assumes you are running these commands on a Linux laptop or desktop computer since its just easier with Linux to do this kind of admin tasks on Linux than other OS's.
- If you ALREADY have existing saves on your retro handheld, then I would say follow this blog post first to set everything up, and then place the SD card of the retro handheld in your Linux computer to then move over the remaining '.srm' saves to the '/storage/saves' folder you setup accordingly.
What you will need to have the following:
- A central server that is capable of running 'rsync': This could include a 'Raspberry Pi', old Dell Optiplex or laptop, etc, basically anything that could run Linux on your local LAN network.
- An Anbernic RG351V, an Anbernic RG351MP, (or both like in my case).
- Also, make sure that you're using the latest version of '351Elec' on the handheld devices ("Pineapple Forest" is what I'm using for this guide).
- Ideally, a Linux laptop or desktop computer that you can ssh into all of these devices so you don't have to just use the central server to do all this.
With this in mind, let's get started.
First, install 'rsync' on the central Linux server you plan on using.
In my case, I have an old Dell Optiplex that's running 'Fedora Server' on my local LAN network.
I simply used this command to install 'rsync', but you might need to modify this command depending on what Linux distro you're using:
sudo dnf install rsync
The next step is to pick a file path location on your Linux server where you want to dump your emulator saves to. In my case, I have a harddrive automatically mounted to '/media/REDHDD' via a cronjob that starts when my Linux server boots up. As a result, I used the '/media/REDHDD/EmulatorsFolder/saves' directory. Keep this in mind for the 'rsync' commands later in the blog post.
After that, you will then need to be able to 'ssh' into each of the handheld devices. Here is the default 'ssh' command with its associated password for the default 'ssh' user on the 'Anbernic RG351MP' and 'Anbernic RG351V' devices. If you have BOTH the 'Anbernic RG351V' and 'Anbernic RG351MP', please open up two separate terminal windows to do the following commands in tandem:
NOTE: Any time you see a '#' sign, this is just a comment within the script below telling you what to enter
Anbernic RG351MP version of the 'ssh' command:
ssh root@rg351mp
# NOTE: Once it prompts you for a password, enter in the following password as the following without the hashtag:
# 351elec
# Then, press the 'Enter' key on your keyboard
Anbernic RG351V version of the 'ssh' command:
ssh root@rg351v
# NOTE: Once it prompts you for a password, enter in the following password as the following without the hashtag:
# 351elec
# Then, press the 'Enter' key on your keyboard
Now that we are connected into the retro handheld via 'ssh', you'll want to do the following command to make a 'saves' directory on the SD card (NOTE: If you have BOTH Anbernic devices, repeat this command in each separate terminal window):
mkdir saves
Great, we now created a dedicated 'saves' folder on the SD card. On a separate note, we will later refer to this file path as '/storage/saves' since 351Elec mounts an SD card in the '/storage' mountpoint by default, and also due to the fact that we created this directory in the 'root' of the SD card. Hence, '/storage' (root of the SD card) + 'saves' (directory we just created) –> '/storage/saves'.
Now, let's change our focus to 'Retroarch' on the retro handheld devices themselves by doing the following steps:
- Turn on the given retro handheld, let '351Elec' boot successfully, and then navigate to 'Tools > Start 64bit RetroArch'.
- Once RetroArch starts up, go to 'Settings > Directory > Save Files'.
- Click on this menu option, and change it to '/storage/saves'.
- Then, go to 'Settings > Saving > Write Saves to Content Directory'.
- Click on this menu option once, and make sure it is set to 'OFF'.
- Then, go to 'Main Menu > Configuration File > Save Current Configuration', and click this menu option to save your Retroarch configuration.
Now for the testing portion of this blog post:
Pick a retro game where you know the game itself would have saved onto the cartridge in real life. Ex: A save point in some Final Fantasy RPG, or Chrono Trigger, is a perfect example of this since when you save the game, it would have saved to the actual cartridge's memory in real life. In the case of emulators like this, when you save your progress while playing a ROM in an emulator like this, RetroArch will create a corresponding '.srm' save file in the folder we chose above, aka '/storage/saves'. On a related note, the '.srm' file will have the same name of the corresponding ROM itself, just with the '.srm' file extension, so they are super easy to spot in a file manager application.
In my case, I used 'Super Mario World', and deliberately beat a previous 'Ghost House' level in a different section of the map to force the game to prompt me to 'Save and Continue'.
Now that we know we have saved our game accordingly, we want to sync our latest battery save to our central file server accordingly.
Here are the related 'rsync' commands you'll need to sync up your save to your file server, so note, PLEASE pay attention to what you're doing since I don't want you to lose progress on your games.
Here are the 'rsync' commands to sync your game save up to the central file server:
GENERAL RSYNC NOTE:
- This is what tripped me up when I first figured out the 'rsync' command in this scenario, but if you think about it, this is more of an 'rsync' file path type idea:
- The rsync command's second argument file path directory is always one directory up from what you think it should be:
- Ex using the first set of commands below to sync file from the retro handheld UP to the file server:
- Instead of using '/media/REDHDD/EmulatorsFolder/saves' as the second argument, I had to instead use '/media/REDHDD/EmulatorsFolder' instead.
- Ex using the second set of commands below from the file server DOWN to the retro handheld:
- Instead of using 'root@rg351mp:/storage/saves' as the second argument, I had to instead use 'root@rg351mp:/storage' as the second argument.
NOTE:
- Please adjust the second portion of the command, '/media/REDHDD/EmulatorsFolder' accordingly to whatever file path you want to place them onto your file server, since this is just what I personally do for my saves:
From The RG351MP To File Server:
rsync -av root@rg351mp:/storage/saves /media/REDHDD/EmulatorsFolder
From RG351V To File Server:
rsync -av root@rg351v:/storage/saves /media/REDHDD/EmulatorsFolder
Then, to sync them from the file server to the other handheld, you can then use these commands accordingly:
NOTE: Please adjust the first portion of the command, '/media/REDHDD/EmulatorsFolder' accordingly to whatever file path you want to place them onto your file server, since this is just what I personally do for my saves:
From File Server To RG351MP:
rsync -av /media/REDHDD/EmulatorsFolder/saves root@rg351mp:/storage
From File Server To RG351V:
rsync -av /media/REDHDD/EmulatorsFolder/saves root@rg351v:/storage
And that's it!
It took a while for me to figure out, but honestly, this is going to be awesome, especially if I utilize some Bash aliases accordingly in my '~/.bashrc' config accordingly.
Here's an example of how to use Bash aliases in this case to make your life easier
I could create a 'mpup' Bash alias to run the first command on the 'RG351MP' to sync UP to the file server:
alias mpup="rsync -av root@rg351mp:/storage/saves /media/REDHDD/EmulatorsFolder"
I could also create a "vup" Bash alias to run the first command on the 'RG351V' to sync UP to the file server:
alias vup="rsync -av root@rg351v:/storage/saves /media/REDHDD/EmulatorsFolder"
I could then make the reverse 'vdown' Bash alias to bring the latest saves from the file server DOWN to the 'RG351V'
alias vdown="rsync -av /media/REDHDD/EmulatorsFolder/saves root@rg351v:/storage"
I could also then make the reverse 'mpdown' Bash alias to bring the latest saves from the file server DOWN to the 'RG351MP'
alias mpdown="rsync -av /media/REDHDD/EmulatorsFolder/saves root@rg351mp:/storage"
You would then just need to place these commands into your '~/.bashrc' config, and you would be good to go!
You can find these Bash alias commands above, as well as any other related alises I've used here, so feel free to steal them to use them in your own dotfiles on your machine:
Hope this helps someone out who has Anbernic retro handheld devices like me!
~ Sam
Completion Of Phase 1 For Flatiron School Software Development Bootcamp
I am happy to have completed Phase 1 of the Flatiron School Software Development bootcamp program!
It has been a learning experience in many ways for me, as I already knew a lot of JS development through various tech support jobs I have had over the past few years.
However, there are a few topics that were introduced within this phase that were challenging even for someone as technical as myself.
One such topic that I would like to go over within this blog post is the 'arrow' function. The idea behind 'arrow' functions is that they allow you to write less code by not having to declare 'function()' sections in your code.
For example, you can write an addition function that takes in two numbers, and returns a sum that looks like the following:
function add(value1, value2) {
return value1 + value2;
}
The cool thing is that you can shorten this a bit by using the '=>' arrow function expression:
const add(value1, value2) => value1 + value;
To the untrained eye, it reads a bit differently, and takes some time getting used to. However, if you compare this to a larger function that requires a more involved built-in method like Array.prototype.map(), you can convert something like this:
const numbers = [1, 2, 3, 4, 5];
const squares = num.map(function(x) {
return x ** 2;
})
… And by using an arrow function, we can then convert the 'squares' function to a single one line function accordingly:
const numbers = [1, 2, 3, 4, 5];
const squares = nums.map(x => x ** 2);
The power of using an arrow function becomes super useful when you start getting into making API calls with the 'fetch()' method.
Using arrow functions becomes more useful when you start getting into using the 'fetch()' function to make API calls to an endpoint, especially when chaining '.then()' clauses after the initial call.
Here's an example of a 'fetch()' API call 'localhost' server running on port 1313:
fetch("http://localhost:1313")
Here's the same example, but this time, we are now processing the data into a resulting JSON object with the '.json()' method. Notice how we still have to use a bulky 'function() { }' section, and also have to include a 'return' statement:
fetch("http://localhost:1313")
.then(function(response) {
return response.json();
})
Now, let's take this same example, and make it elegant with yet another arrow function. Notice how we can totally get rid of the 'return' statement, since the 'return' statement for an arrow function is implicit if the function only calls for one line of code:
fetch("http://localhost:1313")
.then((response) => response.json();)
The cooler variation is that we can even drop the additional parentheses outside the initial 'response' since we are only dealing with a single variable. The end result is a cleaner, and easier to read function. See how nicer this looks?
fetch("http://localhost:1313")
.then(response => response.json();)
With time I was able to slowly really appreciate how awesome arrow functions were as they made writing functions for various projects that much more simpler, and manageable.
Though there are other topics I could go on about at a later time which were a bit tricky for myself, such as determining the 'Big O' for a given algorithm to determine its efficiency, and more advanced object orientated programming styles in JavaScript, I honestly can say that I feel a lot more confident than before in being able to reference MDN docs from Mozilla when it comes to most JavaScript methods. This skill itself is invaluable since there always will be a built-in function that you might not remember how to utilize completely, and the MDN docs page usually offers a pretty good example of how to actually use it in action.
Overall, I am glad to have made it this far into the program given what I have had to balance in terms of job work load, as well as personal issues during the last few months. I am slowly making it, one day at a time, and becoming the web developer I truly want to be!
By the way, here are some MDN resources for some of the JS topics mentioned above for reference:
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions/Arrow_functions
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map
- https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map
- https://www.freecodecamp.org/news/big-o-notation-simply-explained-with-illustrations-and-video-87d5a71c0174
~ Sam
Completion Of Phase 1 Project For Flatiron School Software Development Bootcamp
I am happy to note that I have successfully completed the Phase 1 project for the Flatiron School Software Development bootcamp, which can be found here:
I don't have a live version of this project up yet, as I am assessing what specific platforms to possibly re-host all my pre-existing projects on, but will update this site accordingly with a list of projects when I am able to figure that portion out. I am currently debating using services like 'Netlify', 'Heroku', etc. to do so.
You can watch my YouTube demonstration of my Phase 1 project here:
Regarding the project itself, I learned a lot in terms of overall expectations of how to plan a web app in general.
At first, I wanted it to be more of a web app derivative like 'Rover.com', but with access to an authenticated API such as the 'PetFinder' API.
I then attempted to try to figure out how to possible utilize an actual authenticated API. The workarounds were a bit too difficult for a project only completed in a week, as they would heavily involve utilizing NodeJS with 'Express' as a workaround with the 'DotEnv' package:
- https://www.digitalocean.com/community/tutorials/use-expressjs-to-deliver-html-files
- https://stackabuse.com/handling-cors-with-node-js/
- https://www.npmjs.com/package/dotenv
However, after dealing with many issues of running an API call within a Chrome browser such as 'CORS' (https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS), I opted to use the easier API endpoint, 'cat-fact':
I then shifted my project's focus to be centered around Wikipedia, and then obtained information regarding the cat breeds from this Wikipedia article:
I was able to scrape the related breed names for my project by using the following JS script that I placed within the Chrome Web Developer console:
let breedNamesList = document.querySelectorAll("th a");
breedNamesList.forEach((breedName) => {
console.log("{");
console.log('"name": "', breedName.textContent, '"');
console.log('"link": "', breedName.href, '"');
console.log("},");
})
I was then able to obtain all of the breed images by utilizing the following JS script in the Chrome Web Developer console:
let breedImages = document.querySelectorAll("td a img");
breedImages.forEach((breed) => {
console.log('"imageAlt": "', breed.alt, '"');
console.log('"imageSrc": "', breed.src, '"');
});
I then utilized the following JS script to obtain a list of all of the cat related Wikipedia articles on that given page:
let breedWikiLinks = document.querySelectorAll("th a");
breedWikiLinks.forEach((breedWikiLink) => {
console.log('"wikiArticleLink": "', breedWikiLink.href, '"');
})
Afterwards, I then was able to pull in this cat information with this specific function which makes a fetch() call to 'db.json', and then dumps it onto the DOM so that the user can view the breeds within the related list:
function displayWikiCatBreeds() {
fetch("http://localhost:3000/breeds")
.then(response => response.json())
.then(data => {
let breedSelectTag = document.querySelector("#breedSelect");
breedSelectTag.innerHTML = "";
data.forEach((catBreed) => {
let optionTag = document.createElement("option");
optionTag.value = catBreed["link"];
optionTag.textContent = catBreed["name"];
breedSelectTag.append(optionTag);
});
});
}
I then started creating three separate event listeners for three separate buttons:
- One button to be used to grab a cat breed image
- A second button to be used to place the cat breed Wikipedia article onto the page
- A third button to be used to grab a random cat fact to be placed onto the page
The first cat breed image button utilizes a fetch() request to make a 'GET' request for 'db.json' itself:
catImageButton.addEventListener("click", (e) => {
e.preventDefault();
// Clear out Wikipedia iframe if present on page:
let wikipediaIFrame = document.querySelector("#wikipediaIFrame");
clearElement(wikipediaIFrame);
// Clear out 'resultsHeader' and 'resultsParagraph' if present on page:
let resultsHeader = document.querySelector("#resultsHeader");
let resultsParagraph = document.querySelector("#resultsParagraph");
clearElement(resultsHeader);
clearElement(resultsParagraph);
let breedSelectTag = document.querySelector("#breedSelect");
let breedName = breedSelectTag.options[breedSelectTag.selectedIndex].textContent;
fetch("http://localhost:3000/breeds")
.then(response => response.json())
.then(data => {
let filteredObject = data.filter(element => {
return element.name == breedName;
});
let breedHeaderName = filteredObject[0]["name"];
let filteredImageLink = filteredObject[0]["imageSrc"];
let resultsHeader = document.querySelector("#resultsHeader");
resultsHeader.textContent = breedHeaderName;
let breakTag = document.createElement("br");
let breedImage = document.createElement("img");
breedImage.src = filteredImageLink;
resultsHeader.append(breakTag);
resultsHeader.append(breedImage);
});
});
The second Wikipedia article button simply makes another 'fetch()' call to 'db.json' to obtain the related Wikipedia article:
let catWikiButton = document.querySelector("#catWikiButton");
catWikiButton.addEventListener("click", (e) => {
e.preventDefault();
let breedSelectTag = document.querySelector("#breedSelect");
let breedLink = breedSelectTag.options[breedSelectTag.selectedIndex].value;
let breedName = breedSelectTag.options[breedSelectTag.selectedIndex].textContent;
breedLink = breedLink.toString().replace(/\s/g, '');
// Clear out 'resultsHeader' and 'resultsParagraph' if present on page:
let resultsHeader = document.querySelector("#resultsHeader");
let resultsParagraph = document.querySelector("#resultsParagraph");
clearElement(resultsHeader);
clearElement(resultsParagraph);
// Place wikipedia article contents into <iframe> within 'resultsParagraph' location
let wikipediaIFrame = document.createElement("iframe");
wikipediaIFrame.src = breedLink;
wikipediaIFrame.id = "wikipediaIFrame";
resultsParagraph.append(wikipediaIFrame);
});
The third random cat fact button was implemented by adding a 'click' event listener to a '#catFactButton' element. I then made a 'fetch()' call to the 'cat-fact' API. Afterwards, I converted the response into a JSON object, and then selected a random fact using the built-in 'Math.random()' library to pick a value from 0 to 4 so I can index into it properly. I then placed the result onto the DOM accordingly:
let catFactButton = document.querySelector("#catFactButton");
catFactButton.addEventListener("click", (e) => {
e.preventDefault();
fetch("https://cat-fact.herokuapp.com/facts")
.then(response => response.json())
.then(data => {
// Pick a random fact using Math.random() with 4 numbers for 5 index values from 0 to 4:
let choiceMax = data.length - 1;
let choiceMin = 0;
// From MDN Docs:
// https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/random
let choiceNumber = Math.floor(Math.random() * (choiceMax - choiceMin) + choiceMin);
let randomChoice = data[choiceNumber];
// Clear out Wikipedia iframe if present on page:
let wikipediaIFrame = document.querySelector("#wikipediaIFrame");
clearElement(wikipediaIFrame);
let resultsHeader = document.querySelector("#resultsHeader");
resultsHeader.textContent = "Random Cat Fact: ";
let resultsParagraph = document.querySelector("#resultsParagraph");
resultsParagraph.textContent = randomChoice["text"];
});
});
And that about wraps up the Phase 1 project itself. It was simple and effective, but to be honest, it did not start out that way. I learned a lot about how to really set expectations going forward on projects, and to have quicker functional prototypes to avoid having to struggle too much on a specific aspect of a project.
With this in mind, I am looking forward to more exciting web development challenges to tackle!
~ Sam
June 15, 2022
I have ported over my entire site to now be a 'hugo' based blog site, the repo of which can be found here:
Now I can easily post in single .org file and export to multiple Markdown files with ease, all with a nice theme to match.
This process was a long time coming since the look of the site needed to be updated accordingly to look more professional, and I believe 'hugo' is just perfect for this kind of thing.
The only thing to consider is where to re-host my existing projects, but to be honest, I can easily just port them over to GitHub Pages and run them there via a crontab job just the same, or just host them on this same box.
If anything, at least the blog is up as intended, which took a lot of work to ensure that the permissions carried over.
Tomorrow, I'll share a related post on my progress with the Flatiron School bootcamp I've been working on as well.
~ Sam
May 12, 2022
I've been busy with working on some coursework for the past few weeks.
I started a Software Engineering bootcamp with Flatiron School a few weeks ago since I decided enough is enough in terms of having to settle with technical support gigs as a career. I only ever get approached for tech support jobs nowadays, which is kind of sad comparatively since I have tried so hard over the past few years to be seen as more than just my current position.
Hoping I can convince recruiters otherwise one day soon, and finally get the 'Junior Software Engineer' or 'Fullstack Engineer' job I have been dreaming about for quite some time.
In terms of coursework, it has slowly been ramping up in difficulty, but in a good way. Honestly, its been very fun to be able to learn how to really get down to the nitty gritty and just work on projects on my own. To be fair, I am actually grateful that I've learned a good majority of the background of some of the material through previous jobs, but more so by dabbling in random topics for the past few years.
I am glad I decided to do a bootcamp because learning the same material yourself is doable, but without direction, deadlines, and actual 1-on-1 help, it is that much harder to do, let alone figure out since most documentation pages on any web dev topics are really only meant for seasoned professionals. Moreso, from what I've seen on most job listings, companies won't even look at you without the Bachelor's in Computer Science or a bootcamp anyway, so it's worth a shot.
I do have some ideas on how to add more to the 'Portfolio' section of this site given what I have learned so far. However, I need slightly more time to refine what I'm thinking to actually present on this site. Shouldn't be too hard to implement as the earlier basic web apps we've made are some basic NodeJS examples using 'fetch()' via 'POST' requests with chained '.then()' blocks to send and retrieve data.
On a related note, I actually created my first pull request (PR) recently since I've been trying to tweak my Emacs config accordingly and remembered that the 'Uncle Dave Emacs' YouTube channel had a video on the 'ihsec' utility that allows you to change Emacs configs on the fly:
- ihsec - Switching emacs configs on the fly! (https://www.youtube.com/watch?v=ns0rsKrG-Mc)
I realized after downloading the 'ihsec' utility and poking around on the related GitHub issues page that the 'Makefile' was expanding an environment variable to an incorrect directory due to the 'SHELL' environment variable not being used. With this in mind, I forked the entire repo, made the necessary revision, and then created a related pull request:
Whether or not Dave actually accepts the PR is one thing, but it was really really fun to do it since I love Emacs a ton.
I also moved my own Emacs config to its own GitHub repo so that I can use 'ihsec' and swap Emacs configs on the fly:
Now I can finally test other people's Emacs configs to finally maybe fix my LSP-Mode issues with autocompletion for various programming languages :)
~ Sam
April 31, 2022
I modernized both the 'Bandcamper' and 'Scripture Of The Day' projects by adding Bootstrap buttons (https://getbootstrap.com/docs/4.0/components/buttons/) and Bootstrap navbar items (https://getbootstrap.com/docs/4.0/examples/navbars/) via the CSS stylesheets for both projects.
I also refactored the code for both projects a bit as well, since they were both in a bit of rough shape:
Overall result looks decent so far, looking forward to creating more JS heavy projects soon.
Other than doing this, I've been trying to get a working 'Navidrome' instance (https://www.navidrome.org/) to host my FLAC music collection on my Dell Optiplex 7070SFF running Fedora Server, but am struggling a bit with the firewall config portion since its a headless server to begin with.
The process of adjusting the firewall will have to be done since I need to be able to access the admin page via port 4533. I don't have a window manager installed on that server, so it'll take a deep dive of the following two links to figure out how to do this via 'firewalld':
Most likely, I will have to do the following commands accordingly to open up port 4533 accordingly:
sudo firewall-cmd --add-port=4533/tcp
sudo firewall-cmd --runtime-to-permanent
Here's to having my own Spotify-like instance, aka Navidrome. One step closer to self-hosted LAN based server freedom :)
~ Sam
April 24, 2022
'ArtPortfolioCreator' is now complete:
Adjusted the stylesheets of both of the following pages to match:
End result:
- An art portfolio page that finally just works as intended by simply dumping all portfolio images into a single directory, and letting the page create itself via an hourly crontab job.
~ Sam
March 20, 2022
I did some changes throughout both of my live sites.
Here are the changes I did for the 'musimatic.xyz' tech portfolio site:
- I changed the styling of this page since I'm gearing this to be more and more of a tech resume / portfolio website.
- I revised the main CSS stylesheet so that the button transitions are nice to show off the effects I have done.
- I also added some ASCII art as the title, which really fits the vibe of the site.
- I revised the webring so that there is a link at the bottom that takes you to the top of the page if needed since webrings always contain a ton of links, and this helps with the discovery of new sites to explore from a new user's perspective
Here are the changes I did for the 'sambanya.com' art portfolio site:
- I modified the 'ArtGalleryCreator2' project so that the sorted() function present uses the 'reverse=True' parameter value so that the latest scanned artwork is displayed first.
- I made great strides to the 'Portfolio' section to align it with David Revoy's site's CSS stylesheet as I really like the way he did the layout for an artist site like his (though I will modify it later on to be truly my own in terms of color schemes present):
- https://www.davidrevoy.com/
A huge note to point out that such a revision for the 'Portfolio' section of the art site, 'sambanya.com' is a giant undertaking to do, so it's not complete by any means. This will require me to create a 'PortfolioSiteCreator' project, similar to the 'ArtGalleryCreator2' project because this will need to have thumbnails automatically created for each image that I dump into a 'portfolio' image folder. This is so I don't have to manually revise this site each and every time, and like a good developer, just automate the boring stuff so you can focus on the fun things.
The 'flexbox' JS library portion of the issue is easy to implement. It's just making sure that the resulting HTML template is repeatable and scalable is a different story. However, I don't think it will take much time, but its not that big of a deal yet, so I am in no rush to complete this.
I did create an Instagram account for my artwork as well under the name of 'ShortstopGFX', but haven't uploaded anything yet, as I am deciding 'the best of the best' of everything I've done so far to post on there:
It mostly will be filled with pen and ink drawings, as well as pixel art that I post to scene demo parties on 'Pouet' (https://www.pouet.net/) most likely.
For the uninitiated, I plan on following in the footsteps of 'ProwlerGFX', since I want to do his style of pixel art later on, but I still know that it will still take time to reach this level of artwork:
- https://www.antialias.se/
- https://www.youtube.com/user/ProwlerGFX
- https://www.facebook.com/prowlergfx/?business_id=10152592499697447
~ Sam
March 8, 2022
I have tweaked the designs of both sites a bit further, and clearly modeled the CSS styling of the buttons from a few examples I found online:
The first one is definitely looking up-to-par, and has vastly improved.
Obviously, I need to create more actual projects, but that comes with time.
Of course, that will only increase once I finish a related Typescript and Postman course, and begin figuring out how to really get more Full Stack experience to create more web apps.
I do have a few goals for the 'sambanya.com' page:
- Make the portfolio landing page standout with a few nice art examples with a 'Flexbox' gallery:
- https://fancyapps.com/playground/17g
- Rip the DOS style buttons from this website:
- https://www.mistys-internet.website/
The only thing I'm debating is the actual workflow of obtaining the images themselves again.
I'm tempted to make an 'ArtGalleryCreator3' where it would basically rip the images and create an associated thumbnails folder to then be used to display in a grid at the bottom of the page.
However, I think the end results so far are looking good so far.
~ Sam
February 27, 2022
I've been in some rough shape with my back health for the past two weeks.
This means I haven't been able to work on much art, or even the synth album I was working on a bit. Kind of sucks, but that's how life is sometimes. Can't say I didn't think of great ideas in the meantime and similar webpages to copy entire styles from though.
That being said, I did some major overhauling with the two websites I run.
I moved all of my art and music to my art portfolio website:
I then overhauled this site to only feature web dev type projects going forward so I can present a decent portfolio going forward:
I also put embedded music players from Bandcamp and Soundcloud on the music section of my main page:
Overall, I probably still need to play around with the button layouts a bit more, but its good for what it is, since I literally only manage the webpages with Org docs in Emacs, and export them into HTML which makes editing them a breeze.
My goal going forward is to make the 'Web Apps' page resemble something like one of the following websites:
To think, my old website for that original solo band, "The Bedside Morale", was never actually put up on the internet, but only was going to run on an Apache webserver back in 2011 or so. I have it archived somewhere on my file server. Should dig it up and host it for fun since the glossy buttons were beautiful for hte time being. On a similar note, it is absolutely crazy how easier things have become to deploy servers with since the average person could deploy even a Wordpress website in seconds without even needing to know a single line of PHP (unheard of back then).
Looking forward to deploying some real web apps with React, Django, etc. when I actually get better though. Probably will just stick with the Typescript course I was working on to completion, and then go straight for another Full Stack type course to tag along with related projects.
Here's to a better web development portfolio and a better career for the future.
~ Sam
February 11, 2022
Found five pretty sick Dungeon Synth albums today:
- https://protodome.bandcamp.com/album/4000ad
- https://zweihander.bandcamp.com/album/primeval
- https://darkagelegendry.bandcamp.com/album/barbarian-master-2
- https://bookofskelos.bandcamp.com/album/cryptic-conjurations
- https://mystictowers.bandcamp.com/album/caverns-of-crystal
Makes me want to complete the one synth album I've been working on for a bit, and really learn the Ardour DAW a bit better to use similar synth VST's.
What's nuts about that first 'Protodome' album above is that the guy made it with 'μMML' or 'Micro Music Macro Language', from his own personal GitHub project:
Found an additional cool OST album as well for a game that was apparently made in a week as well, neat:
Also found a crazy Impulse Tracker based album too:
Found another computer music based album too, alot of neat DOS and Windows 95 type sounds:
Found a cool ambient tape album as well:
Found a cool Japanese artist who makes random MIDI songs:
Found a cool synth album in which there are brief songs, purely made from synth samples and messing around with analog equipment:
Found a cool album that just features random DOS music:
~ Sam
February 10, 2022
Found this artist aka 'Flooko' who does some cool sci-fi type paintings.
He does a ton of timelapse videos on YouTube which showcases his technique to make acrylic paintings, cool stuff:
Found another artist that does Elder Scroll paintings as well which is neat, since they do it on small canvas discs:
Even found a guy who does Tolkien based Lord of the Rings styled paintings in a related outfit:
~ Sam
February 8, 2022
Found some more cool sci-fi artists to check out:
- Chris Foss's portfolio artwork:
- https://www.chrisfossart.com/category/portfolio/
- Chris Foss's space artwork:
- https://www.chrisfossart.com/category/portfolio/space/
- Chris Foss's related YouTube channel:
- https://www.youtube.com/c/TheChrisFossArt/videos?view=0&sort=da&flow=grid
- Chesley Bonestell's website:
- https://www.chesleybonestell.com/
- Rudolf Zallinger's dinosaur based artwork:
- https://duckduckgo.com/?t=ffab&q=rudolf+zallinger&iax=images&ia=images&iai=https%3A%2F%2Fi.pinimg.com%2Foriginals%2Fcd%2Fe5%2Fbc%2Fcde5bc4fc2c3570b0cc4f83cb3597248.jpg
Debating somehow checking out this video course by Syd Mead since it goes into exactly how he's able to produce space art:
Also debating getting a Wacom Intuos Medium PTH-660-N or a decent printer.
This random YouTube video got me thinking to maybe either get the Canon PIXMA TS3320 or Canon PIXMA TS5320 printer:
- The Best Printers for Crafters : Affordable crafting printers for every budget!
- https://www.youtube.com/watch?v=bbJ9qoOCQrA
Thankfully, there exists printer drivers for Linux for those printers too, so I'd be set with either one:
Honestly, some of the best comparison videos for printers weirdly enough has been from 'crafters' on YouTube since its not that easy to figure out what would actually work with heavier paper like bristol paper.
Slowly trying to figure out what works best for my art workflow.
Results with printing on grey toned paper with colored pencils and alcohol markers has been cool, but I definitely would like to do something between 70's sci-fi art meets classic illustrators like Franklin Booth.
One day at a time.
~ Sam
February 7, 2022
I am still debating the exact CSS stylesheet to use for the main art portfolio website:
I am still leaning heavily towards copying the style of these three sites somehow:
I found this site to be useful for studying posing figures since Blender makes my old Thinkpad X230 become a furnace, so this is a nice browser based alternative:
I also got lucky and found a YouTube channel that actually interviews a lot of the awesome 1970's sci-fi artists which is great since their workflow is so elusive even after heavy research.
Here are some cool highlights of videos I found:
- Artist Depiction by Steve R Dodd:
- https://www.youtube.com/watch?v=wePM-O-ayKg
- Artist Depiction by Rick Guidice:
- https://www.youtube.com/watch?v=eqgXo0KmgCw
- Closer Than We Think | Complete Syd Mead Interview | Retrofuturism:
- https://www.youtube.com/watch?v=Nvewl5Tlphc
For fun, just check out Syd Mead's art to see some cool retro futurism:
~ Sam
January 21, 2022
Found a couple of cool illustrators via this YouTube playlist named 'illustratori', which I'm assuming is Italian for 'illustrators':
Here are the highlights of what I found
- Mirko Hanak: Cool illustrator that emphasizes the watercolor WITHOUT an outline, very cool and unique:
- https://duckduckgo.com/?t=ffab&q=Mirko+Hanak&iax=images&ia=images
- Syd Mead: Cool sci-fi illustrator into retro futurism kind of art:
- https://duckduckgo.com/?q=Syd+Mead+illustrator&t=ffab&iar=images&iax=images&ia=images
- Chesley Bonestell: Cool sci-fi illustrator into sci-fi type backgrounds:
- https://duckduckgo.com/?q=Chesley+Bonestell+illustrator&t=ffab&iar=images&iax=images&ia=images
- Zdzislaw Beksinski (I like the color usage, but dang is this guy's work dreary and death based)
- https://duckduckgo.com/?t=ffab&q=Zdzislaw+Beksinski&iax=images&ia=images
- Dan McPharlin: Cool sci-fi type illustrator:
- https://duckduckgo.com/?t=ffab&q=Dan+McPharlin&iax=images&ia=images
Inspiring stuff for the most part.
In terms of Linux stuff, I tried installing Emacs on ScPup64 aka a derivative of Slacko Puppy Linux this week, and its been a mess trying to find the different dependencies present even with help on their forums.
Going to just stick with Manjaro for the time being until I can MAYBE find a Puppy or Dog Linux derivative that has Emacs 27+ by default.
~ Sam
January 17, 2022
It's funny how one simple change to a CSS stylesheet can make or break the effects that you're after.
Thankfully, I was able to ask the 'FancyBox' JS package about a CSS issue I had with my Art Gallery page via this GitHub issue:
They told me that I was applying style changes too broadly to the 'img' tag in general.
Therefore, I changed the section for images in the CSS stylesheet for the 'ArtGalleryCreator2' project to be the following (Related link: https://github.com/SamuelBanya/ArtGalleryCreator2/blob/main/artgallery.css):
#right_art_gallery img {
padding: 5px;
background: white;
border: 2px solid #BBB;
margin: 7px 14px 7px 0;
width: 160px;
}
#right_art_gallery img:hover {
border: 2px solid red;
}
By specifying the ID of the element itself, it then gets rid of the previous override.
This means that when images are viewed, it doesn't start at such a small size like the art images BEFORE you click into them.
The result is a better looking Art Gallery page upon clicking into images:
One problem solved at a time :)
~ Sam
January 16, 2022
I was able to deploy my art portfolio website yesterday after a ton of work to get it working with the 'Epik' domain registrar, and 'Vultur' VPS provider:
I am in the process of migrating all my artwork to it so that I have more space for it going forward.
Is it fully functional?
No way. Not at all.
There is still a lot of work to be done, since I'm trying to figure out how to create a CSS stylesheet that will rival the following artist websites that I think look fantastic:
- https://karlkopinski.com/
- https://wyliebeckert.com/
- http://www.brucepennington.co.uk/
- https://turnislefthome.com/
- https://davidmattingly.com/sketches/
- https://www.mathewborrett.com/
- https://www.stephenfabian.com/gallery
The goal is to have a look similar to the sites above, but have as minimal JS present on the site so it doesn't become too bloated.
I might even opt for a minimalistic look as per this website that was created entirely using Emacs Org Mode since I really like the fonts being used:
I do plan on carrying over the same 'Art Gallery' page from this site, but I have since updated the project to become more friendly for anyone who wants to deploy the same Art Gallery but for their OWN website.
This means if you modify the '.env' file in my 'ArtGalleryCreator2' project after deploying it on your 'nginx' based website's VPS, you too can run a similar Art Gallery page that is exactly like mine, aka read the 'README.md' and you'll be fine:
Have fun hacking away at my project on your own site.
NOTE, if you are oblivious to what hacking really means, and still think hacking has some sort of negative context, read this article by Richard Stallman that goes over why hacking is what everyone should be doing to their own computers to make their lives easier:
In short, every device should be hacked since you should have the freedom to do whatever you want to any device you own.
Stop treating the word 'hack' like such a bad thing.
~ Sam
January 9, 2022
I have been trying to figure out how to contribute to Emacs Org Mode and Puppy Linux.
In terms of my web development projects, I can't seem to get my ElectronJS project to actually produce sound on Linux, but modeling it after a similar example from GitHub, I was able to get it to work on the workbased Macbook:
This is the project that I heavily modeled after since the docs on ElectronJS on how to actually incorporate other libraries like sound libraries (ex: synths, etc) are very limited, and there are barely any actual working examples that do this on the ElectronJS docs page:
I think what I've realized is that some advice from a developer at a couple jobs ago was absolutely right:
- Just stick with web apps in an actual browser
This is to avoid having to support desktop apps that vary so widely on different architectures on different machines.
It's better to just have it work in a modern browser so that I can at least present the work.
Hoping to at least get it working in the next week or so, but if it doesn't, I might just host it on an actual page and move on. I don't want to spend too much time on a simple music application that doesn't work, and would rather it would 'just work'.
~ Sam
January 8, 2022
I have been doing a ton of artwork, and having a ton of fun as a result.
I skimmed a ton of art courses, and realized two things:
- Every single teacher that tries to cover anatomy basically rips off Bridgman or Hogarth
- Past the basics, most of these same teachers just showboat for the remaining portion of a given course with 'rendering'
With this in mind, I pretty much have just been drawing along with the Bridgman based anatomy book whenever I feel like learning more of the figure, and then will literally draw mannequin forms of action figures to further simplify poses with gesture drawings.
I even found an awesome free site that is dedicated to art reference poses which I will probably use in my workflow as well:
My current workflow for illustrations includes the following:
- Scan in ink drawings into GIMP at the end of the month for all the ink drawings I did
- When I'm ready to work on the image, open the .png, and then nuke the white background and bump up the levels to make every black pixel on the screen to be fully black
- Export the resulting .png from GIMP, and open it in Krita to work on as a digital illustration
- Once I'm done with the illustration, I can then resize the illustration to something of a super low resolution like 256 pixels in width, and then force the colors to be 16 bits for an indexed palette which pretty much converts it into pixel art
- Open up the resulting image in Grafx2 to complete the pixel art image
Though the last point doesn't guarantee a perfect pixel art image, it gets pretty close to what I want.
What I have found is that working on multiple pieces throughout a given week is actually kind of fun and rewarding.
It really goes to show you the amount of work it takes to really pull off a good illustration, and lets you have a window into what you want to actually work on in that given day.
There were days when I just wanted to work on background lighting for an illustration, vs. other days when I wanted to apply painterly type ideas to a given figure's form.
I have also come to realize that digital art itself requires so many different masking layers in order to really pull off some cool effects.
This particular video on how to do this in 'Krita' was VERY rewarding in this respect:
- Krita 4.4.3 tutorial - clone layers, filter masks, transform masks (https://www.youtube.com/watch?v=3VratqYiarc)
In terms of the artwork on this site, I've realized a few things:
- I will probably need to host a completely separate website for my artwork if I want to continue on the digital realm of art
- This is because the file sizes even for the .png's alone are pretty dang big
Luckily, there DOES exist some options but it will cost extra money to pull it off so here's my research what I did so far on this topic from my notes:
- https://www.epik.com has 'sambanya.art' available for $15 a year:
- There exists two options on 'Vultr' for a VPS that could run the specs of a site like this:
- https://www.vultr.com/products/cloud-compute/
- Related comparison table:
Storage | CPU | Memory | Bandwidth | Monthly Price |
55 GB SSD | 1 CPU | 2 GB | 2 TB | $10.00 |
80 GB SSD | 2 CPU | 4 GB | 3 TB | $20.00 |
We'll see how it goes :)
~ Sam
December 29, 2021
I re-uploaded my developer projects back to GitHub:
Reason being is that I wanted to distro-hop on my personal machines (laptop, Desktop, etc) like a mad man to check out some other workflows, though mostly because I think Thunar file manager's bug in XFCE desktop on Manjaro desktop that automatically mounts SD cards as 'root' is crazy hence I'm switching out to something better.
The less I have to actually depend upon existing on the file system for a given laptop or Desktop via a different Linux distro .iso used in 'Ventoy', the better.
This will save me some time since I keep having to re-deploy a laptop like my Thinkpad X230 each time I change my setup, so I'd rather just offload stuff to either GitHub, or my file server.
Really, I'm just aiming for something a bit more simple and hands off going forward.
I'm aiming to play around with Puppy Linux to make an Emacs specific Operating System, since I don't see too many Puppy Linux derivatives that give you a decent Emacs config from the get-go.
On the art side of things, I finished most of the Watts Atelier art course. Learned a ton, but man does that guy like to talk too much. Dude's got respectable skill though, and I definitely will use the course PDF handouts later as a reference.
I've been going through the 'Meds Map' from Ahmed Aldoori (https://medsmap.mykajabi.com/landing-page). Probably one of the best digital art courses I've sifted through in a while.
Other than that, I've completed a few digital art illustrations, and am working on getting my workflow between GIMP, Krita, and Grafx2 down pat.
I do plan on scanning stuff in, but it always take a ton of time just to get some images scanned in the first place.
But, I've since turned away from using sketchbooks for this reason, and literally just draw on printer paper to make the scanning process 100x easier. Plus, printer paper looks awesome with ink anyway.
My current art workflow includes the following:
- Scan in an ink drawing at 300 DPI with GIMP with the 'SANE' scanner plugin that you can install on any Linux distro
- Open the same drawing in GIMP, and remove the white layer to create a transparent .png
- Bring the same transparent .png into Krita to then lower the opacity to 80%, and to then create a pencil layer ontop
- Print the progress, re-ink the printed ink drawing, and re-scan it as a new layer with 'Multiply'
- Use a new layer on the layer below and fill in the color with a round hard brush in Krita
- Once it looks completed, save it, and then re-scale a different copy with 256 pixels at its width
- Re-index the painting to ONLY 16 colors
- The result is a cool looking pixel art piece, but which can still be brought into Grafx2 for further dithering
So far, the results have been pretty sweet. However, I'm still trying to figure out which specific demoscene parties to upload my work to since there are ton still going on these days at these sites:
I've already decided on an alias for my new work, which will be 'Shortstop' for a few reasons:
- Funnily enough, I actually don't like baseball much at all, so there's some irony already present
- However, I do consider myself to be an alright mediator, hence the name
- Kinda fits my personality already
Now to make my pixel art pieces more known :)
I don't plan on posting my pixel art on this site either because of the overhead, and plus, no one really cares to go to Joe Schmoe's personal site for that kind of thing.
It's just expected to be on those platform sites above, so I think I have a better shot on those instead.
Regarding music, I also thought a bit deeply about the instruments I currently play aka synth and guitar, and even though I've relegated them to just on the weekend to devote more time to art, I think my main goal going forward is to at least always create every time I sit down and 'practice' or 'play'.
Reason being is that I'm kind of sick of the 'maintenance' mode of playing that comes with playing instruments, ex: Playing old songs so I can remember them etc.
I'm really only interested in creating new things going forward.
Career-wise, I'm shooting for getting both my documentation skills and web development skills up to par. I plan on focusing on Typescript first as this is heavily used for most frameworks anyway, and serves as the foundation for things like NodeJS, Electron, React, etc.
Sure, you might get your job automated later in the future as a documentation based technical writer, but that track still might teach me the right skills to make commits on larger project repositories.
Furthermore, you never see anyone saying that there is perfect documentation out there, only the lack thereof.
Luckily, work has been really cool with allowing me to gain some related skills by allowing for some work based GitHub projects, and the opportunity to also edit the related documentation as well.
Might help out Puppy Linux, Emacs Org Mode, or some kind of emulators. Not sure yet, but documentation's a good place to start no matter what project it is.
There's even a cool Hungarian based Puppy Linux page that might be worth checking out to help out, who knows:
Might give me some cool translation skills for Hungarian as well since the dude who runs the site writes the entire page in Hungarian and is into pretty much the same things I am into for the most part.
Whatever it takes, I'll make it happen :)
~ Sam
December 16, 2021
I was able to complete all of the tasks I had assigned myself this entire year.
This is saying something because I'm a pretty motivated person, and am surprised I got through all of those tasks. Seriously.
Recently, I got the following tasks complete:
- Pretty much almost sold the old Odroid HC-4 I bought since it was such a hassle to figure out how to setup with Open Media Vault –> Don't buy one, just don't. They are a waste of time, and there are better SBC's out there for this kind of thing.
- Got 'Ventoy' (https://www.ventoy.net/en/index.html) to work with a spare 1TB external HDD to add a ton of cool Linux Live DVD ISOs to try out
- Installed Open Media Vault on a spare 128GB USB Flash Drive to basically turn my old Desktop into a mega, multi-HDD SAMBA machine
- Completely hacked the Nintendo Switch I had laying around with up-to-date GitHub patches, which is awesome
- Made a slew of work based projects on the work-based GitHub account, and have plans to put more of my own projects on my personal GitHub
- Going through most of the 'Watts Atelier' art course videos, which has been pretty good
- Completed a 'Haynes MK1-2K16' synth kit to build a functional monophonic synth
- Completed the guitar build kit from Fretwire.com, which still needs the neck to be secured in, and the bridge to be fastened
- Getting into figuring out how to do Demoscene art, which will be mega fun to do with 'Grafx2'
- Installed 'Manjaro' Linux on my Thinkpad X230 as well, since I previously only had it on my Desktop spare HDD
- Converted my old Raspberry Pi 3B+ into a SAMBA share with 'psx-pi' which now serves Sony PS2 games DIRECTLY to my slim Sony PS2 via ethernet, which is amazing
- Got both Anbernic 'RG351MP' and 'RG351V' handheld devices, and put '351Elec' ('https://351elec.de/') on them both to make them into emulation powerhouse machines. Very fun devices to have around, and man, the form factor as well as the aspect ratios are on point!
- Learned how to transfer saves from Virtual Console games from the 3DS to extract the '.dat' files to just rename them to .srm files for later use in Retroarch on the Anbernic handhelds I got, and the saves transferred beautifully
- Finally swapped out the 2032 CMOS clock battery in the Dell Optiplex file server I have since it never remembered the correct boot option to boot properly into 'Fedora Server' without a new one
Other than this, I plan on making more digital art illustrations going forward. I have shifted my mentality to really train on the fundamentals Mon to Wed, and just have fun with it for the rest of the week.
I might also try hosting a 'BBS' on a spare machine, specifically on a 'VM' within 'Cockpit' on 'Fedora'. Maybe that or a slew of game servers in some VPS instances.
The only other thing I could think of is to maybe get 'Batocera' working on a spare USB flash drive or something. Might convert over to Puppy Linux via a USB flash drive if I get bored of Manjaro though.
As long as Emacs works on any distro I'm on, I'm good :)
Overall, a decent end to the year.
~ Sam
November 19, 2021
I added some older projects to the 'git.musimatic.xyz' site, specifically under an 'Archive' repo:
I also open sourced my projects running on crontab jobs on this site as well:
- https://git.musimatic.xyz/ArtGalleryCreator/tree/
- https://git.musimatic.xyz/Bandcamper/tree/
- https://git.musimatic.xyz/ScriptureOfTheDay/tree/
- https://git.musimatic.xyz/RandomCSSColorGenerator/tree/
For my new job, I've been working on making 'hello world' typo repos as well as some basic GUI type program examples for the team to learn from. Reason being is that I have to deal with a variety of programming languages to assist Dev's on a daily basis, so I wanted to know how the very basics of the following languages at the bare minimum:
- Python
- Java (Maven, Gradle)
- Ruby
- DotNet (C#)
- JS (NodeJS)
- Elixir
I've shifted to primarily doing some skill-building work-based tasks in the beginning half of a given week (Mon to Wed) and then just doing my own thing towards the end of a given week Thursday onward. It has done wonders for my mental health, and would recommend anyone else to do the same if possible if they're trying to move their careers forward, but somehow balance it all. Life is kind of insane these days, so its good to keep it in check if you can, God willing.
Other than that, I hacked a PS4 the other week, and the older Nintendo Switch I had laying around. However, more so, I got an RCM Loader device which allows me to apply CFW (Custom Firmware) without needing to plug the Switch into a computer, which is really nice and convenient since it's kind of a pain to load any form of 'Tegra' using Linux. Now if only I could get 'Gold Leaf' to work properly (probably through related but obscure GitHub patches), that would be cool too.
Been primarily working on ink drawing when I can though. Might contribute to Emacs Org Mode though one of these days, as I'm looking for the PR commit experience :)
Planning on getting an Odroid soon to get a good SAMBA share drive going so I can easily access the ton of art resources I have on a 4TB drive laying around:
Related YouTube video which showcases it, and got me interested, as I was kind of getting sick of my Dell Optiplex file server setup I currently have since its a bit bulky. It even supports "Wake On LAN" with magic packets which is sick:
~ Sam
October 28, 2021
I released the second JeeveSobs album called "Breakpoints":
Planning to do a synth based third album later on. Probably will involve using 'JACK' via 'qjackctl' with multiple input configurations between synths, drum machines, and lots of samples with loops. Should be a lot of fun.
~ Sam
October 24, 2021
I was able to finally re-design the Art Gallery to incorporate the 'FancyBox' JS library, which makes it SUPER easy to view any images on the page.
Related link for the 'FancyBox' library:
I specifically modeled it after this example that they provided:
This is the end result:
Also, I was able to re-design the main site, and also scrape random palettes from 'Lospec'('https://lospec.com/') and also determine if the background and foreground palette colors were light or dark based upon a few random posts and articles. The end result is that the page reaches out to flip a coin to change its color scheme every minute, which is pretty a cool idea on my part. Here are the resources I used as reference materials:
- https://www.codespeedy.com/convert-rgb-to-hex-color-code-in-python/
- https://stackoverflow.com/questions/22603510/is-this-possible-to-detect-a-colour-is-a-light-or-dark-colour
These are the few sites I ripped off in terms of styling ideas:
I was also able to add a random artwork using portions of my 'Art Gallery Creator' project's code as well, and incorporated the idea of using a transparent background using this random post I found:
The Art Gallery page was created via my project named 'ArtGalleryCreator', which is an art gallery page that literally creates itself.
Here's the RandomCSSColorGenerator' project which is my Python 3 project which rips color schemes from the Lospec website ('https://lospec.com/'):
import os, random, requests, math
from pathlib import Path
from pathlib import PurePath
from pathlib import PosixPath
import itertools
# Taken from here:
# https://www.codespeedy.com/convert-rgb-to-hex-color-code-in-python/
def determine_light_or_dark_color(value):
value = value.lstrip('#')
lv = len(value)
rgb_color = tuple(int(value[i:i+lv//3], 16) for i in range(0, lv, lv//3))
# Taken from here:
# https://stackoverflow.com/questions/22603510/is-this-possible-to-detect-a-colour-is-a-light-or-dark-colour
[r,g,b]=rgb_color
hsp = math.sqrt(0.299 * (r * r) + 0.587 * (g * g) + 0.114 * (b * b))
if (hsp>127.5):
return 'light'
else:
return 'dark'
def grab_lospec_palette():
response = requests.get("https://lospec.com/palette-list/load?colorNumberFilterType=max&colorNumber=8&page=1&tag=&sortingType=default")
palette_length = len(response.json()['palettes'])
palette_list = []
for i in range(palette_length):
palette_list.append((response.json()['palettes'][i]['colorsArray']))
random_palette = random.choice(palette_list)
return random_palette
def create_css_sheet_with_lospec_palette(random_palette):
print('Now entering create_css_sheet_with_lospec_palette() function...')
print('Checking random_palette to make sure it has at least 4 colors...')
if len(random_palette) < 4:
print('random_palette doesn\'t have 4 colors... Skipping')
else:
print('random_palette DOES have at least 4 colors. Proceeding...')
content = str('#page_background {')
content += str('position: fixed;')
content += str('top: 0; left: 0; width: 100%; height: 100%;')
# content = str('body { background-color: #')
# content += str(random_palette[0])
# content += str('; ')
content += str('background-image: url("')
# Borrowed code from 'Art Gallery Creator' project:
art_gallery_path = '/var/www/musimatic/images/ArtGallery'
os.chdir(art_gallery_path)
picture_directories = sorted(filter(os.path.isdir, os.listdir(art_gallery_path)))
print('\npicture_directories: ' + str(picture_directories))
directory = random.choice(picture_directories)
print('\ndirectory: ' + str(directory))
picture_paths_jpg = (x.resolve() for x in Path(directory).glob("*.jpg"))
picture_paths_png = (x.resolve() for x in Path(directory).glob("*.png"))
picture_paths = itertools.chain(picture_paths_jpg, picture_paths_png)
picture_paths_strings = [str(p) for p in picture_paths]
print('\npicture_paths_strings: ' + str(picture_paths_strings))
picture_path = random.choice(picture_paths_strings)
print('\npicture_path: ' + str(picture_path))
regular_image_version = str(picture_path).replace('/var/www/musimatic/', 'https://musimatic.xyz/')
content += str(regular_image_version)
content += str('");')
content += str('background-repeat: no-repeat; background-attachment: fixed;')
content += str('background-size: 100%;')
content += str('opacity: 0.4; filter:alpha(opacity=40); z-index: -1; }')
content += str('#top_banner_div { border-top: 3px solid #')
content += str(random_palette[0])
content += str('; border-bottom: 3px solid #')
content += str(random_palette[0])
content += str('; background-color: #')
content += str(random_palette[1])
content += str(';')
# Determine if 'random_palette[1]' color is dark or light:
print('random_palette[1] hexcode: ' + str(random_palette[1]))
dark_or_light_palette_1 = determine_light_or_dark_color(random_palette[1])
print('dark_or_light_palette_1: ' + str(dark_or_light_palette_1))
if dark_or_light_palette_1 == 'dark':
content += str('color: white; text-align: center; }')
if dark_or_light_palette_1 == 'light':
content += str('color: black; text-align: center; }')
content += str('#left_menu_div { font-size: 15px; width: 134px; float: left; clear: both;')
content += str('font-family: Arial, Helvetica, sans-serif; }')
content += str('#left_menu_div a { color: white; }')
content += str('#left_menu_div a:hover { text-decoration:none;')
content += str('text-shadow:-1px 0 red,0 1px red,1px 0 red,0 -1px red,-1px -1px red,1px 1px red,-1px 1px red,1px -1px red;')
content += str('transition: 0.3s }')
content += str('.left_menu_section { border-radius: 5px; overflow: hidden; box-shadow: 4px 4px 10px -5px rgba(0,0,0,0.75);')
content += str('margin: 0 auto 15px 0; }')
content += str('.left_menu_section p { margin: 0; }')
content += str('.left_menu_top_bar { text-align:center; ')
# Determine if 'random_palette_2' is dark or light:
print('random_palette[2] hexcode: ' + str(random_palette[2]))
dark_or_light_palette_2 = determine_light_or_dark_color(random_palette[2])
print('dark_or_light_palette_2: ' + str(dark_or_light_palette_2))
if dark_or_light_palette_2 == 'dark':
content += str('color: white')
if dark_or_light_palette_2 == 'light':
content += str('color: black')
content += str('; box-shadow: 0 16px 20px rgba(255,255,255,.15) inset;')
content += str('background-color: #')
content += str(random_palette[2])
content += str('; margin-bottom: 0px; }')
content += str('.left_menu_bottom_section { padding: 4px; background-color: #')
content += str(random_palette[3])
content += str(';')
# Determine if 'random_palette[3]' color is dark or light:
print('random_palette[3] hexcode: ' + str(random_palette[3]))
dark_or_light_palette_3 = determine_light_or_dark_color(random_palette[3])
print('dark_or_light_palette_3: ' + str(dark_or_light_palette_3))
if dark_or_light_palette_3 == 'dark':
content += str('color: white; }')
if dark_or_light_palette_3 == 'light':
content += str('color: black; }')
# Place css sheet in '/var/www/musimatic/css' directory:
with open('/var/www/musimatic/css/index.css', 'w') as f:
f.write(content)
f.close()
def create_css_sheet_with_grey_purple_scheme():
print('Now entering create_css_sheet_with_grey_purple_scheme() function...')
content = str('body { background-color: grey; }')
content += str('#top_banner_div { border-top: 3px solid blue; border-bottom: 3px solid blue; background-color: purple; ')
content += str('color: white; text-align: center; }')
content += str('#left_menu_div { font-size: 15px; width: 134px; float: left; clear: both; ')
content += str('font-family: Arial, Helvetica, sans-serif; }')
content += str('#left_menu_div a { color: white; }')
content += str('#left_menu_div a:hover { text-decoration:none;')
content += str('text-shadow:-1px 0 red,0 1px red,1px 0 red,0 -1px red,-1px -1px red,1px 1px red,-1px 1px red,1px -1px red;')
content += str('transition:0.3s }')
content += str('.left_menu_section { border-radius: 5px; overflow: hidden; box-shadow: 4px 4px 10px -5px rgba(0,0,0,0.75);')
content += str('margin: 0 auto 15px 0; }')
content += str('.left_menu_section p { margin: 0; }')
content += str('.left_menu_top_bar { color: lightblue; box-shadow: 0 16px 20px rgba(255,255,255,.15) inset; text-align: center;')
content += str('margin-bottom: 0px; }')
content += str('.left_menu_bottom_section { padding: 4px; background-color: black; }')
# Place css sheet in '/var/www/musimatic/css' directory:
with open('/var/www/musimatic/css/index.css', 'w') as f:
f.write(content)
f.close()
def main():
random_number = random.randint(1, 100)
if random_number < 50:
print('HEADS! Revert back to the grey purple color scheme!')
create_css_sheet_with_grey_purple_scheme()
elif random_number > 50:
print('TAILS! Let\'s change the color palette!')
random_palette = grab_lospec_palette()
create_css_sheet_with_lospec_palette(random_palette)
if __name__ == "__main__":
main()
Here's my 'ArtGalleryCreator' Python 3 project, which is an art gallery page that literally creates itself:
import os
from pathlib import Path
from pathlib import PurePath
from pathlib import PosixPath
import pprint
import itertools
from wand.image import Image as wand_image
import wand
import pendulum
def create_thumbnails():
print('CALLING create_thumbnails() FUNCTION...')
art_gallery_path = '/var/www/musimatic/images/ArtGallery'
os.chdir(art_gallery_path)
picture_directories = list(filter(os.path.isdir, os.listdir(art_gallery_path)))
for directory in picture_directories:
print('Checking for thumbnails directory')
thumbs_path = str('/var/www/musimatic/images/ArtGallery/' + str(directory) + '/thumbs')
print('thumbs_path: ' + str(thumbs_path))
# Check if a thumbnails directory exist
thumbs_path_exists = Path(thumbs_path).exists()
if thumbs_path_exists:
print('thumbs_path_exists is true: thumbnail directory exists')
# if not thumbails directory:
if not thumbs_path_exists:
print('thumbs_path_exists is false: thumbnail directory does NOT exist')
# mkdir thumbnails
# https://csatlas.com/python-create-directory/
Path(thumbs_path).mkdir()
# Create globs for each file type
picture_paths_jpg = (x.resolve() for x in Path(directory).glob("*.jpg"))
picture_paths_png = (x.resolve() for x in Path(directory).glob("*.png"))
picture_paths = itertools.chain(picture_paths_jpg, picture_paths_png)
picture_paths_strings = [str(p) for p in picture_paths]
# Cycle through each picture_path string
print('Cycling through each picture_path string')
for picture_path in picture_paths_strings:
# Use PosixPath() to split path parts accordingly
current_filename = PosixPath(picture_path).name
current_stem = PosixPath(picture_path).stem
current_parent = PosixPath(picture_path).parent
print('current_filename: ' + str(current_filename))
print('current_stem: ' + str(current_stem))
print('current_parent: ' + str(current_parent))
thumb_image_version = str(str(current_parent) + '/thumbs/thumb_' + current_filename)
# https://www.geeksforgeeks.org/python-check-if-a-file-or-directory-exists/
thumb_image_version_exists = Path(thumb_image_version).exists()
print('thumb_image_version: ' + str(thumb_image_version))
print('thumb_image_version_exists: ' + str(thumb_image_version_exists))
# if not thumbnails/image.ext:
if not thumb_image_version_exists:
print('Creating new thumbnail image...')
# create_thumbnail(path_to_image, thumbnail_path)
# with Image(filename = picture_path) as image:
# https://www.geeksforgeeks.org/wand-thumbnail-function-python/
with wand_image(filename = picture_path) as image:
with image.clone() as thumbnail:
thumbnail.thumbnail(175, 150)
thumbnail.save(filename=thumb_image_version)
def create_thumbnails_gifs():
print('CALLING create_thumbnails() FUNCTION...')
art_gallery_path = '/var/www/musimatic/images/ArtGallery'
os.chdir(art_gallery_path)
picture_directories = list(filter(os.path.isdir, os.listdir(art_gallery_path)))
for directory in picture_directories:
print('Checking for thumbnails directory')
thumbs_path = str('/var/www/musimatic/images/ArtGallery/' + str(directory) + '/thumbs')
print('thumbs_path: ' + str(thumbs_path))
# Check if a thumbnails directory exist
thumbs_path_exists = Path(thumbs_path).exists()
if thumbs_path_exists:
print('thumbs_path_exists is true: thumbnail directory exists')
# if not thumbails directory:
if not thumbs_path_exists:
print('thumbs_path_exists is false: thumbnail directory does NOT exist')
# mkdir thumbnails
Path(thumbs_path).mkdir()
# Create globs for each file type
picture_paths_gif = (x.resolve() for x in Path(directory).glob("*.gif"))
picture_paths = itertools.chain(picture_paths_gif)
picture_paths_strings = [str(p) for p in picture_paths]
# Cycle through each picture_path string
print('Cycling through each picture_path string')
for picture_path in picture_paths_strings:
# Use PosixPath() to split path parts accordingly
current_filename = PosixPath(picture_path).name
current_stem = PosixPath(picture_path).stem
current_parent = PosixPath(picture_path).parent
print('current_filename: ' + str(current_filename))
print('current_stem: ' + str(current_stem))
print('current_parent: ' + str(current_parent))
thumb_image_version = str(str(current_parent) + '/thumbs/thumb_' + current_filename)
thumb_image_version_exists = Path(thumb_image_version).exists()
print('thumb_image_version: ' + str(thumb_image_version))
print('thumb_image_version_exists: ' + str(thumb_image_version_exists))
# if not thumbnails/image.ext:
if not thumb_image_version_exists:
print('Creating new thumbnail gif image...')
# Taken from this SO post:
# https://stackoverflow.com/questions/9988517/resize-gif-animation-pil-imagemagick-python
# TODO: Create thumbnail versions of GIF images
def main():
print('CALLING main() FUNCTION...')
with open('/var/www/musimatic/pythonprojectwebsites/ArtGallery/artgallery.html', 'w') as f:
f.write('<!DOCTYPE html>')
f.write('<html>')
f.write('<head>')
f.write('<title>Art Gallery</title>')
f.write('<meta charset="utf-8"/>')
f.write('<link rel="stylesheet" href="https://musimatic.xyz/css/artgallery.css" type="text/css"/>')
f.write('<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fancyapps/ui@4.0/dist/fancybox.css"/>')
f.write('<link rel="shortcut icon" type="image/ico" href="favicon/artpalette.ico"/>')
f.write('</head>')
f.write('<body>')
print('CREATING LEFT MENU')
f.write('<div id="left_menu">')
f.write('<h1>Art Gallery</h1>')
f.write('<a href="http://www.musimatic.xyz">BACK TO HOMEPAGE</a>')
current_date_eastern = pendulum.now('America/New_York').format('dddd, MMMM D, YYYY')
current_time_eastern = pendulum.now('America/New_York').format('hh:mm:ss A')
f.write('<p>Last Time Updated:</p>')
f.write('<p>' + str(current_date_eastern) + ' at ' + str(current_time_eastern) + ' EDT</p>')
art_gallery_path = '/var/www/musimatic/images/ArtGallery'
os.chdir(art_gallery_path)
picture_directories = sorted(filter(os.path.isdir, os.listdir(art_gallery_path)))
for directory in picture_directories:
picture_directory_anchor = str('<a href="#' + str(directory) + '">' + str(directory) + '</a>')
f.write(picture_directory_anchor)
f.write('<br />')
f.write('</div>')
print('CREATING IMAGE GALLERY FOR RIGHT SIDE')
f.write('<div id="right_art_gallery">')
print('WORKING ON CREATING IMG TAGS')
for directory in picture_directories:
picture_directory_header = str('<h1 id="' + str(directory) + '">' + str(directory) + '</h1>')
f.write(picture_directory_header)
f.write('<br />')
# SO Post on Globs:
# https://stackoverflow.com/questions/4568580/python-glob-multiple-filetypes
picture_paths_jpg = (x.resolve() for x in Path(directory).glob("*.jpg"))
picture_paths_png = (x.resolve() for x in Path(directory).glob("*.png"))
# TODO: Once I fix the 'create_thumbnails_gifs()' function, return to this:
# picture_paths_gif = (x.resolve() for x in Path(directory).glob("*.gif"))
# picture_paths = itertools.chain(picture_paths_jpg, picture_paths_png, picture_paths_gif)
picture_paths = itertools.chain(picture_paths_jpg, picture_paths_png)
# SO Post on string replacement:
# https://stackoverflow.com/questions/9452108/how-to-use-string-replace-in-python-3-x
# picture_paths_strings = [str(p).replace('/var/www/musimatic/', 'https://musimatic.xyz/') for p in picture_paths]
picture_paths_strings = [str(p) for p in picture_paths]
# pprint.pprint(picture_paths_strings)
for picture_path in picture_paths_strings:
current_filename = PosixPath(picture_path).name
current_stem = PosixPath(picture_path).stem
current_parent = PosixPath(picture_path).parent
regular_image_version = str(picture_path).replace('/var/www/musimatic/', 'https://musimatic.xyz/')
thumb_image_version = str(str(current_parent) + '/thumbs/thumb_' + current_filename)
thumb_image_version = str(thumb_image_version).replace('/var/www/musimatic/', 'https://musimatic.xyz/')
print('thumb_image_version: ' + str(thumb_image_version))
picture_img_tag = str('<a data-fancybox="gallery" href="' + str(regular_image_version) + '" data-fancybox="' + str(current_filename) + '" data-caption="' + str(current_filename) + '"><img src="' + str(thumb_image_version) + '"/></a>')
f.write(picture_img_tag)
# Sealing off right side of page's div tag for the image gallery portion:
f.write('</div>')
f.write('<script src="https://cdn.jsdelivr.net/npm/@fancyapps/ui@4.0/dist/fancybox.umd.js"></script>')
f.write('<script type="text/javascript" src="https://musimatic.xyz/js/artgallery.js"></script>')
f.write('</body>')
f.write('</html>')
print('ART GALLERY COMPLETE!')
if __name__ == '__main__':
create_thumbnails()
# create_thumbnails_gifs()
main()
August 29, 2021
Been going through Greg Vilppu's drawing courses, and learned a bit of figure drawing.
However, I will have to really go through his anatomy courses to figure out more in depth on how to actually draw the head, torso, arms, legs, etc. This is more so because that particular teacher knows his anatomy inside and out so its kind of hard to follow along when I've been guessing, since its been so long since I ever took an anatomy course anyway.
I uploaded my newer sketchbook on the 'Art Gallery' page as well, and some of the results are pretty good. I even uploaded older art as well, and let's just say, most of it is not that great, but I guess there's some progress to be made. My goal is to eventualy make some cool concept art, and do some lithography via linocuts, etc, and eventually oil paintings.
Art really has been helping me deal with stress these days, more so than playing guitar or keys ever did. Though, I did take a more refined approach to keys recently, and have been going through the Hanon and Czerny exercises which have helped a lot. Also, I've been just focusing on literally one song a week and haven't been rehearsing anything else to just get stuff up to 90 BPM and moving on. This is more so since I don't have much time for it in the morning, but its something I do right before work, and something to still look forward to these days.
I have been working on that second JeeveSobs album as well, and the songs are pretty much done. I just have to mix, and master it and it should be just fine. Overall, still guitar heavy, but I'm planning to mix it like some kind of Pinback album.
I should get back into my guitar building project too as well soon since I still need to apply clear coat to the guitar itself.
Maybe next weekend, we'll see.
~ Sam
August 15, 2021
I spoke too soon.
That self-creating art gallery page was great, but the amount of images, and the default sizes are CRAZY big.
Therefore, I had to incorporate creating thumbnails into the logical process as well.
I tried many, many, many different attempts to resize the GIF's without success.
I have no idea how to resize the GIF's. I tried the 'resize2gif' library, and even tried to follow two vague Stackoverflow posts to manually hack the module's code to work with Python 3 without matrix array errors. Ultimately, its not worth the hassle.
I'll just have to figure out the GIF's portion section another time.
Most likely, I will have to take the first frame of a given GIF, save that as an image, and then repeat the process of creating thumbnails from those images.
Gallery page found here:
Latest code attempt here:
import os
from pathlib import Path
from pathlib import PurePath
from pathlib import PosixPath
import pprint
import itertools
from wand.image import Image as wand_image
import wand
def create_thumbnails():
print('CALLING create_thumbnails() FUNCTION...')
art_gallery_path = '/var/www/musimatic/images/ArtGallery'
os.chdir(art_gallery_path)
picture_directories = list(filter(os.path.isdir, os.listdir(art_gallery_path)))
for directory in picture_directories:
print('Checking for thumbnails directory')
thumbs_path = str('/var/www/musimatic/images/ArtGallery/' + str(directory) + '/thumbs')
print('thumbs_path: ' + str(thumbs_path))
# Check if a thumbnails directory exist
thumbs_path_exists = Path(thumbs_path).exists()
if thumbs_path_exists:
print('thumbs_path_exists is true: thumbnail directory exists')
# if not thumbails directory:
if not thumbs_path_exists:
print('thumbs_path_exists is false: thumbnail directory does NOT exist')
# mkdir thumbnails
# https://csatlas.com/python-create-directory/
Path(thumbs_path).mkdir()
# Create globs for each file type
picture_paths_jpg = (x.resolve() for x in Path(directory).glob("*.jpg"))
picture_paths_png = (x.resolve() for x in Path(directory).glob("*.png"))
picture_paths = itertools.chain(picture_paths_jpg, picture_paths_png)
picture_paths_strings = [str(p) for p in picture_paths]
# Cycle through each picture_path string
print('Cycling through each picture_path string')
for picture_path in picture_paths_strings:
# Use PosixPath() to split path parts accordingly
current_filename = PosixPath(picture_path).name
current_stem = PosixPath(picture_path).stem
current_parent = PosixPath(picture_path).parent
print('current_filename: ' + str(current_filename))
print('current_stem: ' + str(current_stem))
print('current_parent: ' + str(current_parent))
thumb_image_version = str(str(current_parent) + '/thumbs/thumb_' + current_filename)
# https://www.geeksforgeeks.org/python-check-if-a-file-or-directory-exists/
thumb_image_version_exists = Path(thumb_image_version).exists()
print('thumb_image_version: ' + str(thumb_image_version))
print('thumb_image_version_exists: ' + str(thumb_image_version_exists))
# if not thumbnails/image.ext:
if not thumb_image_version_exists:
print('Creating new thumbnail image...')
# create_thumbnail(path_to_image, thumbnail_path)
# with Image(filename = picture_path) as image:
# https://www.geeksforgeeks.org/wand-thumbnail-function-python/
with wand_image(filename = picture_path) as image:
with image.clone() as thumbnail:
thumbnail.thumbnail(50, 50)
thumbnail.save(filename=thumb_image_version)
def create_thumbnails_gifs():
print('CALLING create_thumbnails() FUNCTION...')
art_gallery_path = '/var/www/musimatic/images/ArtGallery'
os.chdir(art_gallery_path)
picture_directories = list(filter(os.path.isdir, os.listdir(art_gallery_path)))
for directory in picture_directories:
print('Checking for thumbnails directory')
thumbs_path = str('/var/www/musimatic/images/ArtGallery/' + str(directory) + '/thumbs')
print('thumbs_path: ' + str(thumbs_path))
# Check if a thumbnails directory exist
thumbs_path_exists = Path(thumbs_path).exists()
if thumbs_path_exists:
print('thumbs_path_exists is true: thumbnail directory exists')
# if not thumbails directory:
if not thumbs_path_exists:
print('thumbs_path_exists is false: thumbnail directory does NOT exist')
# mkdir thumbnails
Path(thumbs_path).mkdir()
# Create globs for each file type
picture_paths_gif = (x.resolve() for x in Path(directory).glob("*.gif"))
picture_paths = itertools.chain(picture_paths_gif)
picture_paths_strings = [str(p) for p in picture_paths]
# Cycle through each picture_path string
print('Cycling through each picture_path string')
for picture_path in picture_paths_strings:
# Use PosixPath() to split path parts accordingly
current_filename = PosixPath(picture_path).name
current_stem = PosixPath(picture_path).stem
current_parent = PosixPath(picture_path).parent
print('current_filename: ' + str(current_filename))
print('current_stem: ' + str(current_stem))
print('current_parent: ' + str(current_parent))
thumb_image_version = str(str(current_parent) + '/thumbs/thumb_' + current_filename)
thumb_image_version_exists = Path(thumb_image_version).exists()
print('thumb_image_version: ' + str(thumb_image_version))
print('thumb_image_version_exists: ' + str(thumb_image_version_exists))
# if not thumbnails/image.ext:
if not thumb_image_version_exists:
print('Creating new thumbnail gif image...')
# Taken from this SO post:
# https://stackoverflow.com/questions/9988517/resize-gif-animation-pil-imagemagick-python
frames = images2gif.readGif(picture_path,False)
for frame in frames:
frame.thumbnail((100,100), Image.ANTIALIAS)
images2gif.writeGif(thumb_image_version, frames)
def main():
print('CALLING main() FUNCTION...')
with open('/var/www/musimatic/pythonprojectwebsites/ArtGallery/artgallery.html', 'w') as f:
f.write('<!DOCTYPE html>')
f.write('<html>')
f.write('<head>')
f.write('<title>Art Gallery</title>')
f.write('<meta charset="utf-8"/>')
f.write('<link rel="stylesheet" href="https://musimatic.xyz/css/artgallery.css" type="text/css"/>')
f.write('</head>')
f.write('<body>')
art_gallery_path = '/var/www/musimatic/images/ArtGallery'
os.chdir(art_gallery_path)
picture_directories = list(filter(os.path.isdir, os.listdir(art_gallery_path)))
for directory in picture_directories:
picture_directory_anchor = str('<a href="#' + str(directory) + '">' + str(directory) + '</a>')
f.write(picture_directory_anchor)
f.write('<br />')
print('WORKING ON CREATING IMG TAGS')
for directory in picture_directories:
picture_directory_header = str('<h1 id="' + str(directory) + '">' + str(directory) + '</h1>')
f.write(picture_directory_header)
f.write('<br />')
# SO Post on Globs:
# https://stackoverflow.com/questions/4568580/python-glob-multiple-filetypes
picture_paths_jpg = (x.resolve() for x in Path(directory).glob("*.jpg"))
picture_paths_png = (x.resolve() for x in Path(directory).glob("*.png"))
# TODO: Once I fix the 'create_thumbnails_gifs()' function, return to this:
# picture_paths_gif = (x.resolve() for x in Path(directory).glob("*.gif"))
# picture_paths = itertools.chain(picture_paths_jpg, picture_paths_png, picture_paths_gif)
picture_paths = itertools.chain(picture_paths_jpg, picture_paths_png)
# SO Post on string replacement:
# https://stackoverflow.com/questions/9452108/how-to-use-string-replace-in-python-3-x
# picture_paths_strings = [str(p).replace('/var/www/musimatic/', 'https://musimatic.xyz/') for p in picture_paths]
picture_paths_strings = [str(p) for p in picture_paths]
# pprint.pprint(picture_paths_strings)
for picture_path in picture_paths_strings:
current_filename = PosixPath(picture_path).name
current_stem = PosixPath(picture_path).stem
current_parent = PosixPath(picture_path).parent
regular_image_version = str(picture_path).replace('/var/www/musimatic/', 'https://musimatic.xyz/')
thumb_image_version = str(str(current_parent) + '/thumbs/thumb_' + current_filename)
thumb_image_version = str(thumb_image_version).replace('/var/www/musimatic/', 'https://musimatic.xyz/')
print('thumb_image_version: ' + str(thumb_image_version))
picture_img_tag = str('<a target="_blank" href="' + str(regular_image_version) + '"><img src="' + str(thumb_image_version) + '"/></a>')
f.write(picture_img_tag)
f.write('</body>')
f.write('</html>')
print('ART GALLERY COMPLETE!')
if __name__ == '__main__':
create_thumbnails()
# create_thumbnails_gifs()
main()
~ Sam
August 14, 2021
Hard work pays off.
Though the CSS styling for this page is far from complete and needs a lot of work, the idea is fulfilled:
Basically, I created an entire art gallery page that creates itself.
How it works:
- A Python 3 script creates the page by iterating through the related image directory on the site, and creates an HTML page.
If you like Python 3, and source code, well, this is for you, since this is how I did it so you can do it on your site as well:
import os
from pathlib import Path
import pprint
import itertools
def main():
with open('/var/www/musimatic/pythonprojectwebsites/ArtGallery/artgallery.html', 'w') as f:
f.write('<html>')
f.write('<head>')
f.write('<title>Art Gallery</title>')
f.write('<meta charset="utf-8"/>')
f.write('<link rel="stylesheet" href="css/artgallery.css" type="text/css"/>')
f.write('</head>')
f.write('<body>')
art_gallery_path = '/var/www/musimatic/images/ArtGallery'
os.chdir(art_gallery_path)
picture_directories = list(filter(os.path.isdir, os.listdir(art_gallery_path)))
for directory in picture_directories:
picture_directory_anchor = str('<a href="#' + str(directory) + '">' + str(directory) + '</a>')
f.write(picture_directory_anchor)
f.write('<br />')
for directory in picture_directories:
picture_directory_header = str('<h1 id="' + str(directory) + '">' + str(directory) + '</h1>')
f.write(picture_directory_header)
f.write('<br />')
# SO Post on Globs:
# https://stackoverflow.com/questions/4568580/python-glob-multiple-filetypes
picture_paths_jpg = (x.resolve() for x in Path(directory).glob("*.jpg"))
picture_paths_png = (x.resolve() for x in Path(directory).glob("*.png"))
picture_paths_gif = (x.resolve() for x in Path(directory).glob("*.gif"))
picture_paths = itertools.chain(picture_paths_jpg, picture_paths_png, picture_paths_gif)
# SO Post on string replacement:
# https://stackoverflow.com/questions/9452108/how-to-use-string-replace-in-python-3-x
picture_paths_strings = [str(p).replace('/var/www/musimatic/', 'https://musimatic.xyz/') for p in picture_paths]
pprint.pprint(picture_paths_strings)
for picture_path in picture_paths_strings:
picture_img_tag = str('<a target="_blank" href="' + str(picture_path) + '"><img src="' + str(picture_path) + '"/></a>')
f.write(picture_img_tag)
f.write('</body>')
f.write('</html>')
if __name__ == '__main__':
main()
~ Sam
July 19, 2021
I found a few art gallery pages worth stealing design ideas from, which include William L. Eaken's illustration page:
Love the layout, how each thumbnail reveals a corresponding page. I could probably accomplish this but would have to create automatically generated pages for each and every artwork I have. No way would I do this by hand, but its definitely possible with a CronTab job Python 3 script though.
Just thinking of some good layouts to replace the art gallery page with, aka here's my current work in progress using the "CSS Grid Layout", though I would much rather a left sidebar. Will have to rework it a bit, and also make it just somehow have two columns, one for the menu, the second column for the rest of the content, aka time to revisit CSS styling ideas I barely remember, fun times:
Might steal the entire design of this site as well, we'll see:
~ Sam
July 18, 2021
I have failed a lot in quite a few projects lately.
So, ultimately, this is a message more so on how to fail, and more or less how to accept it.
Things I have failed at for the past month:
- I just can't figure out how to get Emacs Tramp Mode to work for 'gcp' boxes for work to make my work life easier.
- My artwork-based WordPress site just wouldn't work due to the "White Screen Of Death" issue, despite so many attempts to fix it so I nuked it.
- I tried to to use "PrivateInternetAccess" VPN on a headless Fedora Server which ended up not working at all, but did allow me to request a complete refund.
However, there are a few takeaways that were positive, and DID work out:
- I tweaked my Emacs config heavily for work to improve a few things (discover-my-major, log4j, multiple-cursors, aggressive-indent, SX-Mode, counsel-grep-or-swiper, csv-mode, dockerfile-mode, docker-compose-mode, webpaste.el, docker.el)
- I got the cable management in my office room to where I want it, with plans to move the desktop to the other wall with keyboard stands for the synths.
- I was able to make toltott kaposzta (Hungarian stuffed cabbage), and goulash for the first time in the last month, and they came out great comparatively.
- I've installed Manjaro Linux on my second SSD on my Desktop computer to utilize for KVM virtual machines.
- I setup Retroarch with DOSBox Pure on the Devuan Desktop HDD, and its been fun chilling out with old school DOS games every once in a while.
- I've learned some basics of Blender for 3D modeling so that I can get good reference models for art projects.
- I bought an LDAP reference book (Understanding and Deploying LDAP Directory Services) and the Networking All-In-One Dummies books as references for work.
- I learned how to use Elfeed for RSS feeds in Emacs as an alternative to Newsboat.
- I'm slowly working on painting my Fretwire kit guitar over time.
- I finished making the Dell Optiplex from eBay into a Fedora Server file server and Git server.
- I figured out how to deploy SAMBA on the same Dell Optiplex to host PS2 games over ethernet that I can play via the hacked PS2.
- I updated the Webring with quite a few more links (https://musimatic.xyz/webring.html)
- I am pretty much down to a short list of 4 things I'd still like to do, which is crazy compared to the giant list I've had this year
What I'd like to do:
- Deploy a minimal artwork gallery page, preferably with thumbnails (something like this: https://sibylleszaggarsredford.com/gallery-simple-thumbnail-page/)
- I might also have to utilize some JS or a crontab job to pull of the art gallery page correctly (https://stackoverflow.com/questions/28778048/get-image-from-json-file-using-javascript-and-display-in-html-img-tag)
- Or, I might have to use 'lxml' Python 3 library to pull in images successfully (https://lxml.de/lxmlhtml.html)
- More importantly, the related WIP progress for the newer art gallery page will be found here: https://www.musimatic.xyz/artgallery.html
- Figure out how to incorporate Grafx2 and Blender together with a good scanner workflow for an improved art workflow.
- Make plans to revisit the idea to deploy my own mail server idea in probably 6 months from now once I'm better with networking concepts.
What I've learned through an entire year of documenting projects:
- Ultimately, I'm an ideas guy at heart, and am weirdly over-technical on things that probably shouldn't be.
- I'm also an art and music type guy, and should be doing things more focused towards that at the end of the day.
- I follow through projects, even if they fail, and am good at documenting the journey.
- Somehow, I will use this as an advantage in my later career if I ever grow past doing tech support.
- I am looking forward to the day when I can combine all my interests into one career.
Here's a cool video that blew my mind a few weeks ago, and has had me thinking differently on how to really incorporate art into projects:
- Creative Computation – Jack Rusher (https://www.youtube.com/watch?v=TeXCvh5X5w0)
~ Sam
June 6, 2021
I forgot to mention this, but the other day, I fixed the 'w3mBookmarkSorter' so that it now is able to take a user's config file, read it, and then determine where their given w3m related 'bookmarks.html' file is so that it can sort it alphabetically:
This is an important utility in my opinion since w3m lacks the functionality to sort bookmarks in this fashion.
I might consider joining their mailing list and just asking them to consider adding my project directly into w3m because I know the last time I had asked about how to contribute to the Debian part of w3m, I was told it basically takes forever for something to get approved.
I spent a bit of time over the last two days, but I was able to get the Dell Optiplex 7010 SFF machine up and running as a server, specifically for backups and as a Git server. I chose to run Fedora Server on it, since I've been using that for my Linux laptop, and its pretty decent.
The only weird issue so far is that it doesn't remember the BIOS settings to specifically boot into UEFI Mode to select the USB harddrive containing Fedora Server, so that's been annoying. I'm not sure what I can do in that regards. It most likely is an issue with the motherboard related battery burning out or something. I can't think of any other reason why the BIOS settings are never remembered.
On the art side of things, I spent some time scanning in an old sketchbook from 2020 / 2021, so I plan on deploying a Wordpress site to house a 2021 based art gallery within a few weeks or a month. Let's just say I'm glad I don't do art in pencil that much anymore as its way too annoying of a medium to begin with, especially when scanning.
Pretty happy with the way things are going, and always thinking of new fun projects.
~ Sam
June 4, 2021
I changed jobs recently, and am now working for a cybersecurity firm as a Technical Support Engineer.
The job itself is really interesting, and the product itself is really neat. It's a lot to learn, but I think I'm ready at this point of my life. Let's just say I spent the last week learning how to use a Mac, and figuring out how to get the best version of Emacs up and running for my daily note taking, which in itself, wasn't too easy. Though the MacPorts version is better than the Brew install version, I'm still running into this weird zoom issue comparatively, but as always, its nothing that a good change to the Emacs config can't work out.
I have been working on deploying my own email address via a Vultur VM with OpenBSD, so that's been a new experience in itself. Never used any of the BSDs before so it was pretty interesting how the default 'ksh' Korn Shell works.
I fixed the "Pizzatime" Wordpress site, so now images appear as they should on posts due to an annoying permissions issue I was encountering. This means that I can continue to create more pizzas as I have some crazy fusion food ideas in mind:
With this in mind, I probably will work on deploying an 2021 based art gallery as a separate Wordpress site, as well as an "Inconsolation" ripoff site that forces me to use Debian purely in a Getty based TTY shell, which will be enlightening to say the least, and will probably put so-called "Linux minimalists" who still boot into X11 with cringy window managers like 'DWM' to shame.
I switched over to Fedora Server Linux, which is awesome in itself. Its like a better Debian with more up-to-date packages, plus the installation process is a breeze compared to Gentoo. Gentoo was cool, but I don't really want to modify kernel configs just for my daily laptop usage. I would come back to Gentoo on an older machine that needed it for pure optimization but the time spent on trying to get things up and running is such a time sink. People who insist upon Gentoo, and Arch, etc, don't have normal day jobs and or other important time commitments. Otherwise, I just don't see how you could fathom spending 5+ hours to figure out how to actually get it working without tapping into IRC. Sure, you learn a lot, but man, sometimes I kind of wish I got the time back just so I can work on better projects instead.
I basically use all my machines like Emacs machines these days so whatever gets me going to keep my workflow improved is what wins. No editor comes close (okay, maybe Spacemacs but yeah that's an exception since I do like Vim and Emacs equally).
I created a server rack using the IKEA Lack Rack trick, see this video for reference as its super easy and cheap to do:
- €25 CHEAP IKEA DIY Server Rack (https://www.youtube.com/watch?v=ARkn_VHzFQg)
I bought an old Dell Optiplex 7010 SFF on eBay to rescue an older machine. What I didn't realize is that I can only really put a 2.5" inch and a 3.5" HDD in there, so I plan on just using a WD RED drive for the 3.5" drive, and a standard consumer grade Seagate drive for the 2.5" drive. To be consisent, I'm also planning on putting Fedora Server on that machine as well as I'm only going to use it for backup purposes and possibly a Git server.
I'm still in the process of doing a ton of cable management for the home office room, but have a while to go as I'm debating if I should get 2 monitors or an ultra-wide monitor instead for both work AND my personal Desktop machine as well. Yeah, I might turn into that guy who has like 4 monitors per machine, but hey, one step at a time.
I plan on figuring out how to use the FreeMCBoot memory card I bought for my old PS2 a few weeks ago as well, and accessing games via a local SAMBA share (most likely via the Dell Optiplex). Its crazy cool to see how far PS2 modding has come along.
I'm planning on building the guitar kit I bought from "TheFretwire.com". Its gonna be cool with a purple blueberry burst ink finish. Just have to sand it in the driveway first one of these weekends and apply individual coats of the ink at a time. Might make a corresponding Wordpress site if I get in the habit of building more guitars.
And I've taken up a bit of gardening but let's just say I think I've overwatered the plants a bit and have learned my lesson :/
What's funny is that the soil that did land on the ground did start to sprout some of the things I planted, so here's to re-planting :)
~ Sam
April 13, 2021
Took a few months, but I'm happy to have released that JeeveSobs album called "Jagged Edges" today, which can be found here:
Pretty happy with the results.
Second album has been worked on for a while, and is more drum and loop focused which should be cool too.
Stay tuned.
~ Sam
March 23, 2021
I was able to deploy my own Searx instance, which means that I have my own search engine.
This means goodbye to Google and DuckDuckGo, I'm using my own search engine from now on.
You can use it too here, have fun:
~ Sam
March 20, 2021
It took a lot of effort and some research, but I was able to get the 'weather' page working again, as I had realized that the National Weather Service moved their Apache server hence why my weather radar GIFs that I ripped using 'wget' weren't working for a while.
They replace their main page with some dumb bloated web app, but luckily you CAN find the related weather radar GIFs if you look hard enough.
For example, if you wanted to look up a New York City based weather radar GIF, you have to instead look for a local forecast here:
You then can right-click the image itself, and view it in a separate tab:
If you go up a level, you can try to find your area:
However, the easier approach is just to find your local area first with a normal GUI browser, and then rip the GIF accordingly. This is because I think they name these radar images based on the weather station they're obtaining it from, or the local airport with a weather radar, etc.
I had to do this for each of the weather pages:
- https://www.weather.gov/okx/
- https://www.weather.gov/ohx/
- https://www.weather.gov/bgm/
- https://www.weather.gov/tbw/
The end result can be seen here:
For fun, I also added cloud coverage based GIFs from NOA's GOES Image Viewer page too:
~ Sam
March 13, 2021
I revamped my VPS and Raspberry Pi within the last couple of days.
Also, I added a random crontab job that changes the color scheme of the front page every 5 minutes for fun. The results are cool, and here to stay. Might even make it more wacky with some interesting background images, who knows.
I did some deep thinking and yeah, I'm not gonna host any bloated web apps on the site since I really feel like I just don't want to showcase any more bloat on the web anymore. However, I might make some cool useful autogenerated pages though, as I have a few more ideas up my sleeve.
Still working on that JeeveSobs album, but mostly just mixing at this point. Debating putting it on cassette tape for a limited release of like 10 to 20 tapes max. Will need a tape deck from eBay though, but it'll be a cool thing to learn, and I already have the album design done anyway.
I modded my Nintendo Switch as well, and its pretty neat, though I don't often play games that much anymore. I've been more focused on just knocking out some life based todo list stuff in terms of goals, and have felt a lot more well-rounded.
I've been practicing guitar once a day again instead of just the weekends, and honestly, its been a blast. It's like I never stopped years ago, and I've been just going over what the best teacher (Pebber Brown) has to offer on his YouTube video playlist, which is here for reference:
I've been itching to make my own guitar from parts, so I might do this with a premade double-cut guitar body with a pre-made neck, and figure out how to spraypaint it, etc.
I've been slowly becoming more interested in the Amiga itself. In terms of Amiga OS variants, I found quite a few, which are showcased in the following links:
- "AROS" which is the "Amiga Replacement OS"
- https://aros.sourceforge.io/pictures/screenshots/
- "ApolloOS Free 68K OS (AROS based) running on Amiga Vampire 4 Standalone":
- https://www.youtube.com/watch?v=Wqv_4IciF2U
- Build an Aros based Amiga computer from scrap parts Part 1
- https://www.youtube.com/watch?v=ttylXRD1S1I
- Why use Amiga in 2011? (AmigaOS 4, MorphOS, AROS, AmigaOS 3.9)
- https://www.youtube.com/watch?v=s1RsvEm7UrU
I've been debating what Linux distro to use instead of Gentoo because I kind of find the whole kernel updating process a bit annoying. I'm not sure, but something about it doesn't excite me really. I kind of want the OS to be minimal, dependable, and NOT to be Windoze or Mac. Also, waiting for compilation of packages doesn't really help either. Might change to Slackware instead, but have been looking for Gentoo derivatives for the time being.
Here's what I found:
- Gentoo Studio (I really like how so much is included in terms of audio DAW stuff, would totally put this on the Desktop)
- https://gentoostudio.org/
- CloverOS
- https://gitgud.io/cloveros/cloveros#what-is-cloveros
- Funtoo
- https://www.funtoo.org/Welcome
- Redcore Linux (Looks a bit try-hard, or weird, but I like what it aims to do)
- https://redcorelinux.org/
- Sabayon Linux
- https://www.sabayon.org/
In terms of my desktop computer, I tried adding this cool Macintosh theme to my "Just Werks" Devuan desktop, but the theme just didn't work right, even with MATE desktop:
At this point, I might just keep using JUST LxQT desktop with Openbox and focus on that since it can do tiling window management if needed.
I've decided on a couple of good email server names, so I might deploy that within the next couple of months since I really would love to host my own email. I kind of got fed up with proprietary ones like MS Outlook or Yahoo! always complaining when I use the UBlock Origin extension (https://addons.mozilla.org/en-US/firefox/addon/ublock-origin/) to block tons of ads. This just makes it all the more important to just take out those service providers from my workflow since if they're basically selling all my data just for a "free" service, they're just not worth it.
~ Sam
January 23, 2021
I have been mostly tweaking my Emacs configuration for the past couple of weeks, both on my personal config and my work-based config.
Let me tell you, it is awesome to be able to utilize Emacs Window Manager inside a VM, along with 'magit-mode' for git commits, and just all of the small tweaks I've added after watching all of Uncle Dave's Emacs videos.
I think I will blow some people's minds at work once my full setup is complete so that I can maybe get them to possibly use it too (though… I doubt it, but one can hope I guess).
I'll be happy when I get work email, as well as access to both ZenDesk API and JIRA Mode enabled so I can have a full-fledged setup present.
His Emacs video playlist can be found here:
I can say without a doubt, that my efficient for work has improved 10x with some of the things I added.
I added a ton of games to my 3DS via some cool Virtual Console injector sites that host QR codes for downloads.
Here are some tweaks I made to the site:
- I also tweaked the recipes on this site to be a bit better to use, as they weren't being rendered properly.
- I increased the font-size for the "blog" page to '20px' to be more readable.
- I also increased the font-size for the main homepage as well to be more readable.
- I tried re-designing the front page's styling to be a bit more simple, but I'll have to keep thinking on how to make it more usable.
- I stole the Dark Theme from some GitHub Gist page for my 'git.musimatic.net' site (https://gist.github.com/Yoplitein/f4b671a2ec70c9e743fa).
- I made the art present on the 'art.html' page look more consistent in terms of the sizes of the images present.
- I removed the lingering Bootstrap CSS styling present from the 'music.html' page.
- I added 'BACK TO HOMEPAGE' hyperlinks on each of the pages.
Regarding the recipe-based rendering issue, I do blame some of the recipes themselves. Some of them either dragged on and rambled when a real recipe should instead only be directions. Nothing makes me more annoyed these days than having to sift through a giant blog post in order to get a food recipe, though I've learned most of these annoying types of sites usually has the recipe at the bottom anyway, so its a 50/50 I guess.
I plan on re-doing my CV instead to be in 'Org-Mode' and NOT LaTeK because LaTeX is bloated, and unnecessary. I will find it hilarious if I can make it look 100x better than some Vim user with the bloated LaTeX install. Sure, Emacs is bloated in its own way, but its tailored to my taste. That's what makes it awesome and function so well as it integrates so nicely with itself. More so, the final point is that I don't HAVE to learn LaTeK to produce a nice looking resume to be exported in PDF, or even an HTML site, with a few keyindings in Emacs Org-Mode :).
I've been reading Steve Howe's autobiography as I've been on a reading kick again. Autobiography books are great. There's really nothing else like it, and I've only did nothing BUT learn from other people's lives.
I plan on reading the one from Bill Bruford too sometime as well. Maybe even the one from Gretzky too, why not.
I bought some cables and adapters to hook up my Baofeng UV-5R to 'svxlink' which is basically Echolink, but for Linux terminal, which is pretty sweetl.
Other than that, I've been working on that 'JeeveSobs - Jagged Edges' album, and it has been coming along pretty nicely. I have the song order in place, though during my last time on that Desktop, I think I might have nuked the ALSA config by accident that I tweaked specifically for that Focusrite Solo Interface… so it will probably take a bit of digging to figure out how to get it to work again, most likely involving the 'aplay -l' command to list devices again and see how to really make sure the devices are being listened to on the hardware level :/
I'm still torn on what specific isolated power supply I would like to my guitar / synth pedalboard, but I'm leaning towards this one from MXR in particular:
~ Sam
January 1, 2021
I archived all older blog posts back into the main "blog.html" page, and have removed all pages and references to the older versions of the site, as I already have done a personal backup of the page itself.
If you ever wish to see the previous versions of the sites (though sometimes incomplete), you can visit the Archive.org archived page here to view different scraped versions of this site from 2018 to 2020:
- https://web.archive.org/web/20180801000000*/musimatic.net
- https://web.archive.org/web/20190501000000*/musimatic.net
- https://web.archive.org/web/20200701000000*/musimatic.net
I was debating making a "museum" section to showcase the older versions of the site. But honestly, it's better to just remove as much bloat from the page as possible.
I think the 'git.musimatic.net' should be the main source page to shine for something like that, and I would rather allow a given user to be able to easily download utilities in one-click (with the caveat that it would only work in Linux anyway).
I'm still 50/50 on totally removing any bloated web app projects I have done in frameworks like AngularJS, React, etc. I might section them off to a part of the site called "bloat.musimatic.net" to poke fun on how bloated these same technologies are. One thing for certain is that I will be removing any "Bootstrap" CSS style link references from any of the pages, so get used to the Geocities looking pages.
This is because:
- I do not care if you are accessing this page on a mobile device.
- I do not care if you want to zoom in or zoom out all the way and don't want the page to break.
Here's what you can do:
- Keep your browser's zoom level at 100%.
- Stop using Windoze or MacOS on your personal computers.
- Start using Linux or a BSD derivative, and surf the web as God intended with a used laptop or desktop to prevent e-waste.
This is because I don't think the world should cave in to become mobile-friendly as I think non-rooted smart phones are the absolute worst. Even if you use it for calling, you should switch to just a landline phone and have a backup phone in your car for emergencies, that's it. Texting is pointless, and outdated since email does it better, and more. Having a phone and any associated account allows companies to mine your personal data, spending habits, location, etc. Granted, ISP's are probably doing the same thing anyway, you should be allowed to be "YOU" at all times without any of these unnecessary worries ontop of modern living.
With that rant aside, I feel great about the years to come as I plan on living my life in a more independent fashion. I am confident that with the right spending habits, and the right attitude on how to figure out next related steps that I will be able to raise a family in a positive manner.
As you can see, this blog part of the site has shown a lot of my efforts throughout its years of existence as to the hopes I've had to really incorporate some cool projects out there. Some have been fulfilled, others kind of didn't work out. On that front, I'm actually GLAD that they didn't.
Just in case you're wondering: No, I am not one of those awkward, ignorant boomers that think that "2021 will solve all of our problems" because it will only get worse. However, we can choose to live our lives our way, and today is an awesome day with God as we have lived to see it with the bare essentials to keep surviving.
That is the attitude you need to have to be happy these days!
Staying Active, And Grateful ~ Sam
December 29, 2020
I am happy to note that I have successfully moved over to Gentoo on bare metal, meaning its on my Linux laptop and no longer in a VM.
I have coupled Gentoo with Emacs Window Manager (exwm), and honestly, it is fantastic!
The fonts, and unicode characters need to be adjusted as I'm not sure if you have to RICE it via '~/.Xresources' or via the Emacs config itself (but this is what the README is for I guess).
However, I even have 'vterm' support as well within Emacs, so that's pretty much spot on goal-wise to even try it out.
Since I'm utilizing Emacs Window Manager though, I can easily just use urxvt as the main terminal emulator as well, which is awesome.
I decided to take the plunge as I was getting sick of using AwesomeWM for some reason. More so, because I just wanted a full screen window manager, and less bloat present. I think I'll also switch my "Just Works" setup to be "FreeBSD" based instead of Devuan as well. Devuan's cool and all, but honestly, I think BSD sounds even cooler since you can run both Linux AND BSD programs on BSD platforms through a compatibility layer.
I'm debating whether or not I should archive these posts from my blog or keep them as a running thing going forward to document the progress of how far I've come for the past few years. I think the historical record would be neat. I do though like starting fresh each year though.
I plan on overhauling the site completely for the new year, and will archive the other sections of the site to remove any unnecessary bloated sections. This includes removing any "Bootstrap" dependent pages. I'll keep their memories alive with a "museum" section to showcase screenshots of how the site used to look, but its a bit much to host 3+ versions of this site on the same webpage. This means that it will have a more basic look and feel, but will work better for anyone accessing the site via text-based web browser like 'Links', or 'w3m'. Also, I'm sick of Web 3.0 sites, and just want this page to look like an Geocities / Angelfire page as I've intended for quite some time this year.
I plan on having rotating color schemes that change each day as well, so it'll be fun to implement within a CronTab job. I will be hosting a "recipes" section from my recipe based .org files, and will include an awesome "links" section as well as I've harvested and archived quite a few awesome links to share. I realized that this idea alone is the single greatest idea from older sites, as the web was supposed to connect individual pages where people spilled their heart out to the world.
Instead… we have only a few major sites that control content for everyone else. I think the best thing we could all do is just make our own web pages again, and link them together to keep the true spirit of the internet alive.
I did a lot of self-reflecting, especially with what I've done with my projects and this site, and I have got to say that I have had the most fun making useful utilities. I still aim on improving the 'w3mBookmarkSorter' with a related config file to match. Though it won't be available in a major Linux distribution's package manager because the approval process takes forever, I think the right people will find it, and find it that much more useful. I maybe could have done more cooler Twitter based bot projects tapping into API's, but those are nichey platform specific ideas that most people won't even find useful in a few years from now. I would love to possibly consider how to tap into Archive.org's API to archive content though, as I think that will be a major step in helping preserve the internet before it becomes another paid cable service variant.
I still plan on hacking my Wii-U this week a bit more with a few more homebrew apps to run Wii and Gamecube backups as well, so that's been fun. I also plan on utilizing my Raspberry Pi as a NAS device, and also to use it for RTLSDR to listen to ham radio frequencies. I'll need the related adapters, but this should keep my interest for a bit before considering a DMR radio + PiSpot in a few months.
I've been plunking away at that album that I've worked on for quite a few months, and the main guitar and vocal parts are done. I have to add drums, and maybe some synth sections. Other than that, just basic mixing, and it should be good to go. I don't plan on re-hosting my music on this site because of how large the bandwidth would be to stream it, but I might host it on a separate VPS as I plan on hosting my own email, and re-host other web applications. I could still just store the files and people could freely access them as intended.
I'm still glad I have the ability to create music though, as I recognize not everyone has the patience to learn an instrument. However, I am living proof that if I can learn guitar, synth, vox, and drums by myself, then anyone can.
Keeping Myself Busy On Good Old' Gentoo :) (Very happy it worked out after the 5th install attempt this year D:)
Merry Christmas, and Happy New Year :)
December 20, 2020
I had to create a throwaway email to post a GitHub issue, as I can't stand Microsoft anymore, let alone GitHub. Yet, like all similar services, you NEED to have an account to raise an issue on some random GitHub project, so I had to figure out a way around this.
Luckily, there are some sites to easily make throwaway email accounts that get destroyed in 10 minutes or so, so here they are for a scenario like this:
There is another mail provider that provides you with basic free-tier email. Its doesn't last only 10 minutes, so this is a bit of an exception, but is better than having to be forced to create a Gmail account which eventually forces you to use two-factor authentication via your private phone number, which they have no right to obtain. This is the better alternative (for now):
I've assembled quite a list of sites to add to a 'Links' or 'WebRing' section to this site in the Geocities-style revision that will be released early next year.
Still debating if I should nuke the old variants of the site and just post screenshots of the design instead, as I wanted to use as little JS as possible going forward.
Here's To Throwaway Email Accounts And Cool 'Links' Sections :) ~ Sam
December 19, 2020
Check out this revival Geocities-esque site where you can browse other people's sites, cool stuff:
In my spare time, I've hacked my PS3 and Nintendo 3DS with custom firmware to load homebrew and similar applications.
The only thing left I would like to consider for the PS3 is how to figure out how to load .iso's via the NTFS backup external drive I have instead of having to transfer the games to a FAT32-formatted flash drive in such a jenky fashion. I'm pretty sure there's a Linux utility to convert to .iso, so I think it won't be too hard.
The result of the hacked 3DS has been awesome, since it gives you access to countless 3DS and DS game backups, etc. The only thing that sucks about the 'old' 3DS or 'o3DS' series, is that it's not that great at emulating older systems via Retroarch. There are standalone emulators, but it just isn't perfect performance. However, you CAN inject game ROM's into Virtual Console to allow for perfect emulation for GB, GBC, GBA, NES, Genesis, and Game Gear for even the 'o3DS'. It just takes time to do this via the homebrew tools out there for this purpose from 'gbatemp'.
I've also settled on a Voip setup with an old school phone and am pretty happy that I went this route. Cell phones sucks,and texting sucks. There's literally no reason why the same person who wants to send you a huge attachment of any kind via text can't literally open up a internet browser on even the weakest Android phone to send an email with a larger attachment size overhead. Plus, I prefer phone calls, and think anything that's like texting that ISN'T IRC or something is just a waste of time.
In terms of pet projects, I'm deadset on going the FreeBSD route, and have backed up my Devuan laptop accordingly. I'm a bit 50/50 on if I should keep babysitting the Gentoo VM since its a love/hate relationship.
Though most Linux enthusiasts online will nail you for it, the "Just Works" philosophy sometimes is just better. At the end of the day, when I boot a computer, I don't want to have to worry about it breaking when I opened it just to use it. I realized that I'm still the type of guy that uses the minimal ISO install anyway, so I'm not like some people who depend on Ubuntu like they're on life support, and in that respect, I do build my computers the way I want, so its not like I haven't made it my own.
However, I do love Gentoo for what it is. It's just a lot of work though, and it almost killed my SSD drive on the compiling alone since it went into read-only mode until I re-installed Devuan on it.
The only thing left I'm doing is debating what window manager to try going forward with the setup.
My current progression in the alternative OS cycle has been:
- Lubuntu (LXDE) > Debian (AwesomeWM + Openbox) > Laptop: Devuan (AwesomeWM), Desktop: Devuan (AwesomeWM, Openbox)
I'm considering any one of the following, but am tied between 'spectrwm', 'xmonad', or 'Qtile', so whatever has as an easy and minimal config will win since LuaScript (aka via AwesomeWM's config) sucks:
- i3 (https://i3wm.org/)
- bspwn (https://github.com/baskerville/bspwm)
- herbstluftwm (https://www.herbstluftwm.org/)
- xmonad (https://xmonad.org/)
- spectrwm (https://github.com/conformal/spectrwm)
- jwm (http://joewing.net/projects/jwm/)
- Qtile (http://www.qtile.org/)
- Ratpoison (https://www.nongnu.org/ratpoison/)
- dwm (https://dwm.suckless.org/)
Also, I'm debating what VPS provider to use next year to deploy my own personal email as well as what the name of the domain name should be.
Choices Are Always Good! ~ Sam
December 11, 2020
I haven't felt well at all stomach-wise for the last few days, but today, I worked through it to get back into a routine.
I did a lot of self-reflection today, and realized that all the goals I set aside for work-related things really actually came to fruition this year.
I still haven't decked out my Emacs work configuration as far as it can just yet, and even then, that's probably maybe even 15% of the potential I could probably experience with a finely tweaked Emacs setup. Even so, I was advised many times on IRC to really really really actually utilize Emacs Window Manager, so I might take the dive. However, despite all of this, I am pretty proud what I know now compared to what I knew two years ago. It's mindblowing looking back, and really positive as well as humbling at the same time.
I re-organized all of the Org docs on my Devuan machine, and the result looks great. Even the ~/hub directory looks awesome too. I have been debating how to really handle how to handle backups though for all of my stuff. Most likely I am going to utilize the Raspberry Pi 3 along with a 2 TB drive to jerryrig a poorman's LAN based NAS.
I got an SDXC card, and am also pretty excited to hack my old Nintendo 3DS soon too for homebrew and backups.
Might look into installing FreeBSD in a VM as I would like all my "Just Works" machines to be based in that. If its a Desktop machine, then I'll use Openbox as the window manager. If its a laptop, then its using AwesomeWM…
OR, I might look into seeing if there's a better lightweight alternative to AwesomeWM with an easier config file, as I DESPISE how it depends upon Lua script to configure it. The syntax sucks. I would rather learn more ELisp to tweak Emacs than to try to figure out how Lua script works.
Even more so, I am at the point where I am debating removing the top widget altogether, and just displaying the current numbered "Desktop" within Tmux and do full screen for each Desktop window.
I basically want the most minimal X-Org server based window manager that would just boot into X. Ideally so I can just focus on terminal apps, with maybe a GUI browser for stuff like banking websites and the like that depend upon sneaky JS scripts.
Some lightweight window managers I'm considering include:
- i3: https://i3wm.org/
- bspwm: https://github.com/baskerville/bspwm
- herbstluftwm: https://www.herbstluftwm.org/
- xmonad: https://xmonad.org/
- spectrwm: https://github.com/conformal/spectrwm
- jwm (Joe's Window Manager): http://joewing.net/projects/jwm/
- qtile: http://www.qtile.org/
- ratpoison: https://www.nongnu.org/ratpoison/
- dwm: https://dwm.suckless.org/
All I know is that one day, I hope to rival K. Mandela's site (https://inconsolation.wordpress.com/) in either content or accessibility and ideas to really push terminal apps, Emacs, Vim, and Linux / FreeBSD forward!
Hoping I feel better soon though, but happy to keep my mind occupied! ~ Sam
December 7, 2020
I tried using my work Windoze laptop to install the PS3 firmware hack, but realized that the dumb laptop doesn't allow flash drives to be used while booted into Windoze –> Into The Trash (Just kidding… but I wish I could just use Linux all day, one can dream).
I instead utilized the 'md5sum' Linux terminal utility that's built in to verify the 'md5sum' hash of the download, and just utilized pcmanfm-qt to mount the drive.
I then used the following command to format the disk into "FAT32" (or 'vfat' on Linux):
sudo mkfs -t vfat /dev/sdb1
I then listed all of the drives, including the USB flash drive, and verified that the command was successful:
lsblk -f
For reference purposes, I took the idea from this blog post, as I needed to figure this out quickly (because of limited patience, and since I just wanted a hacked PS3 ASAP), so I must give credit where credit is due, as its a pretty good post:
After much attempts, I was able to get PS3 backup games running just fine with the "Multi-Man" utility, so it's been pretty awesome on that respect.
I plan on also hacking the 3DS later this week with this guide and a new SDXC card:
Speaking of emulation, this page itself goes over what "No-Intro" rom sets were all about, and is worth a read as I'm aware of early ROM dumps of games that had title screens (annoyingly) but its funny how the same project that aims to remove them included the very same people that put them into ROMs in the first place:
I am also debating the following home based VoIP phone services:
- https://www.1-voip.com/
- https://www.axvoice.com/
- https://www.ooma.com/home-phone/
- https://www.phonepower.com/voiphome.aspx
- https://voipo.com/
- https://www.vonageforhome.com/
Keep Having Fun! ~ Sam
December 5, 2020
Enjoying surfing the net this fine Saturday morning, and I came across a few cool things in terms of old-school Geocities-esque pages.
This is a cool designed "Neocities" site that I absolutely adore the color and design of, never thought to put the navigation pane on the far right:
This is a search engine you can use to find older pages:
Found a page that imparts the old-school tropes of all those 90's pages too. I forgot about the visitor counter, and background MIDI music, good idea ;)
Found a cool tech reference page as well:
That reminds me… I should probably start working on the site re-design to de-clutter this site again for the next re-design. Looking forward to it!
For the website re-design, I do plan on including a "Recipes" section in which I literally have a .org files for any of the food recipes I often refer to (which contain links to the actual people who made them for author credit purposes). Since .org files are pretty readable on its own, I don't think I'll need to convert to .pdfs either, since that would take a lot of space. However, it would convince a lot of people interested in learning how to cook Hungarian food to also utilize Emacs Org Mode, so its a Win-Win :)
Other than that, I was debating if I should hack the PS3 first, or figure out how to run Wii, Gamecube, and Wii-U backups on the hacked Wii-U I have.
Since I've been taking a mind vacation from doing anything major in terms of pet projects, I got a lot of great ideas for the "Sieges" side band, and plan on really exploring the potential of the Dungeon-Synth genre. There's probably going to be a lot of spoken word tropes with heavy reverb, and continuous motifs to match.
Really looking forward to getting my work-based Emacs config to get email working as that will help my daily workflow so much.
Keep Having Fun!
~ Sam
December 1, 2020
I created a separated config for Emacs for work, which is working out pretty nicely.
So far, I have Slack working in my work-based Emacs, which is really cool.
You can find out more about how to use 'Emacs-Slack' on their GitHub page:
Slack sucks on its own to be honest. It is a dumb proprietary ElectronJS app that wants to be IRC so badly, but had to settle for being a commonplace item in most workplaces. It's better than Google Suite tools I guess, but it's still based in good ol' spooky Chromium, so you can't really trust it either. Especially since you don't control the logs –> your employer does. More so, with the trend of everything being a layer of a Chromium browser, you start to realize Google really has a stranglehold on a lot of software out there now unfortunately.
Ideally, my entire work-life would be encapsulated within Emacs, so one could dream. I plan on taking stabs at this each week, ex: Got Slack working this week, next week: work e-mail, the following week: ZenDesk's API via a Python 3 shell in Emacs, the following week: JIRA Mode in Slack. The reason why you would want to do something like this is that you could manage your work tasks all in one place, with the multiple buffers available to you so that you can easily copy and paste, and move around between them without EVER having to use your mouse. It's beautiful ;).
Later, I might incorporate Emacs Window Manager into the mix to get an internet browser into the mix, but I think I'll be happy if I get the basic workflow improved for my current job.
Speaking of Emacs, the Emacs Conference last week was pretty sick. Check out the pre-recorded videos here (I plan on checking out Rainer Konig's Org-Mode talk in particular):
Other than that, I've been taking it easy. I got a used PS3 recently, and have been having a lot of fun. I plan on hacking it to put some emulators and copied games on it, and other cool utilities. Also, I might beef up the Wii-U to play some Gamecube and Wii ISO's as well, since I already hacked it a while ago with the "Haxchi" hack.
I have been doing art on Tuesdays, so I got further into the Loomis book I have. I studied and referenced bone anatomy, which is coming along, though I have more fun sketching from random images or ideas to be completely honest.
I'm still debating how to best showcase my art from this year, and going forward. There's gotta be a lightweight "gallery" type app I can deploy for this reason alone since my current implementation is ok… but not exactly what I would want.
Still thanking God for every day that I have the basic necessities, and am thankful to have had a good Thanksgiving week off. Really had a good time as I made a ton of Hungarian food since family is so far away these days.
I'm still making topic ideas for YouTube vids, and will be recording a few screencasts with most likely 'ffmpeg'. The topics are probably going to be on Linux, FreeBSD (and BSD derivatives), as well as audio production as I don't see too many people online that help with lower hardware, or just good sources of information on how to teach yourself some of the techniques present. I'm not saying I'm an expert, but there are definitely things I have learned from doing things myself, and I feel that a lot of people could benefit from having easily accessible Free-As-In-Freedom alternatives to bigger proprietary programs to empower them on a daily basis.
And yeah, maybe get the occasional sponsored hardware review to smack Linux or FreeBSD on wouldn't hurt either ;)
More so, if anyone like myself could learn and install Gentoo, I think at that point, ANYONE can (and should if they want to :D).
FreeBSD is pretty cool, and can be found here:
You basically can install whatever you want, especially with the minimal install, so its like Linux in a lot of ways but a bit better in terms of where the project is going. You can also run a lot of Linux software ON FreeBSD as well, so its a win-win for me in a lot of ways. I've been debating making the plunge, but want to grow deeper into the Gentoo VM I had going, and swap to Slackware possibly before going to FreeBSD.
Keep Staying Positive, And Keep Your Life Balanced With Fun Side Projects!
~ Sam
November 21, 2020
I got my Gentoo VM up and running again, but its the classic scenario where you can't necessarily follow the Gentoo AMD64 handbook verbatim, as I had made the mistake in making '/dev/sda2' or /boot as 'ext2' when you need to make it 'vfat' to handle the UEFI based boot process.
I had to adjust this by moving everything from /boot to /home, reformatting /boot to be with:
mkfs.vfat /dev/sda2
Then, I re-installed Grub 2, and it worked thankfully after rebooting. I also enabled 'dhcpcd' this time around, so that networking is handled for me automatically.
That had to be like the 4th time I installed Gentoo (for reference, the third and fourth time were successfull). So, it's not like I didn't learn anything. I learned so much in how Gentoo works with its "emerge" tool, as well as how to merge changes between files. It's a pretty exciting process in the weirdest way, but then again, I'm really a Gentoo fan from the deepest part of my heart, and loathe Arch. The main reason is that Gentoo FROM THE GET-GO offers you the ability to choose to create a Linux system without 'systemd' while you have to use a fork of Arch to even give you this option. This fact alone makes Gentoo that much more based.
However, this did not come without a few losses as I did unfortunately lose all my Gentoo installation notes that I made since the stupid SSD that went into read-only mode is exactly where I stored the notes like an idiot. Honestly, SSD's are such a meme, but unfortunately they are the future of drive storage.
As a result to kind of circumvent this issue from happening again, I've vowed to only make anything OTHER than the main Linux laptop to have one-way Git repos meaning they should only PULL changes, and not do any upstream changes.
I also did some re-organizing on my local Linux laptop to have a "hub" section to house all my repos and directories in one spot. I'm debating how I might use this kind of idea to also host my Org-Mode notes on my site so I can interact with them between machines easily. I've been getting into really thinking and writing down different tasks for at-home projects, or even just pet projects, and it really really really has kept me focused on what I can do now, vs. what I can do another time.
I did a lot of research into cable management as well since I still have the world's most giant Ethernet cable running throughout the house, so there are a few options to fix this, as well as the multitude of audio cables for music stuff in the home office room too. I prefer it this way because most Wi-Fi drivers on Linux simply run on non-Free software (Free As In Freedom), so you can't trust any of the non-free drivers as there's always the potential of it working against you. So, just use an Cat 7 ethernet cable, and your problem is solved. Luckily there are a few ways to help manage cables like this, so I am happy to gauge what what options are the best for this scenario.
I found this dude's site since he's a guy from Freenode IRC that is into "Software Defined" Radio, which is pretty neat. Love the site's easy layout, and might steal some good design layouts, and I like how easy it is, especially with the left and right sides of the page:
Speaking of software defined radio, it's not that expensive to get into it, and you don't even need a Ham Radio license to listen in since its all about RECEIVING signals and not transmitting (Yes, there are transmitting-capable SDR's too, but they're boomer-tier priced, and its better to just use the cheaper option listen in anyway for this kind of thing).
Here's the more budget friendly option to listen to Ham Radio bands since you can stick this near a window sill, and you're good to as you just need to plug it into your computer's USB port and utilize it with related free radio software:
Here's the boomer-tier priced one I mentioned earlier that can transmit, but it ain't cheap:
Here's a list of RTL-SDR compatible software for Windoze and Linux:
On a total separate note, I've been debating getting a used Sony PS3, since the games are super cheap, and I would love to play some of the "Warriors" series ports again, as the Sony ports were always superior. More and more, I've become okay with game consoles being just better than PC's, as the modern Windoze PC spies on you anyway, and I prefer just single player offline games not connected to the Internet from time to time.
The deals on eBay for old used PS3 games are pretty enticing, I must say.
I might look into stealing more cool ideas from Emacs configs from the likes of "Uncle Dave" from YouTube, as well as Sacha Chua, both of which have deep knowledge of Emacs inside and out. Here are their configs for reference:
- https://github.com/daedreth/UncleDavesEmacs
- https://awesomeopensource.com/project/daedreth/UncleDavesEmacs
- https://pages.sachachua.com/.emacs.d/Sacha.html
Looking forward to maybe getting EXWM aka Emacs Window Manager up and running as well as X-Org running on that Gentoo install, as I wanted to "live" within that Gentoo VM for 6 months before transferring the entire setup from a virtual machine to bare metal hardware. I just don't want to take the jump necessarily yet because I don't want to lose any data if I screw up along the way, and also, since I'm trying to ween into being that kind of elevated super type of Linux user.
Planning to use this Gentoo guide as a reference for the X-Org setup, as I'm kind of a noob that's a bit too used to making a login manager like 'lightdm' do this for me automatically, but I'm looking forward to figuring out how to make X-Org work with 'startx' and then figure out how to tweak it to my needs:
Ideally, I would want to boot into X, which would then boot into Emacs via Emacs Window Manager so that everything contained within each buffer could interact with Emacs seemlessly.
Some really cool YouTube videos that show how awesome this is include the following:
- https://www.youtube.com/watch?v=MquoGuU8sHM
- https://www.youtube.com/watch?v=GJjjflU67tE
- https://www.youtube.com/watch?v=Gk9-q8tXbMs
Keep Having Fun Tweaking Your Setup!
~ Sam
November 20, 2020
I finally uploaded my 'w3mBookmarkSorter' project to my Git repository, which can be found here:
You can 'git clone' the repo with the following command so you can use the utility locally on your Linux machine:
git clone https://git.musimatic.net/w3mBookmarkSorter
Further installation instructions can be found in the "README.md" file included in the project.
It's a pretty cool little simple utility that sorts the bookmarks from A to Z for 'w3m' as the functionality just doesn't exist yet. Is it slightly bloated because its based on Python 3? MAYBE, but some programming languages like Python 3 just have better libraries for this kind of idea straight from the box, so its worthwhile for someone who needs this done quickly.
I DID note that there is an HTML parser from 'w3.org' themselves, which helps maintain the internet standards for the 'www' protocol which seems kind of neat, and light-weight:
We'll see if its worth a re-write later using this utility instead. However, I'm too quick to just make a Python 3 based webscraper due to old habits, so honestly, I can't blame myself for it at this point.
I tried playing around with the '/etc/cgitrc' config for the Cgit instance of my Git repository to include the following config settings, which I'll have to see if they actually worked for the Git repository itself. The goal was to enable downloads, and to have syntax highlighting to easily read any code that is present:
# Enable ASCII art commit history graph on the log pages
enable-commit-graph=1
# Allow download of atr.gz, tar.bz2 and zip-files
snapshots=tar.gz tar.bz2 zip
# Highlight source code with python pygments-based highlighter
source-filter=/usr/lib/cgit/filters/syntax-highlighting.py
# Format markdown, restructuredtext, manpages, text files, and html files
# through the right converters
about-filter=/usr/lib/cgit/filters/about-formatting.sh
Ideally, I'm literally shooting for copying the same kind of ideas from the Cgit creators' own repository for 'cgit', since they have easy accessible "Download" links, and "Clone" instructions:
Anyway, got some major wins today on those two points alone. There's definitely something that can be learned from my 'w3mBookmarkSorter' project: Keep your project scope small, keep it useable, and easy to use.
Here's To Making Awesome Utilities For People To Use To Improve Their Workflow!
~ Sam
November 19, 2020
I debated how to properly deploy the 'w3mBookmarkSorter' project, and it turns out that it'll be a hard uphill battle on how to package it for the Debian release. This is important because the major 'w3m' fork, even on GitHub, is for Debian itself. The project itself is based in C, while my plugin uses Python 3 with the BeautifulSoup4 library.
That being noted, I'm most likely going to just create an alt account on GitHub to host stuff as well, but also mirror it on my own Git page as well.
I've been assembling ideas in Org-Mode docs for what I want to learn, and this has helped me a TON for focusing on what I want to learn for work based skills, and especially in building my own current Linux skills.
I am really understanding that to better learn anything new, its better to consult the man page or the Arch Wiki, which is hilarious for someone like myself that refuses to run anything Arch based in the first place. However, the Arch Wiki is an amazing resource, so I think its something I'm going to learn towards. Too often I quickly try to search for something on DuckDuckGo when I could have easily just tried to find it myself.
For example, I'm trying to learn LaTeX so I can re-create my own resume in LaTeX because I think modern WYSIWYG editors are trash, and I want a resume that's not dependent on Microsoft products. I had to install a package called "texlive", which is apparently a distribution of LaTeX. That being noted, I tried to do the most obvious thing, and tried to just do:
man texlive
This yielded nothing for me.
Then, I looked into more resources on how to take advantage of man pages (manual pages), and figure out what manuals actually exist on your system. I found out that the 'apropos' utility is your friend in this scenario.
So, to use the previous example, we can pipe that into 'less' so that you can have nice readable output, and figure out what man page to actually use:
apropos tex | less
Using this, I was able to see many, many, MANY LaTeX related resources that already existed on my system.
And then… I figured out I could just look at the 'man' page for 'latex'…
But hey, I learned something in the process, and in doing so, I answered my own question in terms of what specific 'man' page I can refer to.
Found a cool site totally by accident as I was looking for games to improve my Vim skills:
It's websites like this that make me want to copy their look, style, and feel. The functionality of that site itself is inviting, and just overall easy to manage.
Definitely will be taking notes to improve the "Geocities"-esque overhaul later this year.
Here are some cool 'Vim' based games to check out too that I found:
I found out about 'Vim' games through this crazy, but informative talk on how to play Vim like an instrument, and YES, this guy actually uses sounds for his Vim macros, which is nuts, cool, and awesome at the same time:
I've learned a lot today in terms of Linux shell commands, especially how to utilize the 'man' command to reference manuals or 'man' pages.
I've also made a schedule of video topics to possibly do screencasts for. I think I'll do YouTube videos like 'gotbletu' without showing a webcam, as I would rather the content to be the primary feature being presented. I also plan to backup these same vids to Internet Archive and to PeerTube just in case as well given the state of YouTube these days.
However, I only want to do informative, helpful, and just fun screencast videos on Linux, BSD platforms, music creation, and neat pet projects to inspire others. Maybe even stuff on Ham Radio, mesh networks, etc., would be awesome too.
I also want to deploy my latest band's album on my own site too to not depend on any platform like Bandcamp. That has been a lot of fun to work on, and I'm up to maybe around 4 decent songs at the moment with a 3 or 4 other ones in very early stages. It's a lot of acoustic and vocals so far, which is pretty new to me as I used to never like acoustic until recently.
Lots and lots of goals, and only so much time.
Good thing there's Org-Mode to organize it all ;)
~ Sam
November 16, 2020
I finished the 'w3mBookmarkSorter' project. Its beautiful, and works just as intended.
I am debating to mail this to the 'w3m' team as a patch plugin for 'w3m' via email as I think anyone who uses 'w3m' would absolutely LOVE this functionality, as w3m doesn't sort bookmarks at all by default.
Emacs Conference 2020 is coming up next weekend (Thanksgiving weekend) and I'm looking forward to some of the talks, especially the talks with Rainer König, who has some amazing Org-Mode tutorials on YouTube, and RMS (Richard Stallman).
Here's Emacs Conference 2020 for anyone interested, as its virtual this year:
These are Rainer's awesome Org-Mode tutorial YouTube videos:
Keep on going! ~ Sam
November 15, 2020
I tried tweaking my Gentoo VM to be able to add a different "profile" to allow it to download the [20] desktop specific option. The reason for doing this is so that I can add the necessary "USE" variable flags to be able to install xorg-server, so that I can install Emacs Window Manager. During this entire process, the SSD drive gave in, and went into read-only mode, which freaked me out to say the least, because I thought the drive died on me. I vowed to never buy into the SSD meme again, but I did re-set the power and SATA cable, and that seemed to fix the issue, even though I have to re-install Devuan on that particular drive, and re-do the entire Gentoo install. Apparently that dumb 'read-only' issue is typical for SSD's unfortunately, so it's something you just have to live with. Just so that SSD drive doesn't go into read-only mode again, I actually might just stick with trying FreeBSD in a VM instead and to go with FreeBSD going forward, and never to try Gentoo again.
I think what I learned out of that experience is that distros like Gentoo or Slackware might put too much stress on harddrives, so even though its cool to learn the underlying components and to "tweak them" accordingly, someone like myself would be better off using something that's bleeding edge, but works better straight from the box.
Installing Gentoo is kind of like the process of maintaining Arch for me, where it literally takes hours trying to figure out what's broken or how it even works in the first place. I realized as I've gotten older that I just care about the pet projects, and as long as the underyling OS isn't spying on me and is working as intended, my level of caring stops there. I want to customize, but don't want to spend an entire weekend trying to wait to get something to work.
The biggest thing I've learned is that I have got to backup my data once a month to my spare external HDD just in case.
On a more positive note, I've been assembling more and more Org docs with todo lists, and am planning to possibly make a Wiki out of them and to place them onto my site.
I re-learned a lot of Vim through 'vimtutor', and am still amazed on how good Vim is comparatively.
I've come to the realization that I'll keep Emacs for Org mode TODO lists, but am more interested in pursuing more plugin options with Neovim instead.
I worked on the w3mBookmarkSorter project a bit, and thought of how to more effectively sort the links present, and will apply the same idea to the actual project sometime this week.
I also got my ElectronJS project for work to actually compile again, so I plan on learning either more NodeJS on the side, or go through a bunch for ElectronJS videos to figure out how to effectively add a feature to use HTTP requests to pull logs for one of the work apps I help support within the ElectronJS app itself.
I am in the process of learning how to use "Jami" on Linux, and will do a test run on Windoze 10 with Firefox so that family members who depend on proprietary operating systems could also use it, as I'm trying to find the best VOIP or messaging solution that's secure, and cross-platform. This is more so because I hate having to spend minutes upon minutes for a feature phone when I have unlimited internet that can do the same exact features. Will do more Windoze related testing later this week. On Linux, "Jami" is run by using "gnome-ring", since that was the name of the project before the name change to "Jami" a few years ago.
'Jami' can be found here:
Looking forward to Thanksgiving this year as we're planning on doing some Vietnamese and Hungarian food, as the usual turkey is kinda boring and we wanted to do something different this year.
Still going strong
~ Sam
November 5, 2020
I worked on the 'w3mBookmarkSorter' project, and made a lot of headway by just re-thinking and reducing the amount of Python 3 code present.
I think I'm pretty close to what I want, and will most likely release utilities like this on a public Git instance as I think 'w3m' as a whole could benefit from something like this.
Though I like Emacs a lot, I realized its much more fun and simpler to just use terminal apps instead. I'm done more fighting with Emacs to even try to get it to run shells, as it just doesn't do proper, fully-feature shells out of the box. I don't want to have to patch Emacs just to get a shell working on it.
Sure, there's "eshell", "shell", etc, but the only decent one, "VTerm", seemed usable only if you re-compile Emacs from source, which is a pain if you want to distribute it amongst multiple machines like I do.
I don't mind seeking to learn how to compile programs from source on distros like Gentoo, but for my current workflow, I just want it to be up and running ASAP. Especially if I'm primarily using Emacs as a text editor, and not an all-in-one utility. Its this lack of shell support straight from the box that makes me want to instead configure Neovim instead, as I still love 'tmux' so much.
I will still configure Emacs to help my Org-Mode workflow for everyday ticket work though, and still look forward to using it for that reason. However, even more on this, I even don't want to use Evil-Mode (Vim keybindings) on Emacs anymore either because I think the keybindings overall just fight you from step 1 when you try to do this. I think the best way to use Emacs is just to use it as intended, and just use packages to make it that much easier to use.
Either way, Vim and Emacs still beats using a mouse for text editing. ;)
I've worked more on the re-design of the site, and it's looking more and more what I want it to be in terms of it being simple to look at, and more Geocities based. You can check it out here:
- www.musimatic.net/geocities.html
I've been watching movies to chill out this week mostly, and haven't done too much art honestly.
Also, I saw that Parabola is moving to BSD in a few years because of dependency on "Rust", "systemd", and even "Pulseaudio" being forced on the Linux kernel level. Definitely will start checking out FreeBSD in that case within a VM, and using that as the daily driver instead of Devuan honestly. The cool thing about the BSD's is that you can totally run any Linux program you want with a compatibility layer, so I could still use AwesomeWM, Openbox, etc, without a single problem. Related Parabola announcement can be found here:
Keep your mind active, be thankful, and God Bless.
~ Sam
October 31, 2020
I deployed my 'cgit' instance here:
- git.musimatic.net
I basically replaced the existing 'GitWeb' instance with 'cgit', which is really nice. All it needs is a dark theme, and I think it should be fine.
I tweaked my Emacs config a bit, and its looking pretty good so far. I'm pretty impressed with just the simply default zoom based Hydra command for example since I really like the idea behind it to just keep repeating the same macro until another key is pressed.
Helm is pretty cool, but the colors with the somewhat default Emacs theme looks a bit jenky, so I might have to tweak it a bit. Also the "Ranger" implementation from MELPA kind of sucks, and makes me want to instead utilize a shell within Emacs for all my 'tmux' based programs to be pretty honest.
I kind of hate 'dired' mode as its not really inviting, intuitive, or helpful in terms of what features it can provide. I think Ranger is far superior to this, so I think the best thing I could do is to somehow run 'tmux' in a shell and go from there.
I played around with the Geocities re-design of the site, and I'll admit, its pretty fun going back to basics in terms of CSS styling. Bootstrap, although nice looking for some startup websites, is a bit too much, and in itself kind of generic if you think about it.
I think my best approach would be to just make the new Geocities site mobile friendly first (or I guess, just not care about mobile) and then size it up from there.
I tried to install Emacs Window Manager on Gentoo, but didn't realize that my networking device had reverted from 'eth0' to some randomized interface name, which is a bit annoying. I had to modify the Grub init file to allow the ethernet cable network adapter to just be named as 'eth0' to resolve the connection issues. With that in mind, I'll have to try EXWM another day.
I've been debating what IRC server to install. I'll have to probably use 'Unrealirc' for the IRC server itself, or 'ngIRCd':
In terms of the front-end IRC webpage, I think I'll probably choose one of the following:
~ Sam
October 30, 2020
I'm in the process of deploying 'cgit' to git.musimatic.net via nginx.
The one important link command to create a symlink in this kind of scenario, let's say "default" would be:
sudo ln -s /etc/nginx/sites-available/default /etc/nginx/sites-enabled/default
It's not working yet, but I do understand the concept of what I'm doing in terms of making sure nginx understands to listen on Port 80, and then run 'fcgiwrap' to actually deploy the website showing the git repository. I did install it from source by using the related tarball.
I know the end result will look awesome, so it's worth the effort.
I tried working on the one 'w3mBookmarkSorter' project, but I couldn't get my head around how to really fix the underlying issues present. The output doesn't really look like the desired format. I'm wondering at this point if instead I could just treat blocks of HTML tags within the bookmark html file in the BeautifulSoup4 parser. I do use 'w3m' quite a bit so it would be a cool utility to have, and I might even fork 'w3m' to add it as an additional script.
I might work more on the ElectronJS app for work to feel a bit more productive. I ran into an issue earlier this week where I got some obscure JS compilation error, but I blame Windows honestly since it was a Microsoft based error. It MIGHT be because of the use of 'puppeteer-in-electron' dependency though as a lot of the related forum posts on the related error are for obscure PATH variable references.
I try to get Emacs Window Manager working on that Gentoo VM this weekend. Will also keep at it in terms of drawing, as I'm getting more and more comfortable with Andrew Loomis style wireframes, though they're pretty crude and hard to do at this point for me.
We'll see.
~Sam
October 28, 2020
I am looking into how to deploy "cgit" instead of my current GitWeb instance, as I never seem to use it anyway, and kind of think it sucks.
There's something a bit uninviting for it, especially if you just have it present to show dotfiles or something else in a public repo.
Here's the site for 'cgit':
I did find a blog post on the same topic that I might rip from if I can't figure out to get it working on 'nginx' myself though. I do still plan on building it from source as I don't see a Debian based port unfortunately:
Here's a related example article from 'nginx' on deploying a FastCGI to host something like 'cgit':
An even neater thing to note is the 'cgit' creator's own website:
I absolutely love how he just displays his projects in a never ending page, that basically links to just cgit repos for each project. That is PERFECT. I plan on trying to do the same thing once I get my 'cgit' instance working.
His own 'cgit' instance is awesome too, and I love the idea of mirrors at the bottom as well:
One minor 'wget' command I learned today to archive entire sites is the following command:
wget -r -np -nH -R index.html
The parameters that it uses include (from the 'wget' man page):
- -r: recursive: Retrieve recursively
- -np: no parent: Do not ever ascend to the parent directory when retrieving recursively
- -nH: no host directories: disable generation of host-prefixed directories
- -R: reject list: Specify comma-separated lists of file name suffixes or patterns to accept or reject
You can use this to archive your own site from a Linux bash terminal.
I've been debating what mail program to deploy for my own email next year, as I want to move away from the paid service I've been using. It's okay, but I want the challenge of doing it myself.
Since I already pay for a separate VPS for this site, and paid for a year of service for the other paid email provider, I think it makes more sense to just then beef up this VPS instead.
On that note, I might even jump to a different VPS provider like Vultur or something.
These are the email clients I've been debating to deploy:
The only downside to hosting your own email is that you have to nuke spam emails from orbit with the right tools.
Some of the best tools I've seen include the following:
Have fun archiving sites, and deploying your own email.
~ Sam
October 27, 2020
I looked into FreeBSD, and wow, I am impressed. It pretty much can run most Linux software in its own compatibility layer. This includes window managers, and other key things I use on a daily basis.
Here's some great vids on the topic of using FreeBSD as a Desktop-centered OS:
I think I might even swap out my go-to Devuan setup for just a FreeBSD setup to run Linux based VM's though I'll have to try out FreeBSD in a VM first. Also, I'll probably get into this if I get bored of my Gentoo install as well.
I saw this website randomly, and I really like how he has his web apps setup, so I might steal this kind of design for the "Apps" section of all my web app projects for the re-design of this site:
I found out about that previous site because that same person is the one that re-designed the Org Mode website, which looks awesome by the way, and WAY better than the previous site:
Found out about "Binder mode", and this looks awesome as well. Definitely would try this in terms of binding together several free-flowing idea type notes. Would be great in the context of songwriting lyrics, etc.:
I bought a used NUX Solid Studio pedal the other day, so I'm looking forward to using it on the album I've been working on.
I've been debating a keyboard amp or guitar amp setup, and I think this would be a great alternative for the time being, as I primarily just record music and play music at home anyway. I can finally use the amp-in-a-box type pedal I've had laying around, and put it right in front of the NUX Solid Studio, and then use an existing multi-fx pedal I have for the effects section.
I also plan on slowly building a pedalboard from some of my existing pedals, but also a separate one dedicated to loops and effects.
I cheaped out a while ago, and got two Ikea Hejne shelves for like around $10, and voilah, two full size pedalboards. Just have to get some more velcro tape and I'm set. It's hilarious how much money you can save on things like this, as the metal variants are about $60 to $100 more, especially if we're talking about the Pedaltrain Pro type pedalboards.
I learned about this trick from this vid, so I would check it out if you're interested in creating a pedalboard on the cheap:
More and more, I've decided that instead of potentially building or buying a cheapo or used guitar, I'll just mess around with my existing Tele clone guitar. I will most likely have to buy a soldering iron later on, but I think this would be my entry point into electronics and instrument building to be honest. I will most likely swap out the neck pickup, and the underlying electronics as I'm interested in the GFS Kwikplug system to easily swap out pickups on the fly.
I think the idea of getting more pedals is because I've been watching more and more guitar pedal demo videos just to relax. This channel in particular is pretty awesome on this topic:
- https://www.youtube.com/watch?v=kgDlf_Pp6os
- https://www.youtube.com/watch?v=1-edx1_Vn4g&t
- https://www.youtube.com/watch?v=jXgoLbXqDYU
Some of these guitar pedal designs are so beautiful and inspiring. I honestly wish I could make some to demo out and have some fun with, and somehow make them interact with the internet in weird ways.
I've been also on the hunt for more VSTs or plugins to use in Reaper. I found this one to be a pretty cool one, as it emulates the Super Nintendo's Yamaha SPC700 sound chip. I'll see if it'll play nicely in Carla on Linux based Reaper. Worth a shot though:
A really good reference on the SPC700 soundchip, as well as related Super Nintendo music is this site. Definitely worth checking out for the simple site design alone:
Here's to still having fun making music.
~ Sam
October 24, 2020
I mostly chilled out today and did some art.
Here's a cool link to a site that features color cycling art from Mark Ferrari that you can experience in your browser. You can change the time of day, and observe the constant shift of color palettes as a result. Really neat and inspiring scenes:
Hope everyone is well. ~ Sam
October 21, 2020
I began working on the "Yahoo Finance Stock Ticker App" project.
I'm planning on assigning a "Scrapy" based agent to each of the pages, and to consistently return the same data feed.
Here is a link to the related "Scrapy" Python 3 module:
I'm using this instead of BeautifulSoup 4 as I'd like multiple agents to send asynchronous requests instead of just one at a time.
I'd like maybe deploy this with some kind of lightweight backend program like 'Flask' in combination with React to make it sort of a hybrid between Python 3 and JS.
We'll see how it turns out :)
I've learned how to use the 'venv' Python 3 command to store a virtual environment for this project as well, as I usually have had the bad habit to just pull in dependencies for cron job projects.
With this approach, you can easily deploy the same project to multiple platforms without having to run a million pip3 commands to make sure that dependencies are pulled in, since 'requirements.txt' takes care of that for you.
To get started, make sure you first 'cd' into your project:
cd /path/to/your/project
Here's the command to get started using 'venv' to create a virtual environment for your Python 3 project by utilizing the 'python3 -m' parameter so that we can focus on utilizing the script from one specific module, which is 'venv', aka the first instance of 'venv' in the following command. NOTE: The second 'venv' parameter can be named whatever you want, though usually, its a convention to name any Python 3 virtual environments as'venv':
python3 -m venv venv
Here's the major 'venv' command to activate a virtual environment once you have entered your project directory:
source venv/bin/activate
Once you activate your virtual environment ("venv"), then use 'pip3 install' and install any required packages. In my case, I did 'pip3 install scrapy' for example so that I can work with that module for this project.
Here's the related command to store all the 'pip3' packages into 'requirements.txt':
pip3 freeze > requirements.txt
The most important thing is to NOT add your 'venv' to your actual .git directory if you plan on using 'git' as your version source control method, as you only need 'requirements.txt', and the user just needs the 'requirements.txt' file to build the 'venv' themselves.
Here's the command to exit the 'venv' virtual environment:
deactivate
Here's another scenario: Let's say that you wanted to utilize the 'pip3' modules that are native and already present on the current machine but ADD to those packages for a given project.
You can instead use the following command, which allows you to add more 'pip3' dependencies for that project by initially piggybacking off the already existing global dependencies and allows you to add in new 'pip3' dependencies JUST for that given project:
python3 -m venv venv --system-site-packages
If you wanted to list the local dependencies in that above scenario, you can utilize the following command:
pip3 list --local
It's interesting that I've gone through the entire gambit of a lot of Linux based installs, both OS-level, and just installing Linux programs (by compiling from source, etc), and something like Python 3's 'venv' virtual environment just makes sense to me. I never used it before because I thought it was useless, but after having to revive my old Python 3 project websites for probably the third time after a site re-design, I realized that its the best way going forward to deploy Python 3 apps.
On a separate note, I found a pretty cool "public access" UNIX system that allows you to access remote shells, and shared logins. Very cool concept, might play with this sometime this week:
Here's to learning from your mistakes!
~Sam
October 20, 2020
I've been doing more and more art in Grafx2.
It's one of those positive things to do despite any frustrations in life.
Any time doing art is time well spent. I don't care what anyone says on that front, since if they say its a waste of time, then they probably don't have any useful talent either.
What I've always like about art is that I can easily see the progression, as well as the risks I took. I definitely hit a block mid-year this year where I didn't really do much art or music. I did some more self-analysis recently, and realized that I still had fun with it, even if the quality of said art was getting good or bad.
I would love to do some demo scene type art, but realize that I still need to keep building the fundamentals. I've been playing around with the ideas of proportions from the Loomis book. However, his eye for perspective is almost too freakishly good to the point where you either quit, or at least try to imitate it.
Perspective is definitely a weakness of mine, even with the reference "Perspective Made Easy" book I readily have available to me.
I can think YEARS back in time when I still thought it was such a weird concept anyway. It sort of almost ruins the creation process a bit, but as you get older, you realize that it's really important in order to scale things up appropriately.
I've been writing more songs for another album for a band I'm going to call JeeveSobs, which is more or less just another midwest emo band.
However, this time, I don't want to suffer from poor audio mixing quality, and I really really REALLY want to make sure it sounds good this time around. Though you can tell I became more aware of how a mix should be over the years through my music, I really never gave the thought to just hand that sort of thing to someone else instead.
I've only trusted maybe a handful of people who even know the vibe of the kind of music I make, but even then, its still hard to find anyone who won't charge a lot just to mix a few songs, so we'll see if I actually get the album mixed by anyone in particular.
I don't think I have a gift for mixing, and I think that's the best thing you CAN admit in order to improve. I'll have to think of the best options around this but I'm glad to say this as I critique my own work alot.
I even sat back and thought about this website, and though I am happy with the progress, I could easily just turn it into a demo scene art page or something and even be happier.
I have learned a lot in terms of what "scope creep" is, and to just keep your ideas to a bare minimum.
I'm at a point of my life where if I don't see progress on something within like 2 weeks or so, I easily lose interest. I just don't want another repeat of that "ExpressOrLocalApp" project where I spent several months on a project to ultimately not really see the effort being actually used. Let's all take a laugh at how I made an MTA data app that is basically now useless. It still is kinda funny.
But yeah, in all seriousness, that's why I stick to small web apps these days.
Keep on working at it! ~ Sam
October 19, 2020
I re-organized the site a bit more, and got rid of the "Radio" section as I really only had web apps on that page anyway.
Though I think Ham Radio is pretty cool, I'm still not that versed or well into the hobby yet to show off any cool projects, so I think its a bit of a waste to have a section on that topic anyway.
With that in mind, I re-organized all those web apps I've made just on the "Programming" site itself:
I'm checking out how to make a literal Emacs config from an Org Mode document to help simplify things a bit, should be fun!
~ Sam
October 17, 2020
Today was a great day.
I finally did it.
I finally installed Gentoo in a VM.
It took three separate attempts over two weekend sessions but I got it booted thankfully. I plan on figuring out how to maybe get Emacs Window Manager present in that particular VM as well, so that's gonna be a cool project indeed. Otherwise, my related installation guide is now pretty complete, so I could easily do it again in less than an hour if I needed to.
I also got my old-as-heck ExpressOrLocalApp project back up and running, which is semi-hilarious because I doubt anyone actualy still uses the 7 Train in NYC anymore. But hey, its updated every minute for anyone who wants this data. I had to re-adjust a few things as the MTA changed their data feed to be more API key specific, so it was a bit of a pain of trying to read their recent post in the related MTA Data Google Group post on the API Key change.
However, I had enough ingenuity to figure out how to change the "headers" section for the requests.get() function call, and baby, we are LIVE again! :D
I've been trying to wrap my head around placing my Emacs configuration file into an Org Mode document since I found out about "Hydra" and "Helm" within Emacs, and just felt the need to figure out how to finally configure Emacs since I've been using it for quite some time now.
Some really good references on this topic include these links:
- https://harryrschwartz.com/2016/02/15/switching-to-a-literate-emacs-configuration
- https://writequit.org/org/settings.html
- http://endlessparentheses.com/init-org-Without-org-mode.html
- https://emacs.stackexchange.com/questions/3143/can-i-use-org-mode-to-structure-my-emacs-or-other-el-configuration-file
Also, in this same burst of research, I found another guy just like myself who literally blogs on his site using Emacs Org Mode in one giant org doc:
I also found this really cool Linux reference link page from a guy on IRC, which is neat and very organized:
Also, through that same guy's site, I found other really really useful Linux reference links for Linux based audio programs as well:
I found this really useful comprehensive list site on Window Managers as well:
This part of his page lists window managers based on the programming languge they're based in, which is super helpful in deciding what window manager to use, since if you can better understand the config file, you can easily modify yourself:
This same guy's list of helpful Linux utilities is awesome too:
On a total side note, I found these pretty hilarious Geocities looking sites, which I might copy in terms of style when I re-design the site later this year:
Keep having fun :)
~ Sam
October 16, 2020
This week has been a bit long in many ways, so I was looking for more ways to get back into doing digital art this weekend to just relax.
I still have that Loomis art book laying around so I might read that.
I found these two goldmines for art learning, both of which have a ton of related links:
I didn't even know people replace drawing tablet nibs with weed wacker cable snippets that are sanded afterwards. This thought alone blew my mind as I just have a cheapo Huion tablet I bought a few years ago which works just fine on Linux with Krita and Grafx2. I think the current digital pen's nib is pretty used at this point, so I definitely am debating how to go about doing this. Here's the specific 0.65" trimmer line that you can use to create your own DIY replacement nibs for digital drawing pens:
I watched so many Adrian Belew based videos this week, so I've been pretty inspired to just keep doing music on the side. The guy's a genius in my opinion. Some of the old King Crimson 80's stuff is just mindblowing, especially their 80's tour videos with the weird looping segments between songs. Such cool stuff. Clips like that always make me want to get a Digitech JamMan Stereo from eBay to do some cool music loops.
Here's some King Crimson 80's vids at their peak, just great stuff:
- https://www.youtube.com/watch?v=-cNwf-lifIw&t=2030s
- https://www.youtube.com/watch?v=JFp95fr9A6w&t=217s
- https://www.youtube.com/watch?v=m1Eavhxlqd8&t=130s
I plan on possibly taking down my Bandcamp and Soundcloud pages, and just re-hosting it on this site. However, I will have to find a nice streamable media player that runs on a page. If that exists (which it probably does), then I'll be set, and depend on one less platform, which is always a good thing.
I might also however have a Donate button at the top of the related music in case the bandwidth caps out though ;).
I found some good ways to self-host audio streaming on my own site:
- http://opentape.fm/
- http://www.schillmania.com/projects/soundmanager2/
- https://nunzioweb.com/streaming_audio-example.htm
On that "nunzioweb.com" site, I noticed how on this page, he was able to change the CSS stylesheet on the fly, which is EXACTLY what I wanted to do. Time to somehow copy his "setActiveStylesheet()" JS function ^_^
After careful examination of that page's "Network" tab, I believe I found the source of the magical JS script that changes the stylesheet on the fly. I definitely am debating how I can adapt this to my site, very cool:
Other than that, I'm doing some cleanup on the "Programming" page and am thinking more about the small React JS app I want to do to pull down Yahoo! Finance stock data. I've been trying to get some of the older projects running again, and realized that a lot of them would have been better suited towards running them using 'venv' in a virtual environment to prevent having to pull in Python 3 library dependencies for cron jobs.
Maybe I'll look into running a BBS or hosting an IRC server on this site, who knows.
Just gonna chill out as far as I know though :).
Hope everyone is doing well.
~ Sam
October 14, 2020
I've been looking up more Hungarian food recipes to make this year.
Here's a great YouTube channel to learn how to make some Hungarian food, as the recipes use both metric and Imperial measurements:
Here are two great YouTube channels on Hungarian cooking, but be warned, they're both in Hungarian without any subtitles or captions, so I would recommend this only if you can understand Hungarian (like me):
- https://www.youtube.com/channel/UCRxaYgjGaaxeQwmMwxQ2pGQ
- https://www.youtube.com/channel/UCwkOFhV3Zj76FArHCQRQTzg
I'm gonna chill out and check out some more Demo Scene art to see if I can get ideas of what to make myself one of these weekends for fun.
I am also thinking about what to add to my Emacs config between all my machines.
I would like it so that the modeline at the bottom has more auto-completion. Also, I would like to try making a few Hydra key combinations to make things a bit easier. I might even learn some more Org Mode as well, who knows.
Still planning on finishing the Gentoo install this weekend, and am looking forward to it :)
~ Sam
October 13, 2020
I began work on an AngularJS app to record callsigns for Ham Radio:
I plan on making it AngularJS based, and want an easy way to quickly record callsigns and look them up on a related callsign lookup site.
I'm still debating the usefulness of it, as it would be cool to export the same data. However at the same time, instead of exporting it to Excel or .txt formats, you could have easily made a similar list in your text editor of choice, so why bother exporting it?
However, that's where we can abuse the fact that its a web app in a browser since we can then create anchor links to easily get information about a person given their callsign for future contacts (email address, etc.).
Here's the link for AngularJS for reference:
I wasn't able to easily get a ham radio callsign lookup using the popular ham radio site, QRZ.com, or even the FCC database. This is most likely because both sites most likely use AJAX calls to refresh the page without refreshing the overall page. This means that the URL itself is never updated with your actual search query so you can't just build anchor links unfortunately. To see this in action, use your favorite search engine, and notice the "q=" variable for your search query change within the top URL as you progress throughout the site.
Also, I know for a fact that QRZ.com uses an API, which means that even if I were to apply for an API key even for a basic app, there's still the chance that someone might abuse my given API key with 60+ API calls per second. Plus, I hate API's so… that's that.
It's always easier to just scrape data instead ^_^
However, I did find this jenky third party website that allows you to easily lookup ham's by their callsign without an API , so I'm just going to use that instead:
It's gonna be fun :)
After that, I'm planning to make a React JS web app for a stock ticker to rip the top stocks from the the Yahoo! Finance stock tickers directly, or through a Python 3 webscraper data pull.
I'm going to most likely have to re-arrange the "Programming" page to accommodate this, but I can easily have a "web app" section near the top, and any of the archive or Git repo related projects at the bottom, so it shouldn't be too hard.
I also have been browsing for some art to follow, and really trying to see what's out there to push myself in what I am to be able to do.
Since its pretty obvious I'm into a lot of technical topics, there's a lot of art that translates from the "Demo Scene" that relates to my interests, and the art I want to do. The "Demo Scene" is a bit hard to explain, but it basically features computer based art for the most part. It also involves gatherings similar to LAN parties, but with mostly demos including computers, music, and art.
Here's some "Demo Scene" art website I found to be pretty cool:
- http://artcity.bitfellas.org/index.php
- http://www.bitfellas.org/e107_plugins/radio/radio.php
- http://janeway.exotica.org.uk/search.php?show=100&query=&what=0
- http://artcity.bitfellas.org/index.php?a=latestpictures
More so, if I could do Grafx2 style art in the vein of this guy, I would be pretty happy with myself:
Unfortunately, that "ProwlerGFX" guy is primarily on Facebook, which I don't use at all, and his website is pretty non-functional at this point.
But, I will find similar artists in this style, and see how they were able to get to this level as I really really really like scene based art a lot.
I believe I will get there to the art based apex I want. It's just that I have to keep practicing art on the weekends whenever I want to chill out. It's hard sometimes because I burn out from the week easily, but I'll figure it out somehow.
I've made a few icons in Grafx2 for fun the other day, so that's a start haha.
Also, I would like to start recording for a new band this weekend so that will be fun too.
Happy to keep on going!
~ Sam
October 12, 2020
Still working on the "Space Weather" JQuery UI app.
I got the actual K-Index and Sunspot data to display on the page itself, so that's pretty cool. I also got the "K Index" graph to also display by using appropriate "src" attribute for the related img tag. Only thing I have to modify is to include a setTimeout() statement to have it continously looping. Otherwise, its pretty much done:
Now to think of what to do for a simple Angular JS app next :)
Maybe something with open data feeds, not sure.
Was gonna mess around with the solderless breadboard setup I have to learn a bit of electronics, but I was a bit dumb and forgot to buy a multimeter, as well as a few ranges of resistors as I assumed the starter kit I got came with everything.
Boy, was I wrong.
More so, I'm debating getting a Ardunino Uno copy from Amazon as well since its purpose is primarily for entry level electronics as well, and they're super cheap and run on USB powerbanks, so there's no risk of getting shocked or anything while learning basic circuits.
I'd like to build up basic circuits, and maybe make a few small synths eventually.
Here's to learning,
~ Sam
October 11, 2020
I have been in the process of installing Gentoo. Though it has been fun and I have learned so much throughout the process, the process itself is pretty long to the point where I had to pause the KVM Virtual machine in Virt-Manager to continue the process sometime later today. The IRC channel for Gentoo on Freenode has been SUPER helpful though, and overall, the process has been so much better than trying to do the same thing for Arch or something. Plus they allow you totally ignore 'systemd' which is a backdoor to avoid putting on your system anyway.
Though I haven't found any Librebooted computers worth getting yet, I still want to move in that direction to maybe score a Thinkpad X60 or Thinkpad X200 on eBay so I can libreboot it for that purpose. In the worst scenario, I could even look into building a computer whose motherboard is Libreboot compatible as well.
I was looking into packet radio, and somehow found the "radio.garden" website, which allows you to tune into radio stations throughout the world. It allows you to move a virtual globe to select any nearby radio stations. They've even got ones at "the ends of the earth" including an arctic radio station, which is pretty neat. You can check it out here:
I found this packet radio network where they have created an IP-based digital network for Ham Radio use, but it seems to be primarily in the Washington state area. Though I have no plans at all to move to that part of the U.S., it is pretty neat though. I would do love to run a similar mesh network through the South though, so it's definitely something to model after:
Sometime yesterday, I was doing a TON of research in credit card churning, and how to go about doing it. I think it's a pretty valid thing to do, especially since the economy sucks for anyone like me in my generation that doesn't have any real estate, and is just continuously working week to week to just to pay bills, and get pretty much nothing else in return . Sure, I could blame boomers for basically rigging the real estate market for what it is now to the point where you can't even fathom how to own a reasonably priced house or even land for that matter sometimes without somehow having to convert a fixer-upper property to make it somewhat affordable.
However, the positive way around that would be to just figure out how to play around those stupid barriers, and rig your current financial system for your own benefit.
Though a lot of what you initially read about the credit card churning subject are articles by credit card companies telling you why its so bad, I have found so many different sources contrary to that narrative to the point where its pretty obvious that these same credit card companies don't want you doing it in the first place.
Sure, a lot of the deals are primarily based on travel points for flights, hotel stays, etc, so if you're not really intro travel, it's not THAT great. However, there are still good intro offers for a lot of credit cards to abuse with the minimum spending limit and to just cancel the card before the annual fee is charged, so if you plan it right and don't over do it, its not that bad.
I'm not going to link to any credit card churning sources I found, since its really really subjective, and is kind of YMMV (your mileage may vary), so its not for everyone. All I know is that it would definitely work for me.
That being noted, I'm totally going to check it out as it looks like a good way to gain some extra cash on the side to maybe invest in a few stocks. It's the best I could do before having to becoming some cryptocurrency shill or something. Even people who are into trying to find the next cryptocurrency just irk me because they don't even use the coin itself, and just literally convert it back to dollars. In that respect, the only reason to ever get into trading cryptocurrencies is to abuse it for some kind of get rich quick scheme. You might as well try one of those pyramid scheme type "Poor Dad, Rich Dad" seminars at that point since both efforts would totally result in failure.
I'm glad that I figured out that people are just into cryptocurrency for that reason alone, so its something to just avoid altogether.
In terms of stock tickers, I found a lot of good links for that purpose, which I might turn into a few dashboards on this site for later use with the help of some Python 3 based webscrapers.
A lot of the stock software I found was primarily Windows and Mac based, but I DID happen to find some Linux based software (thankfully :/).
Here's a link for any Linux based financial software:
The "JStock" Linux stock software alone looked pretty promising as well, so I might considering figuring out how to use it as well:
Enjoy the day!
~ Sam
October 9, 2020
I did some slight work on that "Space Weather" app.
I was able to index into the related JSON data from the NOAA Apache Web Server in the JS console, as its just a JSON object with specific key values.
I'm still debating on how I want it to look though, so I'm torn between the JQuery UI's "Tabs" vs "Accordian" look.
I found this cool site that gives homage to old Geocities cities, and is worth looking at, as the guy took assets from older Geocities sites:
An even cooler page is the "Oocities.org" website, which basically archived ALL of the Geocities web pages before it got taken down.
This means that if you somehow remember your Geocities username, you can find your site still active here:
Here's a very nichey Super Mario themed Geocities site I found as well:
The reason I was looking into old Geocities sites is that I want my next re-design of this site to be Geocities themed.
Maybe, just maybe I can find my old Geocities page with all the Pokemon sprites and icons.
That would be the day.
Also, I've been debating if I should move over to a VoIP setup since even though I prefer the fliphone setup I currently have, even not having text would be ok with me too.
Also, they're going to slowly get rid of 3G towers, so sadly I probably would be forced to move over to a 4G phone eventually.
After that, I would DEFINITELY swap to a home phone at that point with an AX-25 enabled ham radio BBS node in the car to match haha.
These VoIP services were hard to find, but here's a list of potential VoIP services for personal use since a good chunk of them are geared towards business use, and I think they're assuming every Zoomer would immediately prefer a cellphone (Side Node: I'm pretty surprised Vonage still exists):
- https://www.vonageforhome.com/personal/phone-plans/vonage-north-america/
- https://www.voipo.com/
- https://www.phonepower.com/usa_canada_calling_plans.aspx
- https://www.ooma.com/home-phone-service/?clickid=0Qk1ek0OBxyLUqCwUx0Mo3YgUkE0AFxJTyVnxk0&om_phone=866-573-1808&irgwc=1&utm_campaign=Ziff%20Davis%2C%20LLC.&utm_source=Affiliate&utm_medium=Impact&Partner_ID=10915&utm_term=524192&utm_adname=Generic%20Page%20Link
- https://www.axvoice.com/
Keep your mind active!
~ Sam
October 8, 2020
I did more thinking about what to deploy on this site.
So far I'm thinking of deploying:
- An RSS Feed Agregator
- Stock Ticker
- Email Server
- Voip Telephone In Browser
- BBS
- Gateway For Echolink, DMR, and Fusion Radio Nodes
- Webpage For Old-School Computer Emulators
- OpenStreetMap Page
- Web fonts repository to store nice fonts (https://github.com/Finesse/web-fonts-repository)
- ytdl-webserver to pre-download YouTube videos
- Office suite software on a webpage to avoid using "Office-365" or "Google" based software
- Pastebin without syntax highlighting
- VPN service
- Search engine (Searx, etc)
- URL shortener client
- Image gallery for my artwork
- tube, a YouTube-like alternative (https://prologic.github.io/tube/)
- homer, a server app front end page (https://github.com/bastienwirtz/homer)
- Apache Guacamole to host VNC's to allow people to access virtual machines online (http://guacamole.apache.org/)
- "Minetest" server (https://content.minetest.net/packages/?type=game)
- Net64 server for multiplayer N64 games (https://net64-mod.github.io/)
- Webpage based IRC client like Kiwi IRC (https://kiwiirc.com/)
- Hawkpost to share encrypted files (https://hawkpost.co/)
- Archivebox to backup bookmarks and other sites (https://archivebox.io/)
- QuakeJS server
- GNU FM instance (https://gnu.io/fm/)
Cool links for other data projects:
- https://github.com/onurakpolat/awesome-bigdata
- https://github.com/awesomedata/awesome-public-datasets
Here is a list of some SysAdmin based apps to deploy as well:
Here is a link to some privacy respecting Linux software:
Here is a page full of alternative internet and privacy respecting software:
Here are libre software projects (free as in freedom):
Here is an Internet alternative I found called "Freenet":
Clearly… there are several options :)
And a lot of choices to be made ^_^
I'll keep an "Apps" page to host all these ideas pumped up by JS.
Otherwise, my main page will become a Geocities type hub spot sort of page.
Keep having fun,
~ Sam
October 7, 2020
GitHub is pretty much trash these days, but here is one cool thing I found from it:
Basically that link is a ton of apps and resources that you self-host yourself on sites like this.
That means, MORE IDEAS FOR APPS TO DEPLOY ON THIS SITE :)
I was already kind of just set on deploying Unrealircd to create a IRC server to connect to via SSH key or password or something, but I'm glad to find more ideas of things to deploy :D.
The only other thing I could think of was to maybe to create a email server, and maybe even a public pastebin to link to code (without syntax of course to prevent SQL injection attacks, or cross-site scripting via PHP scripts).
I still shudder when I think of when this site was hosted on a public hosted server, and the dang PHP infested portion that appeared out of nowhere which was a result of some hacker in India. Worst part about is that the shared hosting provider, InMotion Hosting didn't even care, despite my multiple emails regarding the issue.
Let it be a lesson to anyone to NEVER pay for shared hosting. Always host your website yourself on a private VPS instance using a VPS service provider like Vultur, Digital Ocean, etc. You can be even more based by hosting it at your own home using a single board computer (Raspberry Pi, etc) but you would have to figure out a safe way to do port forwarding as well to not allow the entire internet to access your home network though. With this in mind, make sure to do your research first before doing this option.
I've been working on hashing out ideas for that Solar Weather JQuery UI app as I've had to learn more about what actual practical applications for showing solar weather regarding Ham Radio's HF (high frequency) propagation.
The coolest thing I learned today was that after a solar flare, the ionosphere will be loaded with more ions which BOOSTS your HF propagation to allow for a much greater distance for contacts.
Now if I could just afford to buy a DMR radio (MD-UV-380), we would be set…
And maybe then I could finally make a DX contact with Hungary :/
I have a few fun things planned for the weekend to relax, so I'm staying positive as I can.
Been going through Book of Samuel (KJV) still between tickets at work sometimes when I need a boost.
God Bless :)
~ Sam
October 5, 2020
I've been debating what I want to do in terms of a JQuery UI style App.
I'm leaning towards using the JQuery UI "Accordian" widget to make some kind of expandable web app.
I found two cool NOAA based directories to either obtain JSON and image data from:
Here's the overarching site if you're interested in other forms of data from the NOAA server, which is neat since you don't have to apply for an API key in order to utilize their data:
Related progress on the "Space Weather" web app can be found here:
Also, a LOT of ideas can be gleaned in terms of space based weather for use in Ham Radio on this site I found from QRZ, which is CONSTANTLY being linked on personal ham radio enthusiast pages:
I've also been playing around with some ROM hacking via some related utilities from this site:
One ROM hacking utility that worked right from the get-go using WINE in Linux was "YY-CHR" which allows you to open up a related ROM file, and just edit sprites right away, which was super cool:
Now what kind of ROM hack I would make… that I'm not sure. But I'll admit, it is super cool how easy it is to just open up a game's sprites and just have at it so I'll come up with something cool I guess.
Way easier than attempting to make a game in some constantly shilled engine like Unity or something.
Probably will make a ROM hack for Gameboy Color ;)
I've been debating what separate piano songs to learn, so I've taken a look at some video game based sheet music:
Also, I got Qemu to load up on the Linux Desktop, so I'm planning on installing Gentoo in a VM this weekend when I get a chance.
Looking forward to learning some more hands-on Linux skills, and finally taking the Gentoo challenge.
Keep having fun :)
~ Sam
October 4, 2020
I got my Jitsi instance running.
However, it's only usable using a Chromium variant browser. Because I despise Google, I've had to resort to using Ungoogled Chromium which works flawlessly for Jitsi thankfully.
There are a TON of Firefox issues for Jitsi-Meet, so I don't feel bad at all why Firefox doesn't work, since its a known issue, and there's no known workaround:
- https://community.jitsi.org/t/software-unusable-on-firefox-why/22143
- https://github.com/jitsi/jitsi-meet/issues/4758
- https://bugzilla.mozilla.org/show_bug.cgi?id=1468700
- https://github.com/jitsi/jitsi-meet/issues/2835
I basically followed this blog post to deploy a Jitsi instance on this site for anyone wanting to do it on their Debian VPS server:
Also, if you ever need to troubleshoot the related technology, aka "WebRTC", there's a test site to test your webcam and mic setup:
I also got my Desktop machine back and running with its video card plugged in, so now I'm just trying to move my dotfiles back on the machine before proceeding with using 'qemu' to install Gentoo in a VM.
The command that I used to obtain the dotfiles on the Desktop machine is:
git clone git@git.musimatic.net:/var/www/git/dotfiles.git
Also, apparently, you can shorten the URL itself by adding this to the "~/.ssh/config" as well:
Host git
HostName git.musimatic.net
User git
Now if I can only get GNU Stow to work to just stow away my dotfiles properly…
October 3, 2020
I've been trying to get the Searx instance running, but it appears that the "filtron" component is the part that is doing excessive rate-limiting for some reason.
I'll have to lurk on their IRC channel to maybe get some feedback of what to do.
Speaking of IRC, I found a couple of links that are interesting and useful as well to find IRC networks OTHER than Freenode:
Been also debating the best ham radio setup as well, since I might just get one of those meme-y type 40 inch antennas for my HT (Baofeng UV-5R) to improve my signal strength to hit a few more repeaters for like $20.
We'll see.
Keep having a good time :)
~ Sam
October 2, 2020
Learned a little more about APIs from the JS book I have.
Debating what kind of web app I should create, either with JQuery UI, or Angular, just for the experience, and also debating the "why" aspect of it.
Also debating what else to deploy on this site, will most likely be one of the following ideas:
- Unreallircd Server
- Mumble Server
- Jitsi Instance
- Searx Instance
- BBS
I've been playing around with KiwiSDR, which is another "Software Defined Radio" type website where people basically allow you to play around with their already configured shortwave radio via a web page.
Here's a few helpful links regarding KiwiSDR:
List of public instances of KiwiSDR:
Map of existing public instances of KiwiSDR:
Signal-to-noise ratio score based site that ranks KiwiSDR instances in terms of how loud their signal is:
And hey, there are a BUNCH of Hungarian KiwiSDR's present too, here are a few:
- http://ha6smfkiwi.proxy.kiwisdr.com:8073/
- http://kiwiradio.proxy.kiwisdr.com:8073/
- http://fsdr.duckdns.org/
- http://hg5acz.ddns.net:8073/
Have fun listening :)
~ Sam
October 1, 2020
I changed the "favicon" for my site to be a cool neat lightning bolt I customized myself, which you'll see at the top of each tab.
The fact that it had the old stupid "InMotion" favicon bothered the heck out of me recently, so I decided it was time to get rid of it once and for all.
I originally tried using this site to create the favicon using some example Pokemon sprites:
However, I realized this site was a little bit easier to use, allowed you to edit it on the fly, and even allowed you to export it:
I'm pretty happy with the results, as icon work brings back such good feelings, and remind me of fun Geocities pages, especially my old one.
Now if I could just find my old email and dig up my Geocities name and find the associated site, and bring it back to life!
Now THAT would be something!
Keep enjoying life :)
~Sam
September 30, 2020
After a lot of effort during lunch breaks at work, I was able to finally fix the issues with the "ISS Location Receiver" project on this page:
I have to thank this blog post since it basically did the same idea, but their project is a little bit more refined and polished:
I found a Budapest, Hungary based SDR to check out for anyone interested in Hungarian Ham Radio:
I've been trying multiple Hungarian based Echolink repeaters, but can't seem to get a QSO with any Hungarian Ham Radio Operators yet unfortunately.
It must be a timezone thing, or maybe Echolink just isn't as popular in Europe.
I've been debating getting a DMR based radio with a PiStar node (either my existing Raspberry Pi 3, or get a Raspberry Pi Zero later).
The idea is that you can hook up a DMR radio to a Pi-Star hotspot, and then connect with radio towers across the world, much like Echolink, but with an actual radio instead of a headset, which is pretty dang neat.
I can't say I have the $200 or so to even do this setup right now unfortunately due to just making enough right now to pay the bills, however, it something to consider down the line.
I bought a breadboard for electronics based stuff, but forgot to get a related battery for it, GO FIGURE ha!
Also, I've checked out a few BBS's by using the 'telnet' command, super cool stuff:
Anyway, keeping my mind occupied with the site, and happy I still have it. I'll keep thinking of cool sections to add, and also the grand ol' re-design I've done every year. This year, I plan on doing a Geocities themed makeover, so it's gonna be rad.
I found out how to use 'mpv' to display videos in a terminal using the following command: mpv –vo=caca (name of video)
It'll look like something similar to this, but you can least view videos on lower-spec computers in terminal without a problem: https://www.youtube.com/watch?v=2C7tGsTA5Og
Makes me want to start a "TTY" or "X-less" based blog where I just PURELY use terminal based programs, and don't rarely boot into X. Now THAT sounds like fun ^_^.
Keep having fun :)
~ Sam
September 25, 2020
I found out about "SDR", which is Software Defined Radio through someone who talked about this on IRC.
This is better explained on the SDR website found here:
Also, there are other ways to listen to Ham Radio online, so check out this link:
Just give the SDR's below a shot by loading one of the links below in a modern GUI based web browser, and play around with the tuner (yellow icon) to change the frequency. You can also change the "band" to change the different wavelength bands as well. Also, you can listen to basic FM/AM radio too if you're into that sort of thing.
Here's the list of web-based SDR's I found:
Washington DC Area Based Web SDR:
Northern Utah Area Based Web SDR:
Milford, PA Based Web SDR:
Currently debating between two separate radios, both of which can be programmed easily via CHIRP:
- Anytone AT-778
- Anytone 5888
I've also been looking into how to properly connect to BBS's via this link: https://www.telnetbbsguide.com/
Keep having fun :)
~ Sam
September 24, 2020
I've been working on utilizing a few links to get the "ISS Location Receiver" project underway, which can be observed in the "ISS Station Location" section on this page:
I've been utilizing the following links to do so:
- http://open-notify.org/Open-Notify-API/
- https://leafletjs.com/examples/quick-start/
- https://leafletjs.com/reference-1.7.1.html#map-options
- https://asmaloney.com/2014/01/code/creating-an-interactive-map-with-leaflet-and-openstreetmap/
- https://wiki.openstreetmap.org/wiki/Slippy_map_tilenames#Tile_servers
- https://openmaptiles.org/
- https://mledoze.github.io/countries/
Still deciding on how to best do this as you can easily just have it run every five seconds with a "setTimeout()" JS function.
Also, I would like to do this via an AJAX request via a button too if possible which would be cool.
We'll see.
Otherwise, still looking for a $200 mid-tier Ham Radio since I'll be able to get a free J-Pole antenna and power supply from two different Ham's in the area. Looking for one that can be easily programmed with CHIRP software, but can reach repeaters in the area.
I think I'll keep myself occupied with Echolink via QTel for the time being though while I keep doing my related research.
Also, I'm looking into VoIP based services to make internet calls with cordless or corded old-school phones, since I pretty much am fed up with using cellphones these days.
Some VoIP software I found that works on Linux:
- https://jitsi.org/downloads/
- https://github.com/mumble-voip/mumble
- https://qtox.github.io/
- https://jami.net/download-jami-linux/
Keep staying positive :)
~ Sam
September 23, 2020
I did a lot of backend website modifications, including re-ordering of my Git repos for my projects.
Though I haven't gotten all of the older projects up and running, one particular new project I have up is the ISS Location Receiver based script that pulls in the longitude, latitude, and Unix timestamp of when the ISS Space Station is overhead.
This means that you can monitor it going over your house over the course of the day.
For now, you can pipe in these longitude, and latitude coordinates into a search engine to figure out where it currently is.
You can find out more about the "ISS-Location-Now" API through the documentation here: http://open-notify.org/Open-Notify-API/ISS-Location-Now/
I'm planning on somehow incorporating this with "Leaflet" to provide a real-time map in a webpage as well to keep it relevant: https://leafletjs.com/
Anyway, you have to celebrate the small wins, as this helped me learn how to save a JSON request file ("iss_location.json") locally, and each time you press the "Click Here To Display Current ISS Space Station", an AJAX request is performed so only that portion of the page is refreshed.
This means that it doesn't have to reload the page in order to display JUST that information since it pulls all of the information from the JSON file that I obtain every minute via the Python 3 based requests module.
Keep having fun,
Sam
September 20, 2020
I've been getting more into Ham Radio recently, and it has been super cool.
The license exam itself is kind of useless for someone like me that is using a low-tier handheld radio, as the questions are geared towards older enthusiasts who have amples amounts of money to build radio towers on their property, with a $500 to $1000 radio to match.
However, once you kind of get past the somewhat useless exam which encompasses a good majority of high school level physics, its not too bad.
Would recommend "hamstudy.org" for anyone interested as it has the SAME exact question pool as what's on the test, and it is what I used to study for the exam: https://hamstudy.org/
The possibilities of using packet radio, BBS's, and Echolink (via QTel) has been really fun to play with. So far, I've received a ton of support from Ham's in the Echolink based net calls, since the Ham's near me don't really do much packet radio. Most people online kind of dismiss packet radio as a "fad from the 90's". I, however, see it differently as the potential of using a mesh network to connect devices such as computers across the world without having a centralized ISP dictating everything is MIND BLOWING.
Of course, a lot of these kind of mesh networks are limited to Hams, but hey, just get the basic Technician level license, and you're set.
I've expanded to the world of VoIP over Echolink, which allows you to basically to anyone throughout the world that has a Ham radio license via the internet. It might sound like Skype to some people, but is way less proprietary or invasive privacy-wise. Though you might be thinking, "Yeah, isn't that kind of dangerous?", I honestly have not heard nothing but positive people that are super encouraging. The ham radio hobby attracts very tech minded individuals, so its not anything weird like in a strange internet chat room or something since its FCC regulated.
Also, you have to keep in mind that although you are accessing these radio towers via the internet, a lot of them are PHYSICAL radio towers which are repeating your same radio signal throughout a PHYSICAL region. With this in mind, people aren't as open as you would think, and are pretty polite with each other as a result. Plus, most of the topics discussed are pretty much centered around the hobby itself. Honestly, a good chunk of the people I spoke to so far are just retired individuals that are looking for positive conversations throughout a given day. I've heard it all from what people are doing at a given moment, such as errands, fishing, etc, to just talking for minutes about a given topic, which is called "rag chewing" in Ham lingo.
Echolink can be found here, but NOTE: You NEED to have a callsign registered with the FCC in order to use it, so you CANNOT use it unless you are a licensed Ham: http://echolink.org/
It's primarily aimed for Windows users, but you can also utilize "QTel" if you have a Debian based Linux computer via your "apt" package manager: https://pkgs.org/download/qtel
I learned that there are "net calls" which are basically group calls that occur once every week on a given topic. I was pretty surprised that there are several net calls around very specific medical based ailments on certain frequency bands, so that was something I never knew people actually did with ham radio.
For example, one guy was going on about how there's a stomach based net for ham's who suffer from stomach ailments, so its kind of like mini-support groups for given topics. However, though I mentioned medical based topics, net calls span a whole range of topics. For example, I listened into a Raspberry Pi based net call, and learned SO MUCH more about Linux with radio than even on IRC. I absolutely will tune in next week at the same time slot, since I want to learn more and more to expand my base knowledge on the topic.
In terms of this website, I've taken care of a few minor things, including:
- Revised my GitWeb site to include a dark theme CSS stylesheet:
- I've been able to make two of my existing project sites work again after the
recent NGINX backend upgrade: https://musimatic.net/pythonprojectwebsites/Bandcamper/tags.html https://musimatic.net/pythonprojectwebsites/ScriptureOfTheDay/output.html
The remaining goal would be to maybe get some of these other project websites running.
However, projects like my "ExpressOrLocalApp", though cool when I first made it, wouldn't benefit anyone since everyone's leaving NYC nowadays anyway, so it might not even be worth it to get that specific project website working again.
The cooler thing would be to start running a BBS or something, and roll with it. That and run a "Searx" instance, run a "UnrealIRC" instance, etc.
Or maybe, even get the page looking like an old Geocities or Angelfire site like this for example (without the dreary gothic theme, but you get the point): https://gothicnight.com/login.html
I've been working on getting the "ISS Location" page running, so that's been fun, so far I've been working on making sure the JSON request saves properly so that I can run an AJAX request with the push of a <button> tag on the page to pull the latest JSON response that's downloaded to the same directory via a crontab job: https://musimatic.net/radio.html
I've been toying with the idea of possibly creating a "Lode Runner" game but based on someone else's game, change out the sprite sheet, and have it running on a JavaScript based engine so I can run it in browser on a page, and across multiple devices.
That alone would be pretty cool imo.
You can play Lode Runner for free on Internet Archive (archive.org) on multiple old-school computer emulator platforms IN BROWSER: Mac Version: https://archive.org/details/mac_Lode_Runner Apple II Version: https://archive.org/details/a2_Lode_Runner_1983_Broderbund_m_mod_keyset_Nut_Cracker_Ace_Mechanic DOS Version (Sequel): https://archive.org/details/LodeRunnerTheLegendReturns
I've also been trying to get Retropie working to use Amiga, Macintosh, and DOS based emulators.
So far, I've been able to boot Retropie without a problem. However, if I try to run any Amiga games, even with the necessary "kick" (BIOS) roms present in the proper directory, it boots into a black screen.
However, it'll be pretty dang sweet when I get some cool Amiga, Mac, and DOS software running on the old Raspberry Pi 3 I have, since its been a while since I used it. I really do like the Retropie menu a lot. I think the last project I tried doing with that box was "DietPi" which was ok, but I'd rather get some kind of old desktop computer, put a super lightweight Linux distro on it, and run it as a Desktop computer with just a TTY prompt without running
Now THAT would be a cool challenge.
I've been trying to find a cool old broken Macintosh from the 80's to house my Raspberry Pi 3 in, but I can't find a good deal for one on eBay or locally since every older dude knows EXACTLY what they're selling, regardless if its broken or not. The idea would be to just remove the guts of the old PC, fit in a new monitor in the front panel of the Mac, and get a glorious BBS running or something.
THIS is what I would love to do, but don't really have the kind of eBay money to do so: https://www.instructables.com/id/Making-ApplePi-Merging-a-Vintage-Macintosh-Plus-Wi/
Looking forward to possibly installing Gentoo in a VM, but I STILL am unpacking boxes from the move (go figure), so I would need to find the screws for the video card so I can remount it to the Desktop machine I have.
Staying positive, and still doing my thing (which is, having fun :D).
Stay well,
Sam
August 30, 2020
I changed the CSS of this page to be a tad bit simpler as the solarized theme for the CSS was a bit much.
Pretty happy to get back to the whole dark blue purple theme, so I'm pretty set.
Might add some small tweaks with fonts, but the simpler the better as I plan on overhauling the theme to like a Geocities-type old school theme at the end of the year as well.
I was able to find my Desktop computer in my moving boxes with the power cable, but need to dig in other boxes for the screws for the video card, as well as the TV I've been using forever as the monitor for it.
That being noted, I'll probably get to trying to install Gentoo in a VM another time in that case.
I've been trying to schedule a remote-based Technician level ham radio license exam. The earliest I was able to schedule it is in the second week of October, which slightly sucks, but I might be able to schedule it sooner with one of the other two remote based ham radio clubs that are running the exams remotely.
I've been pretty much consistently passing the practice exams online, so it shouldn't be too bad.
However, the requirements of most of the remote exams minus ONE of them include the requirement to use Zoom, which sucks since its basically glorified spyware, but I'll just smack it on my work computer so I don't have to risk a test procter getting mad about me running it in a VM or something since a lot of the remote tests are kind of vague about using VM's to run Zoom.
The main formulas to remember for the Technician-level Ham Radio License Exam include:
- E = I * R
OR with its units: V = A * O
This would be Energy (E) = Current (I) * Resistance (R)
Also, the second portion regarding the involved units include: V = Volts, A = Amps, O = Ohms
- P = I * E
OR with its units: W = A * V
This would be Power (P) = Current (I) * Energy (E)
Also, the second portion regarding the involved units include: W = Watts, A = Amps, V = Volts
Everything else, despite what anyone else says online, is basically just memorization of answers, since someone like me is only planning to utilize the junky Chinese type handheld radios like the Baofeng UV-5R to make contacts locally, and even if I DO like the whole hobby, I'll probably only get a CB type radio at best for like around $200. I kind of laugh when I see these boomer type prices on eBay for like OK looking ham radios for like $500+, so I'm only skimming the surface of the hobby for fun, and not planning to invest too much into it.
In terms of Emacs Org Mode, I learned that if you want to change an ordered list item to have a different index, you would use: (number of item) '[@(new index number)]'
For example:
- Cool Thing To Do
- Second Cool Thing To Do
Could Become:
- Cool Thing To Do
- Third Cool Thing To Do
I've been taking it easy otherwise as there's still a few errands that need to be done after settling into the new place over the course of the month.
I'm looking forward to cooking a lot of Hungarian and Vietnamese food to keep my mind occupied despite the times, since the days and weeks are pretty much blending at this point, which sucks on a personal level, but there's not much else that can be done.
I'm definitely still thinking of useful things I can add to this site as well, including mini sub-pages would benefit people like myself who are getting interested in ham radio, as well as Linux based info.
Stay thankful, and happy for the things you have today.
~ Sam
August 23, 2020
I did a very big move recently, and we just got all of our stuff settled into the new place.
It's been great so far, and pretty laid back comparatively.
However, its been a lot of ups and downs regarding how to rent a house, so I can't say its been too easy, but I've been grateful that we made it in one piece and are closer to family given these crazy times.
After a few weeks of just chilling out after work so I don't burn out, I realized my efforts in terms of learning any tech stuff on the side should be focused on what I can apply directly into actual projects.
For example, instead of doing the related JS book from start to finish, I think I just need to read through a related chapter, see what's applicable, and make a part of this actual site that features that capability instead of working on silly fake websites that would be used as part of some "portfolio" or something.
Even as far as portfolio websites are concerned, I'm pretty sure if you can deploy a Wordpress site, you're pretty much close to doing anything similar, so I might actually devote some time deploying some small tinkering Wordpress (WP) sites in that case to gain that skill.
As far as the work based ElectronJS app I've been working on, I plan on just following some YouTube tutorials, see what sticks, and just apply it to the app itself as its not rocket science.
I've been also working on getting my ham radio license, and figuring out what I'd like to do with the yard gardening wise since I do have a garage to get some projects done now.
Also, I'm posting some useful Emacs based Org Mode shortcuts for anyone who does finances like myself in Org Mode as well:
C-x-h: Select everything on screen
C-c-|: Turn the entire Org document into an Org-Mode table
C-c-}: Turn on row and column numbers to make formulas easier in Org-Mode
M-S-Left: Delete a column
C-c-^: Sort a column based on the provided choices (Note: Do this in the Date column based cell for an easy date sort)
Other plans for this site:
Idea | Description |
Deploy Searx Instance | Search engine without the ad tracking, and to be used among people I know / better alternative to Google or DuckDuckGo |
Useful Ham Radio Utility Webpages | Maybe could scrape some useful frequencies, NOAA weather, etc |
Radio (FM, AM, etc) | Could be a fun little side page |
Change the CSS stylesheet for the "Blog" page | Solarized theme is kinda looking OK at best, could be better |
Deploy UnrealIRCd IRC Server | Would be a great private IRC instance for family and friend use |
Staying positive, God Bless.
August 2, 2020
I've been in the process of trying to get "BasiliskII" emulator to work, so that I can run some cool old Mac programs for fun. I was thinking to maybe setup my old Raspberry Pi 3 to boot into BasiliskII to run MacOS7 or MacOS8 to possibly do some cool audio recording ideas, and play some old games / use older Mac software.
This link looks promising as a good guide, but we'll see:
For the time being, I've been checking out Internet Archive's collections for fun.
Archive.org's Mac Software Collection:
Apple II Based Software Collection:
August 1, 2020
I have been in the process of trying to deploy a 'Searx' instance on this site, but so far, I haven't made much progress and am kind of stuck on why its always stating "Rate limit exceeded" when you attempt to visit the Searx instance via the 'curl' Linux terminal command.
I've been trying to utilize this part of the Searx official documentation guide and a related GitHub issue that has a very close nginx based configuration for the 'searx' site but haven't really figured out the root cause of the issue as I've followed each of the installation steps VERY closely:
- https://asciimoo.github.io/searx/admin/installation-nginx.html
- https://github.com/dalf/searx-instances/issues/68#issuecomment-659293546
Only other thing I noted this weekend is that "invidio.us" looks like its going to taken down in September 2020, which sucks because I try to access YouTube through that instance to pull down videos for later viewing so that I don't get a million tracked ads while on YouTube.
It is 10x better to watch content locally on your computer than having to stream and potentially deal with ads and the like. Also, watching videos locally with 'mpv' is amazing. I just hope someone forks the "Invidio" project here, and keeps it going so that I can keep checking out content on YouTube with a minimal browser like w3m:
There are other public instances of "Invidious", but who knows how long these will last if the main project will end in September:
Will post details if I actually get 'searx' up and running though, as I'm looking forward to it.
July 28, 2020
I'm back to just using regular Emacs.
I tried Doom Emacs out, and what bugged me is that the overall community's sentiment towards it is correct. Doom Emacs is really just someone else's config. Sure it might be faster than regular Emacs, but at least I know how I want my Emacs experience to be configured.
Also, using Doom Emacs with Org-Mode kind of sucks since the C-u C-c C-. command doesn't work right at all, which is SUPER crucial for my every-day ticket based work at my actual job.
Hence, I nuked Doom's configs and am back to plain Emacs. The one thing I did like was the auto-completion sections within the mini-buffer at the bottom of Emacs, as well as the recently found files part in the intro-menu.
Other than that, eh.
Anyway, here's some cool links to check out for old-school style internet fun, or just fun in a Linux terminal:
Here's a cool old site from the early days of the Internet as I found this randomly after searching for "Tera Melos" online, worth it to check out as his page is pretty extensive:
From the guy who made 'wttr.in' which is that cool Weather based internet service I've used for the "Weather" tab on my site, this person listed some pretty neat console services that I found to be cool:
Notable Highlights:
Finding remote work through terminal, very cool:
The "Telnet/SSH-based games:" section is pretty cool too, so all you have to do is run the following commands in a Linux terminal to play some neat games via 'ssh', 'nc', or 'telnet':
- ssh sshtron.zachlatta.com ~> snake game; play with AWSD keys
- ssh netris.rocketnine.space — multiplayer tetris
- ssh play@ascii.town — 2048 (source)
- ssh gameroom@bitreich.org - 11 arcade games
- ssh play@anonymine-demo.oskog97.com -p 2222 — guess free minesweeper; Pass: play
- ssh twenex@sdf.org — play various games including checkers
- ssh intricacy@sshgames.thegonz.net - Competitive puzzle; password: intricacy
- ssh simulchess@sshgames.thegonz.net - Multiplayer Chess; password: simulchess
- ssh pacman:pacman@antimirov.net - Pacman; password: pacman
- ssh lagrogue@sshgames.thegonz.net - Roguelike; password: lag
- ssh ckhet@sshgames.thegonz.net - Khet; password: ckhet
- ssh slashem@slashem.me - nethack and others
- ssh rodney@rlgallery.org - rogue; password: yendor
- ssh pong.brk.st - singleplayer pong
- ssh tty.sdf.org - requires you to make an account first
- nc aardmud.org 23 — MUD (MUD list here, also works with telnet)
- nc freechess.org 23 — Chess Game (also works with telnet)
- nc igs.joyjoy.net 6969 - play/watch the game of Go (also works with telnet))
- nc fibs.com 4321 - multiplayer backgammon (also works with telnet)
- telnet dungeon.name 20028 - infinite cave adventure
- telnet milek7.gq — games: Pong, Break out, Tetris
- telnet mtrek.com 1701 — Star Trek
- telnet telehack.com
Have fun :)
July 27, 2020
I was able to utilize a few Crontab commands to update the weather part of the page.
Crontab based 'wget' commands are as follows:
*/5 * * * * wget -O /var/www/musimatic/images/newyorkweather.png wttr.in/newyork.png
*/5 * * * * wget -O /var/www/musimatic/images/nashvilleweather.png wttr.in/nashville.png
*/5 * * * * wget -O /var/www/musimatic/images/scrantonweather.png wttr.in/scranton.png
*/5 * * * * wget -O /var/www/musimatic/images/poincianaweather.png wttr.in/poinciana.png
*/5 * * * * wget -O /var/www/musimatic/images/newyorkweather.gif https://radar.weather.gov/lite/N0R/OKX_loop.gif
*/5 * * * * wget -O /var/www/musimatic/images/nashvilleweather.gif https://radar.weather.gov/lite/N0R/OHX_loop.gif
*/5 * * * * wget -O /var/www/musimatic/images/scrantonweather.gif https://radar.weather.gov/lite/N0R/BGM_loop.gif
*/5 * * * * wget -O /var/www/musimatic/images/poincianaweather.gif https://radar.weather.gov/lite/N0R/TBW_loop.gif
The end result can be found here: https://www.musimatic.net/weather.html
If you ever want to find a specific area's weather from the National Weather Service's Apache server, you'll have to dig a bit yourself here: https://radar.weather.gov/lite/
If you ever want to bookmark a specific section from the 'Weather' page, you can use one of the following links as they take advantage of the "#" id element values of the specific locations within the links themselves:
Weather Forecast Links:
- https://www.musimatic.net/weather.html#newyorkweather
- https://www.musimatic.net/weather.html#nashvilleweather
- https://www.musimatic.net/weather.html#scrantonweather
- https://www.musimatic.net/weather.html#poincianaweather
Weather Radar Links:
July 26, 2020
I was able to figure out with the help of IRC how to actually git clone my repos as the 'git' user:
For example, if I had SSH access to my VPS on another machine, then I could do:
git clone -v git@www.musimatic.net:/var/www/git/dotfiles.git
Also, I learned how to configure Virtualbox on a Windows 10 'host' to run Devuan inside of it as the 'guest' with the help of this guide:
The only other tweak I had to do was make sure that in Virtualbox, I had went into Settings (Gear Icon) > Display > Graphics Controller: VMSVGA
Pretty stoked because I decked out the Devuan based virtual machine on my Windows 10 work computer with my dotfiles config, which means I can potentially work primarily in a Linux environment in the near future and never have to touch Windoze for work ever again unless in a VM or something locally on that machine.
July 25, 2020
I am happy to note that my git repos are live at the following site: https://git.musimatic.net/
What does this mean?
You can see the progress of any of my own public repos that I contribute to, so that you can steal my 'dotfiles' configurations for your own use to tweak for example.
For example, I will literally be able to go to another machine, pull down related configurations, and it would look exactly like my current machine without any problems.
I've been at this for a dang week, and I'm happy to note that its all because I had to be inclusive of ports INCLUDING port 80, which is the specific port that helps handle HTTP requests on the web.
Because the lack of Nginx docs or guides on how to specifically configure "GitWeb" to work, I'm literally going to copy and paste my working config so that anyone using 'nginx' can benefit from it as I had to use over 5 different separate guides to even attempt to figure it out:
NOTE: You'll have to place this in your '/etc/nginx/sites-available' directory on your website's VPS as a file name of your choose, for ex: you can save it as 'git':
server {
listen 80;
listen [::]:80;
server_name git.musimatic.net www.git.musimatic.net;
location / {
root /usr/share/gitweb;
index index.cgi;
}
location /index.cgi {
root /usr/share/gitweb/;
include fastcgi_params;
gzip off;
fastcgi_param SCRIPT_NAME $uri;
fastcgi_param GITWEB_CONFIG /etc/gitweb.conf;
fastcgi_pass unix:/var/run/fcgiwrap.socket;
}
}
Here are the specific links I found to be IMMENSELY useful in this process of using 'nginx' with Debian 10 and GitWeb:
- https://www.vultr.com/docs/http-git-server-with-nginx-on-debian-8
- https://mescanef.net/blog/2016/05/git-http-backend-with-nginx-and-fastcgi/
- https://gist.github.com/mcxiaoke/055af99e86f8e8d3176e
- https://palatana.wordpress.com/2014/11/17/gitweb-with-nginx-on-ubuntu/
- https://misterpinchy.wordpress.com/2012/11/01/gitweb-and-nginx/
- https://stackoverflow.com/questions/6414227/how-to-serve-git-through-http-via-nginx-with-user-password
July 17, 2020
I am in the process of trying to figure out how to get the Git bare repos online as public repos on this site.
Unfortunately, the Git book is mostly 'Apache2' based, which doesn't help because this site is now running using 'nginx'.
However I did find a couple links that I have to go through to make some decisions on how to properly configure and serve the following directory: git.musimatic.net
I'm also debating whether or not I should even serve the Git repos in the var/www directory on this site as well or keep to using the /srv/git repo as mentioned in the Git book.
Helpful reference links on how to possibly do this include:
- https://git-scm.com/book/en/v2/Git-on-the-Server-Smart-HTTP
- https://serverfault.com/questions/483726/how-to-make-git-smart-http-transport-work-on-nginx
- https://www.toofishes.net/blog/git-smart-http-transport-nginx/
- https://www.howtoforge.com/tutorial/ubuntu-git-server-installation/
Ideally, my Git repos would look like the one from 'Suckless.org', which looks awesome, minimum, and just perfect:
Things To To Do This Weekend:
- Work on my C based ncurses project as well.
- Learn more JS for work and keep utilizing this practice site as a means to apply the techniques from the related chapter: https://musimatic.net/FakeWebsites/PageCeption/index.html
- Work on my "ArmoryApp" NodeJS / Electron based app for work as well. It's coming along pretty nicely as I'm able to automatically pull logs for the one app I help do tech support for, which is pretty cool.
- Configure the Devuan work based VM so I can move all my work inside of it.
- Keep researching how to install Gentoo and configure the related installation guide.
- Deploy 'Searx' public instance on this site now that I have the desired 'nginx' + 'Debian' combo that's preferred
- Make Awk script to sort ~/.w3m/bookmark.html based on <h2></h2> tags present
- Make LinkedIn Job Scraper based on their API so I don't have to use LinkedIn anymore +10k points for making an NCURSES interface to read results afterwards
- Learn more from gotbletu videos
- Work on weather based webscraper based on attr.in, and the National Weather Service Apache server to display the contents on a webpage
- Turn Emacs into a C based IDE with this guide: https://martinsosic.com/development/emacs/2017/12/09/emacs-cpp-ide.html
July 16, 2020
I am happy to note that this site is now running on 'nginx' thanks to Luke Smith's video on the topic: https://www.youtube.com/watch?v=OWAqilIVNgE
Pretty stoked because dealing with Apache configurations was a bit of a pain, especially since I wanted to add a Git server to this site, as well as Searx.
We are now running on a Debian 10 based VPS droplet running on Digital Ocean's VPS servers in NY :).
I've been meaning to do this for a very long time, so I'm pretty glad.
I finished learning C, and went through that ncurses guide. I've been modifying two or more projects to get the hang of it, and am basically making a glorified menu that runs a few Git commands, which is about it.
Does it work yet? Not really.
Can I show it off yet? Not yet, because I'd need to setup the Git server first.
However, because I'm now using Debian 10 on this box, it should be way easier to do it than friggin' Ubuntu was.
Plus, since I now have the great combo of 'nginx' + Debian 10, this means I can finally revisit being able to host my own Searx instance as well so stay tuned for that.
Other than that, I've been debating making a LinkedIn Job Webscraper that would access the LinkedIn Job API since I hate logging into that site, and be constantly spammed with unnecessary news articles.
LinkedIn, like other platforms, needs to learn its place, and just stick to jobs, and nothing else.
Here's 'nginx' for reference: https://nginx.org/en/download.html?_ga=2.125714506.138062483.1594951322-1859715614.1594951322
July 3, 2020
Regarding My Digital Art:
I got good news in that I was able to finally get around to modify the existing JS code for the "GIFs" section to make the Digital Art 2020 and Digital Art 2019 "back", "random", and "forward" buttons actually work.
You can check it out here: http://musimatic.net/art.html
Regarding 'Audacity':
I tried to get Audacity to work on my Devuan laptop, but attempting to do overdub tracks made weird sounds on the overdubbed track. I don't think it's due to CPU issues present, but I'll see if I can just at least record demos with Audacity on the desktop instead. It would have been nice to do demos on the fly with the laptop alongside my Focusrite Solo (2nd gen) audio interface, and then master songs in Reaper on the desktop later, but ah well.
So that would have to be the third fail of the week, but ah well, good and bad things happen in 3's, so I'm hoping that stupid bad luck spree is over.
Regarding 'GNU Stow':
I re-arranged my dotfiles config and plan on deploying them with GNU Stow, so that I can go to another machine, and use the following stow command so I can deploy configuration dotfiles easily:
stow -t ~ *
This basically sets the target directory as the '~/' or $HOME directory where normally all your dotfiles are located anyway since I usually place my dotfiles in its own repo, ex: ~/programming/dotfiles. By doing this, Stow would properly place them into the home directory on the target machine.
This helps a lot if you want to have your nice looking AwesomeWM or Openbox config to be present in two seconds on a newly installed machine.
'GNU Stow' can be found here: https://www.gnu.org/software/stow/
'AwesomeWM' can be found here: https://awesomewm.org/
Slight meme-tastic picture of AwesomeWM in use (from the AwesomeWM website):
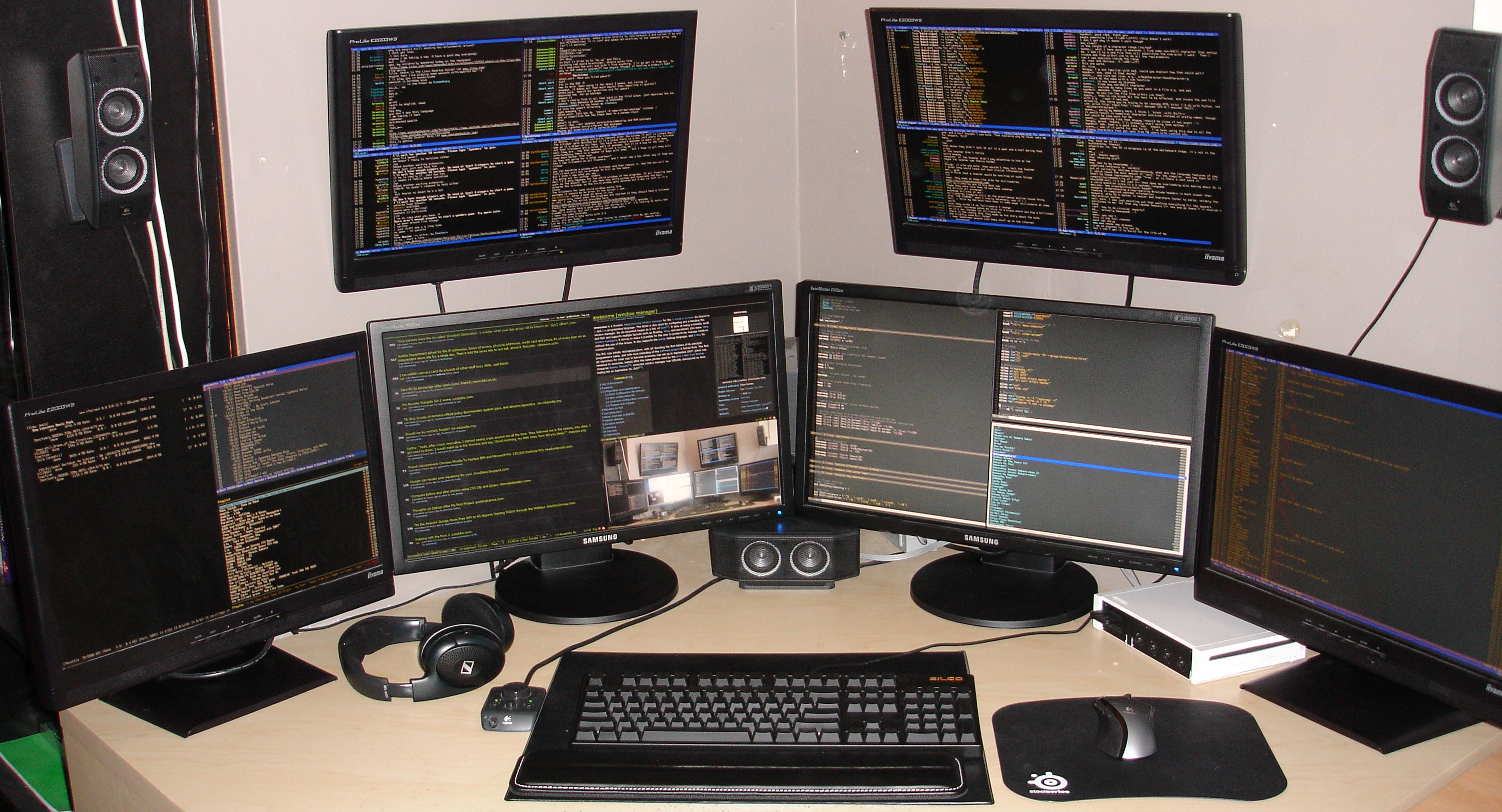
'Openbox' can be found here: http://openbox.org/wiki/Main_Page
I'd like to probably overhaul the design of this blog post portion of my site since solarize themes aren't really my thing anymore, but I at least got to figure out how to change the CSS styling of an Emacs Org-Mode document. This is important since that's all this page is at the end of the day, just an blog.html file that was exported from the 'blog.org' file. It's hilarious that you can just make a website using an Org mode file, and I still plan on carrying the torch as there's no really big reason for wasting extra time in configuring HTML/CSS with JS just for blog posts anyway.
Regarding 'Emacs Org-Mode' Being Used For Finance Based CSV's:
First of all, I've been using 'sc-im' to do my finances for the past few months. I first attempted to document my attempts of the commands I wanted to do on each of the CSV files involved in the process, which include the credit card and checking account statement CSV files. However, after combing through the 'sc-im' internal help a few times, and their GitHub page, it doesn't seem that there's an easy way to do commands like 'dc' or "Delete Column" with external scripting at all.
I then got the idea that if I like Emacs so much, then why the heck SHOULDN'T I use it for CSV spreadsheets either.
Then, I realized after a little bit of searching that you can easily make your finance .csv files into Org-Mode tables by opening up the .csv file in Emacs, select all the values, copy it into your copy / paste buffer, and then paste the values into a new .org document.
Then, in the .org document, highlight all of the values, and then use the following command:
C-c |
This turns the entire sheet into an Org-Mode table, which is sweet!
I learned this from this Stack Overflow post: https://stackoverflow.com/questions/1241581/emacs-import-a-csv-into-org-mode
Since I wanted to learn how formulas in Emacs Org Mode tables work, I also learned that the following command will turn on row and column numbers to make formulas easier in Org-Mode:
C-c }
More info on how spreadsheet formulas work in Emacs Org-Mode can be found here: https://orgmode.org/worg/org-tutorials/org-spreadsheet-intro.html
Very happy to be moving on past 'sc-im' for this purpose alone. 'sc-im' neat for basic spreadsheets but was just a bit disappointing in terms of its scripting ability to manipulate existing .csv files but I guess there's other terminal programs for that purpose too.
'sc-im' can be found here: https://github.com/andmarti1424/sc-im
July 2, 2020
I debated installing 'firefox-esr' since Qutebrowser just wasn't working with YouTube's sign in page at all. It turns out that the dumb blanket error message they give when you try logging into YouTube with Qutebrowser, "you're using "a browser […] that doesn't allow us to keep your account secure" is totally a lie.
They just want more control over your browsing experience to monitor your viewing habits, and to make ad revenue, plain and simple. This is explained more in detail within the following workaround found here from Qutebrowser's GitHub page: https://github.com/qutebrowser/qutebrowser/issues/5182
Here's the workaround you have to use within Qutebrowser itself to make it work with YouTube's Google Sign-In page: :set -u https://accounts.google.com/* content.headers.user_agent 'Mozilla/5.0 (X11; Linux x86_64; rv:57.0) Gecko/20100101 Firefox/57.0'
What does this workaround do?
From the looks of it, it utilizes a Mozilla based agent that makes it seem like you're using a Mozilla based browser to Google, when in reality, you're still using Qutebrowser.
What does this prove?
Don't depend on Google for anything. I literally only login to maybe check related content to see if there's anything else cool within the Linux YouTuber scene these days to add to my RSS feed list. However, like most based Linux users nowadays, I mostly just download videos from RSS feeds from Newsboat with 'youtube-dl' to be later viewed with 'mpv' anyway because streaming videos is a waste of internet bandwidth, time, energy, and patience.
Just in case you're one of those people stuck into the "Oh, but I NEED Google for things like Gmail, Google Drive" etc, then you need to read these blog posts and wake up to an improved user experience for better alternatives: https://www.kylepiira.com/2020/01/09/why-i-quit-google/
Also, here are better 'Gmail' alternatives, or even 'Outlook' for that matter since I despise Microsoft as well: https://tutanota.com/ https://protonmail.com/ https://posteo.de/en
I personally have used 'Fastmail' so far, and I like it a lot. However, for good things come a price, so even though its not free like all of these other email clients where YOU are the product, it does give me a little peace of mind at least. The middle tier plan they have is $50 a year, and seems worth it. If not, I'll probably just host my own email server later on a VPS next year if I don't like it, but I'll keep it for now: https://www.fastmail.com/login/
Regarding 'searx': Oh man, what a fail this was too.
Why is it that every deployable web app just does NOT work nicely with Ubuntu 20.04? This is the second fail I've had this week since the first was Jitsi Meet which just did not play nicely with Ubuntu 20.04 + Apache running this site.
Now, after a few hours of trying, I just could not get Searx to work at all.
It was so frustrating because they changed literally EVERYTHING about their installation process within a month of Luke Smith's video: https://www.youtube.com/watch?v=oufXi3e-VuA
This makes his recent video absolutely useless in comparison. Even though there are installation scripts, its just mindboggling how nice and easy the installation process was a month ago via the instructions on screen on Luke's video vs the current mess that exists: http://asciimoo.github.io/searx/admin/installation-searx.html
I DID however find a guide on how to deploy this on the Raspberry Pi, but this would be for a local instance, and not necessarily a public instance for people in my family unfortunately: https://raspiblog.noblogs.org/post/2018/01/27/installing-searx-with-apache-and-morty/
Let's just say I am not going to be attempting deploying another web app for family any time soon. I would be more inclined for changing the entire backend of this website to 'nginx' instead of Apache2, which I aimed to do at some point anyway, so this is probably just a lesson to never use Ubuntu as a server basis, and to finally overhaul Apache with 'nginx' instead.
The only thing I'd have to recreate is the bare Git repositories I created earlier, but that can ALWAYS be re-done.
Anyway, moving on to more positive things and projects, and will just label this as another attempt I guess. The best way to learn is to fail, so I plan on just moving on.
July 1, 2020
After much deliberating, I was able to kind of get 'transmission' working on both my local machine and my Raspberry Pi since I wanted to download safe files like Linux distro ISOs, wallpapers, etc.
Think of 'transmission' as one of your typical BitTorrenting clients, but with a bit more control, and a heck of a lot of less ads and potential for getting viruses.
The reason why you would want to have 'transmission' on a Raspberry Pi device is that you don't want to waste so much power downloading things like torrents, images, etc, when you can do it on a low power device to do it all day for you if needed. The capabilities of this kind of setup are cool because if you hook up an external HDD to a Raspberry Pi, you can also debate how to make it work on scraping the internet for data with cool scraper projects.
For example, I would love to somehow scrape the "Wayback Machine" for old school icons, and early internet assets as it brings lovely memories of Yahoo! Geocities back to mind. Ah yes, back when everyone had their own personal website, although heavily templated. Looking back, its easy to say that having your own site WAS the internet, and the direction it took really sucks.
Face it, its pretty sad when most people only can think of maybe 5 major sites that they visit on a daily basis. Back then, you had tons of splintering personal sites, all for the basis of being able to express yourself freely. Sure the DotCom bubble was a meme in itself, but I remember all of those weird personal pages that had highly specific content. Ex: If you searched for old school game art on Yahoo! back then, you would likely land on some dude's personal website where he hoarded tons of video game sprite sheets that he somehow collected from using ROM palette tools. Sure there's tons of community sites that still do this, but it meant more when a guy's whole personal page was just dedicated to solely that purpose, which shows the life and soul of the early internet.
That makes me want to grab every Pokemon based sprite sheet from late 90's websites from The Wayback Machine ASAP :), good times.
'The Wayback Machine' can be found here, and chances are, your old personal website or favorite site was archived, so check it out: https://web.archive.org/
You can also laugh and see how badly I designed this site over time, but also keep in mind just how restricted I was in terms of being able to develop this site while on my lunch break at my first tech job. Geez, I used to have to SSH into a shared hosted site just to be able to modify this website, but nowadays I just work on this site locally, and then use 'rsync' to post my changes onto the website that's hosted by a private VPS.
Here's the old past history of 'musimatic.net' for you to enjoy (and wow did I love a good purple color scheme early on haha): https://web.archive.org/web/2019*/www.musimatic.net
Back to the topic of 'transmission': I tried to make it easy by modifying the 'settings.json' file in the '/etc/transmission-daemon' directory to accommdate the '~/Downloads' folder. However, after trying two or three workarounds in this Stack Exchange post, I gave up: https://askubuntu.com/questions/221081/permission-denied-when-downloading-with-transmission-daemon
However, I will work on creating a related symlink that will link to the 'var/lib/transmission-daemon/downloads' directory as 'torrents' or something in my '~' home directory.
I believe all I would maybe have to do is just to add the 'sam' user to the 'debian-transmission' group anyway. This is important because for the time being, in order to access that particular directory, '/var/lib/transmission-daemon/downloads', I would literally have to use sudo priviledges to do so. This is fine for now, but might be easier if I just add the user to the 'debian-transmission' group appropriately.
Anyway, I'm pretty happy it works at least, but let's just say 'transmission' out of the box is NOT newbie friendly in that respect. However, it is a pretty powerful torrenting tool that can be run on any lower end PC if needed, which is super cool.
'transmission' can be found here, and can be used with CLI or with a GUI; https://transmissionbt.com/
Also, if you're someone like me who's just looking for legit torrents for nice purposes like 'wallpapers', Linux ISOs, etc, I found a good blog post for relevant torrent sites in 2020, which is new to me as I only knew Pirate Bay back in the day like everyone else: https://www.vpnmentor.com/blog/10-best-torrent-websites/
As always, try the CLI version of 'transmission' instead.
In Debian distributions, you're gonna need 'transmission-daemon' and 'transmission-remote-cli' installed, so you're going to want to do the following command in terminal to install the terminal version of transmission:
apt install transmission-daemon transmission-remote-cli
After installing the two parts listed above, I would follow along with gotbletu's YouTube videos on the topic as its not too easy to figure out on your own, so here's a related playlist to help figure it out: https://www.youtube.com/playlist?list=PLqv94xWU9zZ05Dbc551z14Eerj2xPWyVt
'gotbletu' shows a lot of bash aliases you can put in your .bashrc config to make using 'transmission' a lot easier too, so I highly recommend checking those out, and any of his other videos since he's pretty good at just demoing Linux utilities in general.
Plus, you can always access your downloads at any time in any browser by going to this website URL once you have the transmission daemon up and running: http://localhost:9091/transmission/web/
One more good thing taken care of this week.
Next, I'll attempt adding a private 'Searx' instance. It's a dang shame about how badly Jitsi Meet doesn't play well with latest Debian or Ubuntu distros though. However, I learned a lot in attempting to deploy it though. I probably might even resort to just deploying an IRC based server like 'XMPP' or something: https://xmpp.org
Also, I'd like to do a few screencasts with gifs using 'screenkey' as well as the idea of being able to showcase Linux utilities with screencasts is probably the next best thing I could do to emulate my favorite Inconsolation blog. That and being able to utilize thumbnails correctly with Emacs Org mode would help as I'd love to replicate his Wordpress site's ability to just do thumbnails out of the box.
Here's 'screenkey' for reference: https://gitlab.com/screenkey/screenkey
Stay tuned :)
June 30, 2020
I figured out how to handle images with Emacs Org Mode and related formatting to make this blog a little more lively with images: https://orgmode.org/worg/org-tutorials/images-and-xhtml-export.html https://orgmode.org/manual/Images-in-HTML-export.html#Images-in-HTML-export
I figured out how to use thumbnails with the 'mogrify' command from 'imagemagick' will become SUPER useful later as I make more and more useful tutorials on this site with just .org mode sites for specific topics: https://imagemagick.org/Usage/thumbnails/
Here's the relevant 'mogrify' command: mogrify -format png -path thumbs/ -thumbnail 100x100 (name of image)
For example, I created a thumbnail for later use in the weather portion of this blog post: mogrify -format png -path thumbs/ -thumbnail 300x300 weatherCommandScreenshot1.png
I also stole this 'Dark Solarize' theme from this GitHub page and used it for my own blog page as well: https://github.com/saccohuo/export-themes/blob/master/org-css/solarized-dark.css
I also combed through the National Weather Service (NWS)'s Apache server, and found the following locations to be useful for myself and for my family's purposes.
They're GIF's of the National Weather Service's doppler radar so you can check if its going to rain or precipitate in your area.
This is the NWS's Apache server where you can comb through each of the '.png' images or gif's present to find out your area: https://radar.weather.gov/lite/N0R/
NOTE: I THINK they're based on airport codes, but let's just say I spent a good amount of time trying to figure out which ones are relevant, and it wasn't easy since Scranton for example doesn't have a major airport connected to the National Weather Service, so you kind of have to look at the doppler for a nearby city instead.
Here's some useful weather doppler gifs I plan on re-hosting on this site at a later point with a CRON job on a related "Weather" tab on the site for family to use:







On that same note, you can also just utilize the 'curl' command on Linux to grab your local weather information.
Here are two example screenshots in which I'm using the following command, 'curl wttr.in', which will obtain the latest weather information for Nashville, TN:


In terms of re-designing this blog portion of the site, I plan on re-designing this blog portion of my site with the Org-Mode based themes from this guy's GitHub as I REALLY like the "ReadTheDocs" theme he's got: https://github.com/fniessen/org-html-themes
Before I used to use the "Ox-TWBS" plugin for Emacs to export this blog into a Twitter Bootstrap CSS styled site. However, after moving into Doom Emacs, I realized I should play around with the default styling a bit for this blog, so I plan on exploring this a bit further.
Speaking of re-design, I STILL need to fix those the "Images" section on this site as I think its just a matter of not having the correct permissions to allow a user to view my old artwork.
I'm in the process of wiping my desktop drive so I can install Devuan on it, and just test out Linux distros in VM's to find some cool setups with some Window Managers. It's more so that I can possibly move to an Arch based distro without systemd sometime later this year, but didn't want to blow up the desktop computer itself like my last attempt with just an Arch install with Steam.
Speaking of Steam and 'muh games', I plan on really only buying games for my Nintendo Switch and to keep computers as just that. I think Desktops are cool for making music or possibly to aid in emulating older games, but past that, playing games on a Desktop computer is a bit of a waste of time. I'd rather be building some cool stuff with that same time.
Also, I'm kind of tired of playing a game on a console, I know when to put it down. However, when I had the latter on Steam, I could quickly forget the time, and kind of regret it later. Anyway, the cryptocurrency bros ruined the PC market anyway, so there's no point in building high end machines unless you plan on running VM's. Even for that use case scenario, you don't even need a top tier video card as well. You would just need to make sure you have a decent CPU and RAM. I do plan on getting a librebooted computer though, so I'm not sure how long my wanting to do VM's on a regular basis will last in that case.
These things I wish I knew before, but at least I have an ok Desktop computer for today's standards to just run VM's on, and to later become a cool media server or something. I've been using it for emulators and have been having a good time with it. I plan on making some demos with Audacity, and then remaster tracks within Reaper with MIDI for that newer band I'm going to create called "Sieges", which will be a Dungeon Synth band. I'm still debating if I should just release it on the Bandcamp platform, or just host it on my own site, with a custom CSS style sheet to mimic Bandcamp since all I would have to do is to just allow people to hit play in some kind of web based music player. I wouldn't charge for the music either, and will most likely do donation incentives through Patreon or something similar.
I also kind of debate making Linux tutorial type videos for sites like Library or BitChute, and not necessarily YouTube since YouTube is just become more and more censored as the years go on. I'd rather be on a platform where I'm welcome than be banned for being conservative or something. However, I might take up Luke Smith's philosophy to maybe just use YouTube until you get banned just to piss them off or something for being on their platform regardless as its still sort of the world's marketplace in the truest sense at the moment.
I'm gonna try deploying Searx sometime later today as a private instance so that should be fun as well. Feels good to put the power back into my hands, and to take back all of that free telemetry ad money from Google and DuckDuckGo.
Also, I plan on ripping this CD I got the other week since I couldn't find it on Bandcamp or Spotify anymore (though I don't use Spotify at all, it used to only be available on there for a long time): https://www.discogs.com/sell/release/9525344?ev=rb
Probably the most funniest, down to earth album you will ever hear in your life. I don't know why, but I liked it a lot as this guy is so honest, but its "so bad, it's good" if that makes sense.
I'm gonna try finding a CLI based CD ripper on these good command-line based blogs: https://kmandla.wordpress.com/software/ https://inconsolation.wordpress.com/
Have fun for now, stay well!
June 29, 2020
I tried to install Jitsi Meet but failed because of these two dumb issues that still haven't been resolved by the Jitsi team:
Related GitHub issue on the same topic: https://github.com/jitsi/jitsi-meet/issues/6371
Duplicate issue where they're still working on this issue: https://github.com/jitsi/jitsi-meet/pull/6627
Basically, in a nutshell, they're using "Certbot-Auto", which has been deprecated for releases like Ubuntu 20.04.
This means that unless they patch this into the installation shell scripts, I would manually have to apply a workaround using 'certbot' to install the appropriate certificate, and manually configure a lot of the files present to get it to run. I don't feel comfortable doing that so, I just nuked the Jitsi Meet private instance I created.
On the flip side, I plan on deploying a private Searx instance, so that I never have to use Google or DuckDuckGo again myself, so that'll be fun.
This time though, I'll read through the whole documentation to make sure that Searx is cool with Ubuntu 20.04.
'searx' can be found here, and you can actively use some public instances of it, though its more recommended to host your own instance to make sure its as secure as possible since YOU control it in that case: https://searx.me/
On a good note, I was able to install Doom Emacs just fine, and I have gotta say, it looks, feels, and just responds amazingly. I know other Emacs enthusiasts would just say "its someone else's config" but I've been using Vanilla Emacs for quite a bit, and there's just so much that should have been added right out of the box as a default like this.
So it goes without saying, I'm back to using Vim style bindings, and I'm pretty happy about it. I still know Emacs style bindings for other shell programs wherever needed, but let's all agree Vim bindings are 100x better and more intuitive. Those who think they've memorized every C-c or C-o style binding in Emacs is insane, or is straight up lying. Some are intuitive for starters but when you delve into Org-mode with vanilla Emacs bindings, then you realize its almost impossible for a normal person to remember half of the bindings anyway.
'Doom Emacs' can be found here: https://github.com/hlissner/doom-emacs
Otherwise, I have the week off, and am doing the following checklist of things to do to keep myself occupied, and have been pretty happy about the progress so far:
Checklist of things to do [11/28]
- Go over moving inventory list
- Relax
- Figure out Neomutt for Fastmail email address
- Figure out how to use gnupg keys for Neomutt
- Attempt to deploy Jitsi on private instance on website
- Deploy Searx on private instance on website
- Download and install Doom Macs
- Figure out how to create a SC-IM based script with commands to automatically format the cc and c files every week
- Install Audacity & Reaper (one for demos, one for mastering
- Install Devuan on the main 2 gig SSD and try out VM's with Virtualbox
- Start "Sieges" band
- Figure out how to get Transmission-remote working
- Play around with Raspberry Pi to make it a downloading agent via SSH or FTP
- Look into how to possibly obtain recipes through a terminal API
- Learn more C
- Research bug zappers for annoying gnats
- Look up which ports my website is using, allow those ports, and block all others.
- Look up Captain Crunch (2600) biography: hard to find, not worth buying $45 signed copy
- Activate Tracphone with new sim card
- Figure out how to split AwesomeWM config across multiple files
- Download gotbletu's playlists of content I want to learn
- Watch gotbletu's video on gnu stow and utilize it for dotfiles repo
- Check out Brody Robertson's Linux vids on U-Tube and possibly add him to Newsboat Urls config
- Look into LinkedIn's Job Search API, and work on making a Bash based console app to do curl requests
- Debate how to use RMWeb Reports API for work based app (ArmoryApp)
- Activate Tracphone with new sim card
- Re-organize Newsboat "urls" to be more accurate for YouTube section
June 24, 2020
I got up to Chapter 5 for that C Programming Language book today.
C is such a cool language, and the power of it can't be overstated. It is embedded in so many devices to the point where if you can program in C, you can pretty much use it to program on anything (that isn't requiring pure assembly language of course :D).
I'm debating what the best things I can do to install on the work based Devuan VM I have so far. I'd like to maybe consider using something like "exwm" which is basically Emacs as a window manager, which is such a neat concept. The idea is that everything would be Emacs based by default. This would be cool because then you can basically run Doom Emacs as well for text editing so that you can use the glorious Vim bindings, but use the pretty decent Emacs standard bindings for other things as well.
Just an fyi, Doom Emacs doesn't have any evil connotation. It's named that way because it adds 'evil' mode style bindings (hence 'vi' in 'evil') which allow you to use 'Vi' or 'Vim' style key bindings within Emacs. Personally, I think Vim's bindings are far superior than Emacs. I love being able to write this blog with Emacs alongside the 'org-twbs' export plugin with standard Emacs keybindings. However, past using Emacs keybindings in Org Mode to write documents, I think they're pretty terrible for actual coding situations. More or less, they're very very very hard to remember and way less intuitive than Vim's default bindings.
However, for those that totally complain about Emacs keybindings as being 'painful' probably don't realize that you can totally use your left thumb for the 'meta' or 'alt' key based bindings, and also use the right ring finger for the 'p' key. Too many times I see the term, "Emacs pinkie", being used, and I just have to laugh since most likely the person is attemping to use the 'p' key with their pinkie, when the right ring finger is almost near the same position anyway.
Just to set the record straight, I like Emacs bindings for Org Mode and some terminal related applications that happen to have them, but I prefer Vim bindings for any coding situations (hence Doom Macs is probably what I should be using going forward).
'exwm' can be found here: https://github.com/ch11ng/exwm
'Doom Emacs' can be found here: https://github.com/hlissner/doom-emacs
'Emacs' can be found here: https://www.gnu.org/software/emacs/
'Vim' can be found here: https://www.vim.org/
I've installed DietPi on the Raspberry Pi, and just plan to use it as a downloading agent. I might get a separate external drive for it to store media so that I can use it to scrape the net for content, and then later access it afterwards purely through SFTP or ssh to retrieve the data from it. That alone is such a cool idea because if you booted your huge desktop computer just to do menial tasks such as downloading wallpapers, then its a bit of a waste of energy.
I'm thinking of the possibilities of what I could do to either deploy Jitsi or Jami as a private instance for family communication purposes. I find both pretty fascinating as they can be used on most platforms AND they are secure communication programs.
'Jitsi' can be found here: https://jitsi.org/
'Jami' can be found here: https://jami.net/
I also wonder what can be done with mesh networks such as 'gnunet'. The idea of being able to link computers and devices together without depending upon a centralized internet service company sounds amazing. This idea alone could be used for sharing files, communication, etc, even when a grid is potentially knocked down. Sounds pretty useful given the times.
'gnunet' can be found here: https://gnunet.org/en/
I'm planning on also figuring out what to do next week since I have it off from work. Most likely, I might go ahead and start that one-man dungeon synth band, "Sieges", as I've been planning in my head forever. That band will probably include loops, drum samples, and keyboard riffs. I'd like it to be the anti-thesis of what you can typically find in this genre under Bandcamp, as I would like to prove that you can create some cool themed music in that genre without having it to be so dark or dreary. As seen by a few select bands in that genre, I think the best encompassing idea of that genre is to utilize RPG video game style music but with relaxing themes. However, I think a lot of those great ideas are kind of lost between the all too many dreary dark albums of that genre on Bandcamp, so here's to proving that positive dungeon synth music can be made.
Never stop learning, and have fun with Linux :) God Bless.
June 23, 2020
Thanks to my dude, Luke Smith, I was able to finally get all my Git repositories back on my site with his latest video: https://www.invidio.us/watch?v=ju9loeXNVW0
If you've never used "www.invidio.us" before, then you probably should, as it allows you to check out YouTube videos in terminal based browsers like 'w3m' easily.
If you attempt to use vanilla YouTube's site in 'w3m', Google complains about the lack of the JS scripts that are not present because 'w3m' runs purely based on HTML alone, so they can't track you nor give you targeted ads.
Here's w3m for reference, as it is an awesome browser I use every day: http://w3m.sourceforge.net/
With this in mind, I'll have to wait until Luke's next Git video to finish off my bare Git repos, but I'm glad to say I have killed any notion of having to depend on Microsoft for GitHub since all I ever wanted was bare Git repositories anyway for my projects.
Other than that, still learning C, and dived slightly into 'ncurses' with the one guide I got from Internet Archive.
Pretty cool stuff, and compared to the C programming book, 'ncurses' is pretty light in terms of the difficulty in figuring it out.
I plan on making a 'ncurses' program to move over my dotfiles, and will host it on my Git instance when I flesh out the beginnings of it so that anyone can pull it down.
I've been playing around with the idea of using a Linux VM purely for work, and just remoting in with a terminal based app for Slack, and just use WINE to emulate the Windows based programs I help do tech support for on a daily basis.
That thought alone is pretty based, and I'd still be playing by the rules for work as I'd be running a VM inside of Windows so that I could still get security updates to make work's IT team happy, but also keep myself motivated as my workflow would most likely increase 100% if I could just do everything with Emacs or Vim bindings :)
Also, I made further progress on creating the all-in-one ElectronJS based app for work. Though I've detested just how bloated web apps are, in this context, ElectronJS would allow me to create a GUI based app for my coworkers to make their workflow better, and their daily life doing ticket work easier. That alone is a cool motivator keeping me going these days in terms of working since the days have kind of been blending for months now.
I'm looking forward to the Florida move in a month since it'll be good to get away from a city center amidst all this crazy stuff going on.
Staying positive, and thankful that I can look forward to better things. On the flip side though, Nashville was so awesome. Its a shame, since I really saw myself trying to start a family out here before all this crazy stuff this year.
The food, people, and music were 100x better than anything I've experienced in the NY tri-state area. I'll definitely miss this place but I'm glad to have at least tried it out. However, I doubt live music will ever recover, so I guess its time to leave 'Music City' for good.
God help us all in these times, and stay safe. God Bless.
June 18, 2020
Learned three cool things this week regarding Linux.
First Thing:
This is Linux file permissions in a nutshell from what I learned:
- You first have '-' which is a file, or 'd' for a directory.
- Past that, you've got three groups: You, your group, and everyone else who can execute scripts on the machine.
- The options include 'r' for read, 'w' for write, and 'x' for execute.
- r: 4, w: 2, x: 1
And that's basically it.
This helps a ton when you're trying to figure out what in the heck '777' means in terms of file permissions or '755'.
You have to break down each part of the number provided.
Ex: '777' in file permissions means the following: You've got read ('r'), write ('w'), and execute ('x') access in ALL three groups. This means that YOU, YOUR GROUP, AND EVERYONE ELSE can do those three actions on the file.
This isn't the best option because you don't want someone to somehow hack into your system and just give them permission to files without even being granted access as the root user.
Second Thing:
This is how you can utilize the 'Newsboat' RSS program across several devices (aka your Android phone running Termux, your website via SSH, your Linux laptop, your Linux desktop, etc).
You can utilize Newsboat's '-e' parameter to export your current news feed into an .opml file.
You can also utilize Newsboat's 'E' parameter to export a copy of the list of articles you read.
You can then go to a totally separate machine, and utilize Newsboat's '-i' parameter to import your newsfeed.
Then you can use Newsboat's '-I' parameter to import the list of articles you already read on your previous devices.
In this kind of scenario, it's better to use your personal website as a midway station so that if you have access to the internet, then you can technically just sync all these devices by using 'wget' to pull down these two files from your 'public_html' folder.
You can even do the same thing with a separate device off the internet with a Raspberry Pi or even an Android device with Termux that has a mobile connection or is connected to WiFi.
Here's my latest Bash alias from my .bashrc config that incorporates these ideas, which I will probably modify to run on my website instead later on but is given as a cool example to possibly copy: alias ne="newsboat && newsboat -e >> ~/transformationStation/newsboat/currentNewsboatFeed.opml && newsboat -E ~/transformationStation/newsboat/articlesRead.txt"
Third Thing:
Here's a cool Bash function I made to change your wallpaper and color scheme with 'feh' and 'pywal'.
Afterwards, it copies the same image that was used by 'pywal' into Awesome Window Manager's theme configuration directory:
ranchw() { wal -i ~/Pictures/wallpapers/ && feh –bg-fill "$(< "${HOME}/.cache/wal/wal")" && cp $(< ~/.cache/wal/wal) ~/.config/awesome/themes/multicolor/wall.png && xrdb ~/.Xresources;
background=$(ex -sc '/\*background:/s/^\S\+\s\+//p|q!' ~/.cache/wal/colors.Xresources); foreground=$(ex -sc '\*foreground:/s/^§\+\s\+//p|q!' ~.cache/wal/colors.Xresources);
ex -c 'theme.bg_normal/s"[^"]\+""'$background'"/|wq' ~.config/awesome/themes/multicolor/theme.lua;
ex -c 'theme.fg_normal/s"[^"]\+""'$foreground'"/|wq' ~.config/awesome/themes/multicolor/theme.lua;
}
June 16, 2020
I was able to get sound working on my Devuan desktop, but let's just say Nvidia drivers are a pain to attempt to get running if you want to limit what's installed via 'pins' in the following directory: /etc/apt/preferences.d
This is important since I'd like to update my video card drivers to use game console emulators effectively, since the performance out of the box was pretty bad, which is weird since that desktop was an older gaming rig anyway, and I'm just using it for emulation and POSSIBLY music creation.
Might create a dungeon synth band with the moniker, "Sieges", since I've been wanting to do instrumental synth music, but be the absolutle anti-thesis of what they'd expect on Bandcamp since a lot of solo acts in that genre is pretty dark, when I'd rather it be a little more light-hearted and cheerful. That fact alone is kind of weird when a lot of people making dungeon synth albums are just ripping off game music ideas in the first place, so why the negativity? Who knows.
I've been learning C, and got to the third chapter of the "The C Programming Language (Second Edition)" book from the 80's. Neat stuff, though thank God I have the answers for the exercises as I am treating it more as a code along to learn enough C to pickup 'ncurses' based programs.
I might dive into trying to figure out Mutt with the manual this week.
I might also simultaneously go through the one 'ncurses' guide on how to make 'ncurses' based programs in C as well.
I would also like to figure out how to make a few hooks with 'Dired' mode in Emacs for my Org Mode TODO list for work. Moreso, I should just archive them into a giant directory and use the archive feature, or at least create a hook that would do the following items for me:
- Create tomorrow's directory in the directory above
- Copy over the contents of the current Todo List into tomorrow's
Todo List.
- Change the date at the top of the Todo List accordingly.
Also, I'd like to possibly change over the theme styling of this page using the "org-twbs-head" function: https://github.com/marsmining/ox-twbs/blob/d5ae9c3fb224d081d59d3686d619edf152523f09/ox-twbs.el#L987-L1002
Have fun for the rest of the week, stay safe :).
June 11, 2020
Minor point I learned the other night is to be able to pipe the 'pwd' command into the 'xsel' command.
This allows you to pipe the 'working directory' that you obtain with 'pwd' command into 'xsel' which copies your working directory onto your clipboard.
This allows you to be able to paste your working directory into another terminal pane in a multiplexer like tmux so that you can work on a similar task in the same directory.
Give this a try in a Linux terminal: pwd | xsel
You can also convert this to an 'alias' in your Bash terminal by using the following in your .bashrc config file ('pwc' in this context stands for 'print working copy'): alias pwc="pwd | xsel"
Oh, and I figured out how to finally do transparency in the terminal with urxvt terminal.
It turns out, you have to modify your .Xresources file to include the following:
! Transparency for urxvt: URxvt.depth: 32 URxvt.transparent: true URxvt.tintColor: white ! Set .shading to: 20 for slight transparency ! Set .shading to: 40 for fuller transparency URxvt.shading: 30
Here's what a glorious transparent terminal looks like (screenshots): Transparent Weechat Screenshot Transparent Newsboat Screenshot Transparent W3M Screenshot
I took the advice on how to adjust my .Xresources file accordingly from this link, so all creds to the answer to the OP on this Arch Linux forum post: https://bbs.archlinux.org/viewtopic.php?id=117543
June 10, 2020
I installed Devuan on my Desktop computer yesterday with Openbox, and I must say, its a pretty comfy experience.
I was trying to get some game emulators working, but sound isn't working on the Scarlett Solo audio interface I have.
I got sound to work in the past, but maybe its an issue with alsa. I'm also thinking that I probably might have given up and installed Pulseaudio as a result.
Will keep researching that another time but I'm making it a mission to not give up and install Pulseaudio since I'd prefer just straight Alsa audio instead.
Openbox on the same note is a really cool and great lightweight window manager: http://openbox.org/wiki/Main_Page
The idea is that if you like Windoze, just use Openbox on a Linux distribution, and kiss Microsoft goodbye. Openbox offers the same type of window experience in terms of dragging windows around manually.
Plus you can customize the window themes, and config files easily as well, so there's really no reason to keep Windoze, other than maybe for "muh games". Even that reason is dumb given that Steam Proton is getting pretty good for keeping up with Steam games.
More and more, if I wanted to play games, I'd prefer just emulating things, and would rather even get PC centered ports on Switch if I really wanted to play any new gen games.
Games in general are fun from time to time, but PC gaming as an industry and as a hardware market have really gone downhill in my opinion on a lot of factors.
Why keep up with hardware cost constraints when you've got cryptocurrency miners just completely ruining the hardware market in terms of video card costs. If you've ever shopped for a somewhat OK video card, you'll see how ridiculous its become. Now its to the point where you should just build an OK desktop, run Linux on it, or even just get an old Thinkpad to run some minimial Linux distro on it. Better yet, even take an old computer, run some stable Debian based minimal distribution (without Systemd :)) and you're golden.
Either way, pretty happy at the moment as I've conquered a few tasks this year so far that were really bothering me which included getting rid of my GitHub, and getting a FastMail email account to move away from evil proprietary Microsoft Outlook.
Richard Stallman was right in that if the product is free (MS Outlook), YOU are the product. So, why give Microsoft free money from the obvious telemetry that they're doing on my account when I could just be on a more secure and freer platform. I'll try out FastMail for a year, and if it sucks, I'll find a different solution (or maybe host my own on a VPS instead).
Looking forward to possibly improving the design of this site's images section, maybe the styling on this Blog portion of the site with a sample Bootstrap theme by modifying the "org-twbs-export-to-html" function's "org-twbs-head" function: https://github.com/marsmining/ox-twbs/blob/d5ae9c3fb224d081d59d3686d619edf152523f09/ox-twbs.el#L987-L1002
On another note, for better work productivity, I figured out how to rip a current ZenDesk ticket tab's information so that I can copy the entire ticket information into a ZenDesk TODO list item onto my clipboard.
I'm trying to figure out how to use the "GreaseMonkey" extension so that I can just run this on the fly as well, so that's been a new good challenge for work.
Other than that, I've been learning more C from the related second edition of the book, "The C Programming Language". C is definitely interesting, and I can see portions of it were used for Python as some of the syntax carries over.
I'll be interested in what projects I can create with C later on, but I'm positive that the possibilities are really endless as C is basically on most platforms anyway. Combined with the 'ncurses' interface library, I'll be making terminal apps in no time, so I'm curious on how far I'll be able to create some pet project C based apps this year.
I'm debating using either Neomutt in tmux for terminal based e-mail or give into using Emacs based email with 'mu4e'. Both options are cool but have their advantages and disadvantages. Mostly, both routes are pretty hard, but I'm leaning towards Neomutt, as I've liked using it before using Luke Smith's "Mutt Wizard" scripts. However, the last time I used his installation scripts, the GPG keys weren't working correctly.
Basically, terminal based email involves the following items as far as what I've researched:
- Sync based utility to sync mail with IMAP
- Utility to send mail via SMTP protocol
- Utility to encrypt emails with GPG keys
I'm definitely curious on how to do this process myself, but know that it will probably take a good majority of a weekend day to figure it out to get it up and running based on the Neomutt manual alone or something.
We'll see what I pick soon I guess :)
Stay safe, God Bless.
June 8, 2020
I completely removed my GitHub account as I want nothing to do with Microsoft going forward.
I think GitHub isn't that great of a service and feel that I do better off with hosting my own projects as just .git directories on my own web page.
If anyone is really that interested in obtaining a repo for their own use, then they could just 'wget' the contents of the page and be done with it.
Pretty happy about this move though.
Now I just have to move all of my personal project repos to their own git instances on the public facing portion of my website, and we'll be good.
One step closer without any dependence upon Microsoft. Today is a good day.
Now to think about how to do this on the work computer to run Linux instead ;) and maybe even LegacyOS to match, ha! That'll be the day.
A day… coming soon :)
June 7, 2020
I re-did my Linux laptop with Devuan instead of Debian min installer.
It went well except I had to follow this post to fix the sound issue present with alsamixer: https://dev.to/setevoy/linux-alsa-lib-pcmdmixc1108sndpcmdmixopen-unable-to-open-slave-38on
Basically, I just had to add a "default.conf" file in the etc/modprobe.d directory.
Devuan is basically Debian without 'systemd'. 'systemd' is pretty controversial as it is an init system that could be potentially used as a backdoor.
I'd say as long as I have the freedom to install whatever I want on my computer, I'd rather opt out of systemd completely, so I did.
Devuan can be found here: https://devuan.org/
It was just as easy to install as Debian minimal stable net installer, so I highly recommend it the sound issue was the only issue I've had so far, but easily fixed it with the forum post link above.
The only other major issue was the pain of having to create a new SSH key by hand since DigitalOcean's dumb website didn't work nicely with Qutebrowser (most likely because they have a ton of ad-trackers that were blocked by default with Qutebrowser). Might pick a different VPS with an easier website later on as a result if I can find one. Let's just say I don't want to have to literally type in an SSH key by hand ever again any time soon to just re-gain SSH access to my site.
Next, I'd like to then look into buying a used Thinkpad X60 or X200 laptop to do a software based libreboot so I don't have to solder a Raspberry Pi onto the laptop itself to remove the proprietary BIOS from it.
However, it looks like people on eBay know what they're selling so I'll have to keep my eye out for a good deal. Might even have to get one without a hard drive, but this is fine since I'm ok with even HDD's as I think SSD's are kind of just ok to be honest.
In terms of learning Linux based utilities, I want to definitely take a look a learning the "coreutils" found here: https://www.gnu.org/software/coreutils/faq/coreutils-faq.html
Along with core utils, learning C has been cool, and Bash looks like its gonna be fun to learn. Definitely want to check out the 'ncurses' library as well for good ideas of terminal apps to make.
I'm not sure what I'm going to do with my desktop machine.
I'm debating to just nuke the Arch installation since that ALSO has 'systemd'. If anything, Steam probably has a ton of telemetry anyway, and those games aren't really that essential anyway.
It would better to just run emulators if I want to run games anyway, however I might change my mind to just keep a games based drive anyway. Might just do Manjaro without 'systemd' for the games based based SSD drive instead.
Here's a list of things I'd like to get done sometime in the next few weeks:
Task | Description |
1. Move over all of my GitHub repos to a public facing one on the Musimatic.net page | Could just do this with a public .git instance |
2. Figure out Neomutt with isync and msmtp for my new Fastmail email account | Might give up and just use Luke Smith's mutt wizard but we'll see |
3. Fix the images page on my website | Doesn't even work for the art I made |
4. Install Manjaro or another Arch derivative on the games based SSD drive | Would be good to replace my Arch install since I have no desire to use Arch now |
On another note, I've been going through 2 Samuel in terms of bible reading, and have learned a lot. Let's just say people in biblical times have had more difficult decisions to make, so it puts any of the dumb stresses of modern life into perspective and makes them kind of laughably meaningless in a good way.
Keeping positive despite the times, and am still having a blast learning Linux based stuff along the way.
Hoping for a good house setup later with a homestead to match, but one day at a time.
God Bless.
June 2, 2020
I'll be working on one of these topics tomorrow:
TODO List Item | Reason |
1. Make the images part of this site actually useable | Because it could look better |
2. Migrate all my repos off GitHub and onto public ones on this site, and be done with Microsoft once and for all | Because life could be even that much better |
3. Install Devuan in a virtual machine on my laptop, take notes, and replace all Debian drives with Devuan | Because 'systemd' is evil |
4. Debate a few Thinkpads to libreboot (Thinkpad X400s, Thinkpad X200, Thinkpad X220) | Because Richard Stallman was right |
5. Keep researching best ways to make a small homestead | Because God will provide for my family when its needed |
6. Learn some cool Linux based apps to use in both 'x' and in TTY Linux terminals | Because minimalism always is good |
7. Keep learning C, while looking ahead in the book chapters itself / look into ncurses documentation | Because C is an awesome programming language |
8. Keep figuring out ways to make my worklife more manageable with Emacs Org Mode | Because its been a huge help in productivity |
9. Keep reading the good word with 'KJV' | Because God's Will is the best plan |
God Bless.
June 1, 2020
After much deliberation, I was finally able to get "youtube-dl" to work with Udemy.
Udemy is basically a site where you can take online video courses.
I combined a few ideas but here's what you have to do to download Udemy videos using 'cookie extractor' and 'youtube-dl':
- You have to first provide a web browser cookie that contains your previous login information.
This means that you'll have to literally use a web browser of your choice, login to the Udemy website, and close out of the browser.
- You'll then need to use a cookie extractor to finish the job.
I used this one to manually do the job: https://github.com/jdallien/cookie_extractor
There are web extensions for other browsers like Google Chrome or Mozilla Firefox as well if needed that do the same thing.
- Once 'cookie extractor' is installed successfully, you then have to point cookie extractor to the correct location.
I've been using 'qutebrowser' as my web browser, so my cookies are located in my home directory: ~/.local/share/qutebrowser/webengine/Cookies
This is the command I ran to export the 'cookies.txt' file successfully, and save it to the ~/Downloads directory: cookieextractor ~/.local/share/qutebrowser/webengine/Cookies > ~/Downloads/cookies.txt
- Then, read this 'youtube-dl' GitHub issue for more information as there's a pretty good guide in the 'Valid Cookie File':
https://github.com/ytdl-org/youtube-dl/issues/4539
- Here's an example command you need to provide to youtube-dl that
I personally used to obtain the .mp4 video of the lecture I was on, which discussed 'Heroku' which is basically a way to host your own apps in a cloud environment:
youtube-dl https://www.udemy.com/the-complete-web-development-bootcamp –cookies ~/Downloads/cookies.txt –playlist-items "184" -o '%(playlist)s/%(chapter_number)s. %(chapter)s/%(playlist_index)s. %(title)s.%(ext)s'
And there you have it.
I can finally watch course videos on my own time without the need of streaming video players for online Udemy courses I already paid for.
Why waste the bandwidth, when you can just download it once, and never deal with buffering videos again?
'youtube-dl' rules, and this proves it.
Will keep learning 'mpv' and other related tools to make some cool scripts to share. Stay tuned.
God Bless.
May 31, 2020
I finally got AwesomeWM to display terminal windows with gaps, with the help of this example AwesomeWM config I found: https://pastebin.com/yTHUmDeL
This is mentioned in the Arch Wiki, however, its not too clear where exactly the "Theme" section begins: https://wiki.archlinux.org/index.php/Awesome
Here's a screenshot to show the "beautiful.useless_gap = 5" setting (which I was able to do via Emacs with C-u C-c C-l):

This is good since I was pretty dang close to just finding a new window manager if I couldn't figure out gaps easily.
However, the more I learned about 'systemd', the more I realized I need to get all Linux computers switched to distros without it for optimization reasons.
With this in mind, I'm debating Gentoo: https://www.gentoo.org/
vs Slackware: http://www.slackware.com/
vs Devuan: https://devuan.org/
I'm also debating getting a backup laptop, specifically one that can be "librebooted".
The 'Devuan' operating system listed above flat out is totally against 'systemd' which is awesome, and their reasoning can be found here: https://devuan.org/os/init-freedom
The reason is that if you libreboot a Linux computer, you're able to bypass the default Intel based BIOS that could be spying on you.
Though, normal users like myself don't have anything to hide, that thought alone is kind of dumb to not at least put the power back into your own hands. Its just better for peace of mind to just use free and open software overall.
Best quote on this topic is from Richard Stallman himself: "To be able to choose between proprietary software packages is to be able to choose your master. Freedom means not having a master."
Richard Stallman's computer habits on his Thinkpad T400s can be found here: https://stallman.org/stallman-computing.html
Of course, though Internet Service Providers are likely to do the same dang thing anyway, I would still like the ability to run FOSS (free as in freedom) software in whatever manner I'd like to, and not on the terms of a Linux distribution like Debian that forces 'systemd' on its users.
Makes me want to get a Thinkpad T400s off eBay to libreboot.
A great resource for this topic for anyone considering to throw their Windows infested computers into the trash, and librebooting it with GNU/Linux is this site I found: https://www.thinkwiki.org/wiki/ThinkWiki
I do however like Debian a lot for its stability so 'Deuvan' seems like the best choice as its basically a fork of Debian without 'systemd'.
In terms of the last week off due to vacation, I'm glad to have been able to complete a lot of things last week in terms of minor things for my Linux laptop.
With that in mind, I plan on just skimming the rest of that course, and finish up the one JS based book to kind of finish my learning stuff for work.
After that, I plan on somehow optimizing my actual job's workflow for Emacs in Windows (or figure out how to dual boot the work computer with Linux and run Windoze as a Virtual Machine :D).
This debate of deciding what is the best OS to pick has kept my mind off the crazier and crazier world we are now living in.
But most of all, I trust in God's Will, since I would like to also get into homesteading one day as well, and need favor on the next couple of decisions for my life.
Looking forward to another month to kick butt at work, and yet another week off at the end of it :).
Stay safe, and God Bless.
May 27, 2020
I was able to install 'pywal' with pip3, which basically allows you to change terminal color schemes on the fly.
Here is the link to 'pywal' on GitHub: https://github.com/dylanaraps/pywal
However, it wasn't necessarily changing my wallpaper as well, so I created a function within my .bashrc config file to change both the color scheme via the 'wal' command AND the wallpaper via 'nitrogen' command:
This can be found in the "# 'pywal' based adjustment" section of my .bashrc: https://github.com/SBanya/dotfiles/tree/master/bash
The result is a bit of a workaround. However, it was pretty neat since it taught me how to obtain the default user input with the "$1" argument.
I'm thinking of ways to maybe incorporate this same idea to possibly give me a color scheme for my "AwesomeWM" (Awesome Window Manager) as well.
Also, I want to learn how to use AwesomeWM with gaps as well.
If I can't get that to work, I might even jump ship and try a completely different window manager.
On another note, the dang Arch drive is having issues powering off again since it just shows a blinking "_" cursor upon logging off from AwesomeWM on the desktop machine.
This most likely is an issue with the video card driver, or the lack of updates.
If I can't really fix it… well, I might just give up and try Slackware instead on the remaining partition, and just re-do the Arch install with Gentoo later on since its been just a dumb uphill battle to get Arch to boot. Using Arch so far on that machine has been a battle of "Will this system actually boot without errors today?".
Maybe I should just use something Debian based but with access to Arch repositories. Who knows.
I'm still glad to have figured out 'pywal' though, cause its so neat.
May 26, 2020
I made improvements to the "Outlook Terminal Client" so that it now has further enhancements to force the user to select correct prompts for the month, day, and year entries.
I also prevented future date entries as well.
I added screenshots and improvements to the README.md as well, which can be found here: https://github.com/SBanya/OutlookTerminalClient/blob/master/OutlookTerminalClient.py
May 25, 2020
I was able to refine the "Outlook Terminal Client" project further, and am pretty much almost done with it.
The only tasks left to work on for this project include:
- Allow user to re-select the already chosen date if they
would like to
- Allow user to select a date before 2020. For whatever reason, it
doesn't allow the user to do this, so this might be an limitation / issue with imap.search() function with the 'SINCE' option.
- Allow user to search for email in mailboxes OTHER than their
'INBOX' folder. Users like myself have heavily filtered folders for their Outlook email, so it would make sense to allow them to select from an integer picklist to do so.
Latest project progress can be found here: https://github.com/SBanya/OutlookTerminalClient/blob/master/OutlookTerminalClient.py
I learned a few things in C which was cool.
Also, had some great food today for Memorial Day and got QTox working.
QTox is a super cool video calling program that runs on most platforms (Windows, Linux, Mac).
I would recommend QTox for anyone interested in Zoom alternatives: https://qtox.github.io/
Jitsi Meet was pretty promising for any smartphone users: https://jitsi.org/
Hope everyone has a great week :) God Bless
May 24, 2020
I'm in the process of relaxing for a bit as I have the week off due to the buildup of one comp day, and I'm piggybacking off Memorial Day weekend.
As such, I have had a bit of time to really adjust my aliases for my .bashrc to allow to for quick two letter commands to open up programs easily in two seconds in Bash terminal, which is pretty cool.
Latest changes to my .bashrc file can be found here: https://github.com/SBanya/dotfiles/blob/master/bash/.bashrc
I also am in the process of swapping out internet browsers to check out IceCat, and then maybe just settle for Qutebrowser instead as well.
As such, I created a parser Python 3 based script that basically takes the "bookmarks.html" file that you can export from Mozilla Firefox and just rips out the "href" attribute into a resulting "links.txt" file so that you can import it into other browsers like "Qutebrowser" or utilities such as "DMenu".
This project can be found here on my GitHub page: https://github.com/SBanya/MozillaFirefoxBookmarkParser
On the topic of GitHub, I've realized that I can simply host a public facing .git instance, so I might actually just move all my projects to this website's public_html folder, and just nuke my entire GitHub profile as well since I'm more of a hobbyist anyway and could care less for proprietary Git vendors like Microsoft.
GitLab is tempting, but it looks like you'd still have to pay to have a free public hosting repository, so I'd rather just host it on my own site anyway.
On the topic of trying out new Linux OS's, I've also thought more and more about it, and I think I'll settle for trying out Slackware on the spare partition I have on my Desktop machine instead of Gentoo.
I'm going to save Gentoo as more of a challenge later on if I get more comfortable using Arch on my Desktop machine.
Currently, my Arch install is broken even though I literally only played a few Steam games on it. Not sure what the deal is with that. However, it is a cool thrill to really have a successful installation of Arch so that's fun in itself and a challenge completed for me.
I really really really like everything Gentoo stands for, but it looks like you have to compile most things to keep the system up to date, which sounds cool at first, but if its basic system level updates, that sounds like a pain to be completely honest, so I think I might save it for a rainy day to try installing it in a VM.
In terms of Bible reading, I've gotten to 1 Samuel - 26:1 so far. I've learned a lot, especially from someone as brave as David to have been chased by his own king (Saul) to his death, and has strengthened by faith a bit more. It really goes to show you that people in older times had it a lot harder, and we should be thankful we don't have to necessarily deal with war on a daily basis. I for one am still thankful for my fiannce, my family, the fact that I have food and shelter, and still have a job in these dark times.
I have a few goals for this week, and they include the following (which utilizes a cool few tricks I learned via Emacs's Org-Mode's Checklist option):
Goals for Memorial Day Vacation Week [10%]
[2/19]
:
- Create Bash aliases for moving dotfiles for w3m, newsboat, bash, etc to dotfiles and doing the commit commands
- Learn and apply Dired Mode Shortcuts to quickly get to ~/programming directory, etc
- Chill Out
- Play retro games on emulator (random SNES, Genesis games, possibly Shining Force CD)
- Play some DOSBox games
- Play some ZX Spectrum Games
- Keep learning C to one day be able to create ncurses interface based programs
- Learn Wallpaper shortcuts with Pywal to make new color schemes
- Backup bookmarks from Firefox, and install Ice Cat on Debian to try it out. Worst case scenario, use Qutebrowser instead.
- Configure Pywal to do a few trigger actions to .Xresources and AwesomeWM:
- Backup the current .Xresources file
- Trigger changes to .Xresources
- Commit changes to .Xresources in ~/programming/dotfiles/Xresources
- Backup the current AwesomeWM config theme
- Change AwesomeWM color scheme to reflect changes from Pywal as well
- Commit changes to Awesome color scheme in ~/programming/dotfiles/awesome
- Learn more about Calcurse to make it more useful OR learn Emacs Calendar mode with agendas
- Create a reverse Pywal program that searches for wallpapers based on a given color scheme.
- Learn how to use AwesomeWM with gaps
Have a fun, and safe Memorial Day weekend everyone!
May 20, 2020
I learned a lot with w3m in the past few days but more so today, since I've been able to utilize a few macros from the YouTuber, gotbletu's videos on the topic.
Here's gotbletu's related video on the topic of advanced macros in w3m browser: https://www.youtube.com/watch?v=lL73xWsaJP8&list=PLqv94xWU9zZ35Yv0s6zMID5JoS8qu19Kh&index=10
Here are some example commands that I added to my 'keymap' file in my ~/.w3m/ directory so that I can easily do some w3m commands with ease, the last of which I came up on my own to toggle the 'color' command in w3m to allow for color to be displayed on a page:
keymap \\\t COMMAND "SET_OPTION display_borders=toggle ; RESHAPE" keymap \\\i COMMAND "SET_OPTION display_image=toggle ; RELOAD" keymap \\\e COMMAND "SET_OPTION user_agent='' ; RELOAD" keymap \\\a COMMAND "SET_OPTION user_agent='Opera/9.80 (S60; SymbOS; Opera Mobi/SYB-1107071606; U; en) Presto/2.8.149 Version/11.10' ; RELOAD" keymap \\\c COMMAND "SET_OPTION color=toggle ; RELOAD"
May 19, 2020
Over the past few days, I figured out how to use YouTube without using YouTube, which has been the coolest and best breakthrough ever.
Enter Indivio.us: "https://invidio.us/"
It's basically a website which you can run with a JavaScript-less browser like w3m which allows you to search YouTube without ever be thrown a targeted ad, nor be given any mention of any forced suggested videos.
What you can do is to literally pipe the link you're currently on, and then output it to mpv on a separate shell window.
This basically allows you to stream any YouTube video you want with mpv, again WITHOUT USING YOUTUBE.
This is totally awesome, and just helps overall as YouTube has been crazy with too much targeted content lately.
I'm just the kind of person who wants to be on their site for Linux info, music, and that's about it. Other than that, could care less about any other content period.
That being said, give it a shot. There's a really good video by the YouTube user, 'gotbletu', that goes over how to add links to Linux clipboard in 'w3m' which I highly recommend.
Here's the video from 'gotbletu' on w3m external commands: https://www.youtube.com/watch?v=YzgCgarUa_M&list=PLqv94xWU9zZ35Yv0s6zMID5JoS8qu19Kh&index=5
I now have remapped the "EXTERN" function in w3m to be the 'e' key, and also remapped the "EXTERN_LINK" function in w3m to be the 'f' key.
Combined with the two separate macros from the video from 'gotbletu', I can easily copy the current URL link (EXTERN) to the clipboard OR the one I'm hovering over (EXTERN_LINK), so that I can pipe it into mpv to stream YouTube videos without ever involving YouTube.
Here are my current modifications to the 'keymap' file in ~/.w3m:
code
########################
## CUSTOM KEYBINDINGS ##
########################
keymap e EXTERN
keymap f EXTERN_LINK
code
Also, you can still output your YouTube Subscriptions into an OPML XML file to be imported by an RSS feed reader like Newsboat here: https://www.youtube.com/subscription_manager/
It took a while as it seems YouTube hid this feature, but alas I found it, and was super happy I did.
You can basically download this file, and 'cd' to your Downloads directory, and run the following command to import it into Newsboat: newsboat -i (name of file)
Also, here is the more example of a YouTube based OPML XML link as well for a given YouTube channel which exposes some possibilities for cool scripting possibilities as you can add any of the XML tags as '&' parameters for the URL itself: https://www.youtube.com/feeds/videos.xml?channel_id=UC2eYFnH61tmytImy1mTYvhA
Example: From that example above, you can see the "<yt:channelId>" tag present.
This means that you can add the 'channel_id' as a parameter to the base URL pattern: https://www.youtube.com/feeds/videos.xml?
BECOMES:
https://www.youtube.com/feeds/videos.xml?channel_id=UC2eYFnH61tmytImy1mTYvhA
This allows you to specifically look throughout a YouTube user's videos, and would allow you to create a OPML file yourself for YouTube if you needed to.
The More You Know :)
Also, I totally want to learn 'pywal' which allows you to change your terminal's color scheme based on your current wallpaper background: https://github.com/dylanaraps/pywal
Plus, I installed 'qtox' so that I can get into video chats as well, so I'm pretty stoked about that as well.
More stuff to learn this week ^_^
May 18, 2020
I was able to modify my Newsboat configuration so that I could use a macro to open up YouTube videos with "',' (Comma) + m" which opens up the video in MPV.
I also made a revision to do the same action but instead be able to open up the same article in Firefox with "',' (Comma) + f" as well.
How this macro basically works:
- It utilizes the "macro" key to set a macro in w3m.
- It then uses 'set browser' to change the browser to the
desired program, which in this case is 'mpv' or 'firefox' respectively.
- It then calls the 'open-in-browser' command to open up the article
(or YouTube video link in this case) in the desired program.
- It then sets my default 'browser' environment variable in Newsboat
back to w3m, as this is my desired default browser.
This means you can literally look at YouTube videos from the accounts you're subscribed to via RSS, and not even be on YouTube to view a single video.
This means you can access YouTube content without ads or any other unrelated content, which is super awesome.
Here's where you can find my current Newsboat config: https://github.com/SBanya/dotfiles/tree/master/newsboat
Here are the modifications I made to my "config" file in my ~/.newsboat directory:
macro m set browser "/usr/bin/mpv %u"; open-in-browser ; set browser "/usr/bin/w3m %u"
macro f set browser "/usr/bin/firefox %u"; open-in-browser ; set browser "/usr/bin/w3m %u"
May 16, 2020
I started work on a new Selenium based Python script project as I find it pretty frustrating how YouTube has elminated the ability to export your Subscriptions as an OPML file anymore.
I believe they are doing this purely for the fact that there's YouTube Premium now, and they want to squeeze as much ad revenue as possible by forcing people onto their site to view content.
However, the lack of being able to export your Subscription feed into an OPML XML file doesn't help anyone at all who accesses their site via Screen Readers who happen to use (ex: blind people who can't see video content but want to keep up with the audio versions of their Subscription content on YouTube purely on text based titles for videos).
This project aims to solve this issue by logging into a user's YouTube account via Selenium with Python bindings + the Firefox web driver so that the user can rip the list of the YouTube channels into a list to then later provide to a RSS reader (ex: Newsboat, etc): https://github.com/SBanya/YoutubeSubscriptionList/
I'm not finished with it by any means yet, but am getting close but I'm running into a few issues with the Selenium driver itself as I had to obtain the latest Gecko driver here, and had to un-tar it to a specific location on my harddrive: https://github.com/mozilla/geckodriver/releases
I also learned how to use w3m more efficiently, especially with a lot of the options enabled within the 'o' menu to quickly refer to each line with 'g' + line number.
I also learned how to use w3mman which is a way to put a man page within w3m which is useful since most man pages have external links at the bottom for documentation references.
Using w3m alone is pretty sweet.
However, I would love to have my entire Tmux session to be based from Newsboat with w3m and other CLI utilities, so its been fun incorporating these tools into a terminal only workflow.
May 14, 2020
I've had a pretty easy going week at work this week, which led me to finish a lot of work projects in the next few weeks ahead of time in terms of a week off of vacation.
That being noted, I've also made strides to really see if I can attempt to tap into ZenDesk. I've come across a few barriers including the fact that I don't have access to the ZenDesk API for work. However, I'm working around this by utilizing Selenium with Python 3 in an effort to convert a list of tickets and import them into an Org mode document so that I would automatically have a todo list of recently updated tickets.
This would help my workflow, and might help others in my situation in which the API access is limited to someone's ZenDesk account. Also, I use Org-Mode quite a lot with TODO lists at work, so incorporating the current status of tickets into my workflow would be awesome. Even possibly having the ability to respond to tickets without even having to log into ZenDesk sounds awesome. I can probably achieve this with Selenium through either ZenDesk or my work email, so either of these two solutions will help me achieve my goal in that regards.
I have been learning more about the w3m browser, which is a super cool terminal based web browser. Using it with the w3m-img add-on is a must though as this allows you to display images while surfing the web in your terminal.
I also recommend using a terminal like urxvt or x-term which supports w3m-img.
More info. on w3m can be found here: http://w3m.sourceforge.net/
I've been playing quite a few ZX Spectrum games, and got X128, a DOS based pemulator for the ZX Spectrum to work just fine. However, I can't seem to figure out why mapping keys is so hard for that emulator. I also installed the FUSE emulator through apt so we'll see if that's better in the long run.
Games I'm Debating To Play This Weekend:
Game | Reason |
---|---|
Dragon Warrior 3 (Gameboy Color) | Awesome music, great art style, great gameplay. |
Mount & Blade: Warband | Seems like a cool game to play |
The Elder Scrolls IV: Oblivion | Wanted to start a new character and just do whatever I want in this game. Fun game for sure. |
Things I'm Debating Doing This Weekend:
Thing To Do | Reason |
---|---|
Decide if I should install Tox, Jitsi, or Jami for video calls | Pretty awesome FOSS apps to use to call family. Beats spyware infested Skype or Zoom |
Do some ink drawings with scans into my computer | Would like to get more into doing more litho style art |
Learn more C | I find learning C to be fun, and the opportunities to modify Linux utilities are endless |
Possibly working on a Dungeon Synth album | A lot of the songs I've been making have been themes, this genre seems to fit pretty well |
Look into a decent image gallery for my art pics using a Bootstrap template | I'll take a look around to see if there's a better way to display my images |
Possibly learn Aria2 for downloading files | Seems like a cool CLI utility |
Possibly learn how to use Alpine Email client | Seems pretty straight forward for my purposes, looks easier than "mu" / "mu4e" |
Possibly modify my AwesomeWM config | Wanted to explore other functions to tap into |
Possibly modify my Tmux config | Wanted to see what else I haven't tapped into yet since I want to use CLI only Gentoo |
Possibly porting some projects from the Web Dev course | Wanted to see if I could run self-contained web apps on my site |
Figure out how to utilize Youtube-dl to access YT without being on the site | Would rather just stream the content I want to watch than deal with ads |
May 10, 2020
I worked on using the "SC-IM" program to manage my yearly finances and expenses.
"sc-im" which stands for "sc-improved" which is basically a spreadsheet program that utilizes Vim bindings: https://github.com/andmarti1424/sc-im
Two really good videos on how to use "sc-im" which I have found to be super useful include:
Luke Smith's video on SC-IM: https://www.youtube.com/watch?v=K_8_gazN7h0&t=19s
gotbletu's video on "sc", which is the predecessor to "SC-IM" which covers some of the formula usage in "sc" which is pretty neat and not necessarily too intuitive in the man page for "sc-im": https://www.youtube.com/watch?v=xrX2isHDQu8
With this knowledge, I've been able to make a master spreadsheet containing my finances for the year.
It took A LOT of manipulation from both the credit card and checking account based .csv's, but hey, it can be done.
It's pretty neat because by doing this, you can take charge of your finances and determine how much you're spending month to month.
Some Useful Keybindings For "sc-im" That You Might Find Useful:
Command | Command Desc. | Example | Example Desc. |
g(cell number) | Go to cell number | ga0 | Go to cell, a0 |
dc | Delete column | dc | Delete the current column |
dr | Delete row | dr | Delete the current row |
// (This is an alias for :int goto | Search for a particular item | :int goto "ONLINE" | Search for the "ONLINE" string |
n | Search for the next search instance | n | Search for the next instance of the "ONLINE" string |
:format "(desired format)" | Allows you to format a cell range | :format "-#.00" | Format the current cell to a negative number with two decimals |
yc | Yank the current column | yc | Yank the current column |
p | Paste the previously yanked item | p | Paste the previously yanked item |
:e! csv | Export the current spreadsheet into a csv of the same name | :e! csv | Export the current spreadsheet into a csv of the same name |
:sort (range) "(+ or -)(# or $)(Col Letter) | Allows you to sort a range of cells in asc(+) or desc(-) order based on a num (#) or str($) value) for a column | :sort A1:C48 "+$A" | Sort the range, A1:C48 in ascending order based on a string value |
G | Go to the last cell in the lower right corner | G | Go to the last cell in the lower right corner |
Once you have formatted both the credit card and checking account .csvs to the desired column formats, you can utilize the 'cat' Linux command to combine them and re-direct them to a single file.
For example, this command would take the credit card .csv and checking account .csv files and combine them into one master .csv file:
cat creditCard.csv checkingAccount.csv >> masterFile.csv
I keep it simple however, so its not as granular as something like a Credit Card statement that breaks down "food" vs "expenses", etc.
However, I have the description fields intact within its own designated column, so I can check out line items if I want to for specific expenses.
To help anyone else who might be utilizing a Wells Fargo bank account, I created a webscraper with the Firefox Selenium webdriver.
You will need to have "pip3" installed Selenium, as well as "getpass" Python 3 libraries to utilize this Python 3 based webscraper script.
It aims to obtain the user's latest balance to copy it into a file of the user's choosing, or onto the clipboard (still deciding what would be the best for my particular workflow since I currently just need it to insert into a related .csv file, but most Linux users would probably want to just add it to their clipboard using x-clip).
My current "Wells Fargo Balance Webscraper" project can be found here: https://github.com/SBanya/WellsFargoBalanceWebscraper
I also played a bunch of games from the "bsdgames" repo from Debian's repository: https://packages.debian.org/stable/games/bsdgames
Also, I checked out a few things from this cool older terminal program based blog: https://inconsolation.wordpress.com/
I highly recommend this blog if you're getting into minimalistic terminal program software since a few things stand out as cool ideas to add to a computer workflow for the newer or medium level Linux user.
Debating what DOS games to play this weekend, and also I would like to un-break my Arch install on the desktop computer that I have Steam on since I'd like to keep messing around with it to see what games Linux can run with purely Steam, and also might try to load a few emulators on that same OS as well.
Also debating whether or not to put Gentoo on the other half of the same drive, since I really really really like their philosophy and take on free software (free as in "freedom").
My Goals For The Upcoming Weeks Include:
Goal | Description |
---|---|
Contribute to Christian based FOSS software | Attempting to do so with Xiphos, but might find a different project |
Create theme based music using MuseScore | Self explanatory |
Learn gardening techniques | Would want to become more self-sufficient |
Learn soldering techniques | Will need soldering equipment but wanted to learn basic electronics |
Learn Alpine Email Client OR mu4e for Emacs | Would like to give this a shot as I'm sick of using Outlook in browser |
Host my own email address either through VM or pay-for-service | Sick of MS Outlook email, and want to change to a new email address |
Figure out how to use cURL for ZenDesk for work | Would rather do all my work in Emacs, but without the API, this might be the only way |
Learn Org-Mode basics for calendaring and agenda | Would want to explore these options for personal use |
Learn Org-Capture Mode | Need to develop better templates for work |
Create a macro to copy today's TODO list to tomorrow's directory for work | It's too manual at this point, and should be automated. |
Learn more w3m browser | Would want to become less dependent on GUI based browsers |
Learn ImageMagick | Reason being is that there are really cool ways to manipulate images via terminal |
Install Gentoo | I would like to attempt to purely use terminal apps using this distro |
Possibly play Dwarf Fortress or Mount & Blade: Warband | Always wanted to play these games |
Develop useful web apps that blend Python3 and NodeJS | Would like to make useful standalone web apps on my site beneficial for normal people's use |
My best advice for these days is to pretty much keep the motto, "God's will".
That's been helping me a lot mentally in these times.
Living for today, and enjoy the blessings that you have each day.
Hope everyone is doing well, God Bless.
May 5, 2020
I am happy to note that I have successfully created the Scripture Of The Day project.
My resulting output webpage from this project can be found here: http://www.musimatic.net/pythonprojectwebsites/ScriptureOfTheDay/output.html
Basically, this takes a random verse from the King James Version of the Bible using the 'kjv' terminal program, which can be found here and built from source: https://github.com/bontibon/kjv
It is updated daily via a cronjob.
Very happy to have been able to complete this successfully.
Looking forward to continuing progress in my other project, "OutlookTerminalClient", as I wanted to make a project to easily display current emails from Outlook.
After that, I will continue to explore other programs that morph Python 3 and JS based web frameworks to create interested web applications.
Also, I want to explore more terminal based applications to minmize the amount of bloat on my system.
I am also debating what should be my long-term email application to use, and am debating between the following options:
Email Terminal Program Name | Pros | Cons |
mu / mu4e (mu for emacs) | Seems light-weight | No 'ncurses' interface |
alpine | Seems easy to use | None that I can see so far |
OfflineIMAP | Stores emails on local computer | I'm a bit hesitant to store emails locally, but most Linux enthusiasts prefer this route |
May 4, 2020
I forgot to post this the other day, but I did make one final album for my Shibes band here: https://shibes.bandcamp.com/album/perspectives
Unfortunately, with all my attempts to re-arrange my Linux harddrives the other weekend, I actually erased the contents of the entire album, so I only have the final exported music files as remnants of those sessions. This is fine since I'm more of a person that just prefers to get stuff out there, then to dwell on stuff and never release anything.
However, this does mean I can never re-master that album, but that's okay.
I'm kind of done with that band as it represents a certain era of my life that I'd kind of like to leave behind a bit. I'm aiming to be more positive despite the crazy times we're living in, and dwelling on negatives isn't really my vibe anymore, even if it was partly for slight joke vibes.
I plan on making music going forward, but moreso, probably theme type music with tools like MuseScore.
I would like to learn more songs on keyboard, mostly game themes, and also a few harder songs as well.
I plan on also learning Ardour after maybe caving into the $35 license fee.
Another thing I've been working on is utilizing more terminal based programs.
One such program is "sc-im" which is a Vim based terminal spreadsheet program: https://github.com/andmarti1424/sc-im
Very cool so far.
Also, I plan on checking out way more terminal based programs from this discontinued blog to add them to my array of tools to use: https://inconsolation.wordpress.com/
I'm currently debating either Slackware or Gentoo for a purely terminal based distribution on a spare portion of my other harddrive as well on the desktop computer, which is going to be fun. I don't mind window managers with some GUI applications, but less bloat always leads to more productivity so I'm all for it.
May 3, 2020
I'm glad that I now have access to my own website again so I can freely post as I please again.
Clearly, the world has changed quite a bit in the last few months.
To stay positive, I've been re-affirming my Christian faith, so that's been a focus of mine.
I've been reading through the Bible with the help of 'KJV', which is a really neat terminal based Bible program: https://github.com/bontibon/kjv
A really cool quote amidst these crazy times that's pretty inspiring is from The Lord Of The Rings - The Fellowship of the Ring from J.R.R. Tolkien.
To give a synopsis, Frodo and Gandalf are discussing the complications involving the ring he received from Bilbo, and the very real possibility that he will be hunted by Sauron for having the ring in the first place.
Here is the quoted conversation from Frodo and Gandalf from the book:
“I wish it need not have happened in my time," said Frodo. "So do I," said Gandalf, "and so do all who live to see such times. But that is not for them to decide. All we have to decide is what to do with the time that is given us.”
With that in mind, time is pretty precious to someone like me with a full time job.
As a result, I've used Emacs Org-Mode for my actual job and my own personal life to manage how much time I've spent on tasks, and on skills.
It's really helped me a lot, so I whole-heartedly recommend giving Emacs and Org-Mode a try if you never considered adding "TODO" lists in your daily routine.
Links: https://www.gnu.org/software/emacs/ https://orgmode.org/
Also, I've been reading up on possibly growing my own food as well in case I'd like a self-sustainable house later on in my life. That being said, homesteading looks like a cool hobby on the side.
To stay positive amidst these times, I have re-vamped my current Linux computer setups, and have re-done several of my computers to try out a few things.
I am currently running Debian minimal installation on both computers, and have been trying out Arch Linux along with Steam to get some games working without ever needing Windows 10.
Pretty sweet so far, and am loving it.
I learned a lot, but let's just say the Arch install was pretty dang hard.
And to say the least, I totally wiped out my one hard-drive by accident as a result. Let's just say that even if you accidentally delete a harddrive's partition, doesn't mean you should use the "dd" command to wipe the harddrive afterwards since you can easily just re-create the partitions afterwards.
I learned this lesson the hard way.
I plan on picking another Linux distro to run on the same drive but with purely terminal based apps without ever using XOrg.
I also have been trying to get in touch with the "Xiphos" team, which helps maintain this cool bible study program, but haven't really heard back from them in their mailing list, so I might have to keep searching for open source projects to possibly contribute to.
My current Linux based goals include:
- Learn Ledger or other CLI tools to monitor finances with .csvs from bank statements
- Possibly learn sc-vim instead of Ledger, since it might be easier with just a master .csv file for all finances
My current programming goals:
- Continue to learn web development through the Udemy course
- Make Node.JS apps that blend with Python 3 as well
- Keep looking for Christian faith based open source projects to contribute to
My current pet programming projects include:
- Goal: Generates a random King James Version of the Bible
- scripture of the day, and displays it on a webpage by using the 'KJV'
- command line utility.
- Link:
- https://github.com/SBanya/ScriptureOfTheDay
- Goal: This project aims to be a simpler approach to
- read emails from an MS Outlook email account directly from your Linux terminal!
- Link:
- https://github.com/SBanya/OutlookTerminalClient
Overall, I hope everyone is safe, and wish the best. God bless.
January 14, 2020
I completed the Bandcamper project which can be found here: http://www.musimatic.net/pythonprojectwebsites/Bandcamper/tags.html
January 9, 2020
I redesigned the entire site, and am writing this blog using Emacs's Org-Mode
November 9, 2019
Here's a pretty useful command if you'd like to search for programs on the command line within a Debian based Linux distro:
apt-cache search (pattern or name of program you're interested in): This allows you to search for programs on the command line to install.
Here's an example of how to apply this idea in action. In this example, let's search for some terminal emulator programs on Debian's apt package manager by simply providing "terminal emulator" as the pattern:
apt-cache search terminal emulator
Here's a screenshot of Sakura terminal with tmux running that command: <img id="IxAptSearchTerminalScreenshot" src="images/aptsearchterminalscreenshot.png"/>
As you can see, this is pretty powerful, especially if you know a few things about pattern matching with grep commands. Try using this idea to try some new programs out!
November 6, 2019
I finally got the Gifs page to work with the necessary file permissions, so go check it out. These are the gifs I've made for the past few years using a few apps on an old Samsung Galaxy S6 phone I've had. Definitely would like to continue making art in this style with the use of the program, GIMP, alongside some scripts to help automate it as creating gifs can be a tedious but fun process.
The Gifs webpage can be found here:
November 4, 2019
In case you ever need to indent an entire webpage (just like this one!) in Emacs, you can actually select all of the code present with:
C-x-f (Ctrl+x+f): This allows you to select all lines of code present in a file.
You can then finally then indent everything with:
C-M-\ (Ctrl + Alt + \ 'backslash'): This indents all lines of code accordingly.
I found out this trick the other day, and it helped so much especially on a site like this when I use multiple programming languages like Python 3 vs. JS vs HTML+CSS. By letting Emacs do the work to beautify the code, it makes the process of creating projects that much easier.
November 3, 2019
I am planning to do a re-design in early 2020 for this site to be more minimalistic in design as I think the style attempt is good, but it could be a bit simpler, and be based on more templated designs. I'll figure out how to do this using either purely templates, or purely JS.
I am really looking forward to working on the Bandcamper project soon. This project's GitHub page can be found here:
The goal of this project is to perform the following actions:
- Scrape the Bandcamp tags page for the latest geographical tags (ex: US states) and genres
- Display a webpage to the user to prompt them to ask what genre or geographical state they'd be interested in terms of exploring bands from via a related email.
- Provide the user with the email at 6 AM every day to make the program static and not dependent on being a web app.
- Provide the user with the ability to opt out of an email every time.
- Limit messages per email to about 10 to prevent spam.
- Provide a user with a script that uses Youtube-dl Linux utility that automatically downloads these Bandcamp music for users.
October 28, 2019
I have finished the Express Or Local App, which is a Python 3 based webscraper runs on a non-stop cron job that pulls MTA 7 Train data and displays it in a nice and easy to read HTML table. I'm glad this project has been finished as it has taken quite a long time to pull off. I've been in the process of making sure my older webscraper projects are working, and have fixed the Hockey Webscraper to make sure it runs daily. Once my projects are back and running, I'm planning to start the Bandcamper Python 3 based project, which is just a daily emailing agent that will email someone their top 5 bands of the day from Bandcamp based on genre or geographical locations they entered via a web request form (and of course they can opt out of it at any time).
Unfortunately, I'm still paying quite a bit of medical bills as a result of that stomach surgery (insurance is a joke) and need to spend on a car soon, so I won't be able to do any maker projects (CNC engraving, synth creation, etc) any time soon but I still am thinking of some cooler projects down the line to do. I am also thinking to possibly review music gear as well as there's a bit of niche territory that's covered by bedroom musicians like myself that aren't really represented on the Internet. I'm also looking forward to making more headway through the "Complete Web Developer Bootcamp" Udemy course I've been slowly working through just so I can improve this site and make better web apps overall. I've kind of realized I still like help-desk type work, and honestly don't care if I end up fully as a developer after all as I've had a lot of fun with these pet projects anyway. Honestly, its what you make out of life, and even with a career, a job is just a job at the end of the day. I think its only when I'll be able to get my side music based instrument business going is when I'll truly be able to push myself for the goals I've wanted to achieve.
I'm also making progress of making new music too, and the keyboard skills have been coming along quite nicely. I'm also debating a complete design overhaul for this site in 2020 to include a way more simpler layout, and washed out colors. Should be fun.
September 2, 2019
I have been healing after a major stomach surgery about a month ago, but have been doing very well in terms of progress. As a test for myself, I put myself up to the challenge of being able to try to tap into GitHub's REST API. Just in case you are wondering, "<strong>REST</strong>" stands for "<strong>RE</strong> presentational<strong>S</strong>tate <strong>T</strong>ransfer", while "<strong>API</strong>" stands for "<strong>A</strong>pplication <strong>P</strong>rogramming <strong>I</strong>nterface". It is basically a way you can access a company's website's data, which in my example is act of me obtaining the company, GitHub's data relating to my GitHub user commits.
In case you'd like to learn about <strong>REST API's</strong>, please check out this really good video on the topic, which is available at this
You can see the related results on the Jumbotron element at the top of my web page, since all my projects are now updated with their latest commit dates and links. This single change is a vast improvement since it makes anyone including future job recruiters more aware of the exact changes I've made to my personal projects, as well as specific code examples if needed. One of a technical job recruiter's first questions is always, "What's your GitHub?". Well, now I can just point them to my web page instead :)
I've also moved away from NYC, and have had a great time so far. I honestly don't miss it since I definitely have spent my time in New York, and would rather return as a tourist for fun whenever I'd like to. I'm looking forward to doing more art projects in terms of lithography, and will actually expand this site to showcase my previous digital art as well since this is my own site after all, and would rather showcase them here than on other platforms. I am also debating how best to make synth projects while in an apartment, and am also debating building a mini-Arduino based CNC in the future.
July 7, 2019
I wanted to share a couple of new Emacs commands I learned while on a plane ride back to New York after a week-long vacation on the West Coast.
m-f: This allows you to go forward one word in emacs.</code>
m-b: This allows you to go backward one word in emacs.</code>
c-a: This allows you to go to to the beginning of a line in emacs.</code>
c-e: This allows you to go to the end of the current line in emacs.</code>
m-a: This allows you to go back to the beginning of a sentence in emacs.</code>
m-e: This allows you to go to the end of the current sentence in emacs.</code>
c-u: This is the 'unit' command that allows you to do multiples of a command in emacs.</code>
c-u 20 c-f: Ex of using the 'c-u' command in emacs to allow you to go forward 20 characters:
June 25, 2019
I am now updating this website with the power of 'rsync', which is a Linux command line utility that I use to automatically sync the changes from my local computer (laptop) to the Digital Ocean droplet that hosts this site. I have also set this to be done on a Crontab job, meaning that if I ever feel like updating this website, I can do so at my own pace, and it will be automatically be updated every 5 minutes. This was also made possible with the idea of using a 'symlink', which is in the same vein as using a shortcut, but between two file locations in Linux. Here's one link to learn about 'rsync':
Here's another to learn about 'symlink' or the 'ln' command in case you're interested:
A special thank you goes out to people from the #linux channel on IRC that helped me through this process since it was a bit difficult to wrap my head around the process of finally using 'rsync' after days of getting so many permission errors.
The specific command in case you would like to use it on your site is as follows, NOTE: I have used parentheses where YOU need to enter in values:
rsync -e 'ssh' -avzp (path/to/public_html/on/local/machine/with/ending/slash/)
user@(ip address for remote server):/path/to/directory/on/website/machine/with/slash/at/end/
Here's an even more specific example with fake ip address values for context:
rsync -e 'ssh' -avzp /home/user/public_html/ user@555.55.555.5:/home/user/public_html/
One more thing to keep in mind is that you will need to create a symlink between the actual directory that's necessary to host the website on Apache. For me, this was in var/www/musimatic.net/public_html You will need to create symlink with the "ln" command to create a "link" or "shortcut" to the home directory that's easily accessible to any user who has SSH access to the machine hosting your website. This part of the process is done so that rsync can do its syncing ability without having to worry about needing any root access.
June 8, 2019
The Creators of SQL: Donald D. Chamberlin, and Raymond F. Boyce designed SQL while working at IBM to manipulate and retrieve data that was stored in IBM's original sudo-relational database management system, System R, which was developed at IBM's San Jose Research Laboratory in the 1970s.
Reasons To Use SQL: SQL (Structured Query Language) allows you to easily query databases for use in your data projects.
Though there are many variants on DBMS (Database Management System), knowing the basics can allow you to interact with databases regardless of what software you eventually use.
Being able to manipulate queries results in far more powerful results than simply using Excel filters on a dataset in a spreadsheet.
The Basics Of SQL: I've been learning SQL as a result of my job, but also on the side as well to include databases for the fun side projects I have. As a result, I've been able to get a bit more familiar with the basics of how SQL works, so I'd love to impart some of the basics in this post. How To Use Sqlite3 In Linux Bash Shell
Starting Sqlite3 Using An Existing Database Here's the basic syntax on how to open up any Sqlite database using the Linux Bash Shell. First, start up a terminal in your Linux OS, and run the following command after installing Sqlite3 to open up an any database you'd like. The syntax is: sqlite3 (name of database).
Here's an example:
sqlite3 example.db
Running A .sql Script After Loading A SQL Database Now that we've gotten the database loaded, use the '.read' command to open up any SQL script that you currently have present in the same directory. For example, I'm going to load an example script called 'example.sql':
.read example.sql
Your First SQL Select Query Now that we've discussed how to open up Sqlite3 with the Bash shell, let's put this to good use by creating our first query. In this example, I am using the Sqlite database provided by the book, "SamsTeachYourself SQL in 10 Minutes", which can be found here:
Let's begin by opening up the 'tysql.sqlite' database on the Linux Bash Shell in Sqlite3, and then query it directly:
sqlite3 tysql.sql
Now, let's use the 'SELECT' keyword to 'select' some of the data present. More specifically, let's just select all of the data present by using the '*' or 'Wildcard' character in order to select all of it with the following SQL statement. Also, we need to specify what table it is from using the 'FROM' keyword, which we'll make sure to tell SQL to grab data from the 'Products' table.
SELECT * FROM Products;
Our result would look like the following: <img width="85%" height="100%" src="images/SelectStatementResult.jpg"/>
Voilah! You now know how to do the most basic SQL select statement of all using the '*' wildcard character.
Sources
May 26, 2019
A Brief History Of The Emacs Text Editor
Richard Stallman created Emacs in late 1976 after combining the MIT lab 'E' text editor, with a set of macros to make the text editor easier to use.<sup>1</sup>
The Basics Of Emacs: I have begun to learn the text editor, Emacs, with the use of their in-built tutorials. I have always liked Vim, but would like to learn both in case it become useful later for a future Dev or Dev Ops role. If there's something I've liked so far is the capability of using multiple buffers, as well as the idea to use multiple plugins to treat Emacs truly as an all-in-one OS-like application.
Useful Beginner Emacs Shortcuts:
Moving Around In Emacs:
Ctrl + v: Move Forward One Screenful
Meta + v: Move Backward One Screenful
Ctrl + l: Clear The Screen, And Redisplay The Text (Center, Bottom, Top)
Ctrl + n: Next Line
Ctrl + p: Previous Line
Ctrl + f: Forward One Character
Ctrl + b: Previous One Character
Meta + f: Forward One Word
Meta + b: Backward One Word
Saving And Quitting In Emacs:
Ctrl + x; Ctrl + s: Save Your File
Ctrl + x; Ctrl + c: Quit Emacs
Sources:
May 12, 2019
Digital Ocean Now Hosts This Site: I've recently shifted this website to now be hosted on DigitalOcean droplet, which means that I now have total freedom of what gets installed on the backend of the site, and can actively support my existing projects with cooler Linux functionalities, especially with Cron jobs.
I have also been getting the hang of using SFTP to download the site and work on it remotely, and then reupload it when I'm done instead of having to use SSH which disconnects regularly. Express Or Local Python Project: I've been still working on that MTA Python based project, but re-wrote it for the third time to instead utilize the Marshmallow Python library to create database table schemas that end up being used by SQLAlchemyCore.
Bandcamper Python Project: I have also been working on a Python project called Bandcamper, which aims to allow a user to search for artists on Bandcamp in a terminal window without having to use an internet browser. Ultimately, I want the end user to be able to stream the final results in their given music player of choice, and also be able to download any songs they want, with whatever quality they want up to 320 kbps mp3. Past that, they'd have to pay the artist for their music, but this is better than the existing comparable project on Python PyPi that only allows for a measley 128 kbps mp3 download, which is terrible beyond words in terms of music quality.
Fake Website And JavaScript Progress: I've been working on "Emulation Nation", which is just a fake website about emulation that I've been utilizing my JS skills with. I've also been doing the tutorials for Puppeter since I'm planning on porting the webscraper I've created at work to JS with Puppeter for a more responsive webpage experience.
January 19, 2019
This is the Welcome Center I have been developing for the past several months with the use of C++, as well as the Qt framework.
Thankfully, it is now a fully functional one window application that is meant to help guide a new user of Lubuntu of what to do after installing the operating system.
Features of the Welcome Center
- Open The Lubuntu Manual Webpage
- Configure Monitor Settings (Resolution)
- Open LXQt Configuration Center To Further Adjust Entire OS Setup
- Open Lubuntu Community Links Webpage To Get Help Through IRC, Telegram, And Mailing Lists
- Open Up Contribution Guide To Find Out How To Help Contribute Work Towards Lubuntu
January 11, 2019
I have begun the new year with quite a few changes to note. I was unfortunately sick for the last two weeks, but began an awesome new job doing customer support for a software firm. I plan on sharing my adventures in learning automation tools like Selenium, and PowerShell, in order to automate some of the boring or repetitive tasks regarding customer tickets in ZenDesk, and JIRA.
I finally have the Hockey Webscraper up and running after getting the FTP side of things resolved with the web hosting site and am trying my best to still work on finishing the MTA based Express or Local App. I also worked on the Jim Davis based fan website as well.
I am actively working on improving the overall feel of this webpage to be more like WordPress in design, but still am adamant on using my own CSS styling, alongside Bootstrap.
I will use this site as an educational tool in articles that I will call "SuperUser Sam" in which I write about programming, automation, Linux utilities, Raspberry Pi, etc. I am really looking forward to this, and hope you will join me in my love to make everyone's lives a little bit easier with the use of computers.
December 23, 2018
I finished the Garfield Comic Of The Day Webscraper, and posted the results on the site. It will now post on Twitter at 11 AM everyday, and spread the joy of Garfield comics :)
December 13, 2018
I again made little progress to my projects this week due to personal things. I am in the process of making a Garfield themed fake website, and have been actively trying to redo the Python based Express or Local project using an example project I found. I also am learning Ruby on the weekends, and am planning to do a Yelp related project based on restaurants in Sunnyside, Queens.
December 6, 2018
I made small progress this week towards Python and C#, since I've had to deal with web hosting issues this week. I am currently in the process of making a Garfield themed fan website to showcase the skills I have gained from Chapter 4 from the HTML+CSS and JS books. I have also begun learning Ruby on the weekend as well, and updated my GitHub repo with all my progress as of late, but will rehost on Repl.it when I get the chance so that beginners can try out my code on their own as well.
November 30, 2018
I posted on the MTA related Google+ group forum to ask how to hone in on the specific 7 NYC Train data. I also switched my focus on the Bull or Bear Webscraper C# app to try to scrape the CNBC website, and then switched the focus back to trying to utilize the Bloomberg C# API since they DO provide examples within the same download, and its worth a shot. I updated the Python and C# sections with new examples from Chapter 7 and Chapter 2 respectively. I also uploaded all of my progress to my GitHub repository called "scripts", and will upload my C++ progress as Repl.it files as well to be posted on the Programming Projects section as well.
November 21, 2018
I was only able to work on Python this week, but made a bit more progress on the MTA Express or Local App, since I cleaned up a lot of the code into separate functions. The biggest issue I'm facing is trying to make the data only look into the 7 Train, by indexing into feed.entity with a specific value. I've been trying to just brute force it and see which value works, but I might be better off actually looking into some of the .txt files provided by the MTA. I also finished the Visit Monaco website, but am trying to fix the styling of various HTML elements throughout the pages present.
November 16, 2018
I finished applying Chapter 3 of the "Javascript & JQuery" book to the "Visit Monaco" website. I plan on modifying the content by adding additional CSS styling changes next week. I only made small progress for Python and C# due to working overtime to leave early for the Thanksgiving holiday next week. The current issue with the Python based MTA Express or Local App is the looping section for each of the train properties.
I've also decided to get this only working for the 7 Train since Amazon announced its 2nd HQ as Long Island City, Queens, so its only applicable to track the 7 train's data for this reason. The C# based Bloomberg Bull or Bear Webscraper is having issues since I was able to scrape the page using the older "WebClient" C# library, but Bloomberg detected my scraper. This means I'll have to access their data via an API instead, and hopefully this will be easy in C#.
November 9, 2018
I updated the "Visit Monaco" website to include Math and Number JavaScript object specific methods. I also fixed the time module specific issue for the Express or Local MTA Python app, but need to fix the looping issue for each of the stats. I also will follow a YouTube tutorial for the C# based Bloomberg webscraper, since the Iron Webscraper module isn't able to be imported with Repl.it.
November 2, 2018
I updated the "Visit Monaco" website with more object orientated specific methods and properties (String and Number Objects in particular). I also have been working on fixing the time module issue for the MTA Express or Local App. I also have begun learning C# 7.0 within the last two weeks, and posted my latest progress work, as well as a Bloomberg related C# 7.0 webscraper project.
October 26, 2018
I updated the "Visit Monaco" website with more JS based scripts, and also included more of my Python Chapter 7 examples in the Programming Books Progress website progress page. The MTA app is turning out to be more or less a database SQL app. I also posted C# examples from the OReilly "C# 7.0 in a Nutshell" book in order to learn it to possibly use the skills to apply for a related job in the Dev department at work.
October 19, 2018
I made better progress on the MTA based Express or Local App by using the .txt files found in a related forum post that the MTA didn't include in the initial .zip download. I also worked to include Browser Object and Document Object model ideas into the Stats section of the Visit Monaco website.
I also have been working on the "qt Beginner Guide" that's provided by the official documentation for qt in order to become more familiar with it so I can further help the Lubuntu team, and will include related examples in the Programming Book Progress section of the site. I also have started the "C# 7.0 in a Nutshell" OReilly book in hopes to possibly utilize the skills later for a related job at work. I also made further progress in the "Automate the Boring Stuff with Python" with more regex examples from Chapter 7.
October 12, 2018
I've made better progress with adding more Chapter 3 ideas for the Visit Monaco website by including Browser Object Model, and Document Object Model ideas, as well as solidifying object orientated scripts in the stats section. The MTA Express or Local app is taking longer than expected since I can't figure out how to interpret stop_id and train id values to make it feasible yet. I found two related projects, and messaged these devs for advice, and posted on the MTA Google + group. My Lubuntu D23 patch was finally committed by Wxl earlier this week, making my first contribution official. I also updated the programming projects section with more "Automate The Boring Stuff with Python" related chapter examples.
October 5, 2018
I updated the Visit Monaco website's stats sections with more arrays and object orientated programming ideas. I also have been making progress on the Express or Local App by displaying information about the first train in the dataset every 5 seconds in console. I hope to soon demystify the route id's and stop id's to make it clear where the current train actually is in relation to an actual NYC subway stop. I also did my first commit to Lubuntu's development, which can be found here:<br></br>https://phab.lubuntu.me/D23</li>
September 28, 2018
I have made more changes regarding object orientated JS ideas to the Visit Monaco website, and also uploaded my progress for the "Express or Local App". I have been actively trying to help the Lubuntu community with two related bugs using C++ and qt, as well as Python.
September 21, 2018
I have been making more progress on the Express or Local Python app with the help of IRC members from the #Python channel. I'm getting closer to being able to parse through the unbuffered .proto protocol data stream from MTA, and will work with it to make it useable. I've been also implementing more object orientated scripts for the "Visit Monaco" website as well.
September 14, 2018
I completed the Hockey Webscraper project, and it now runs at 1 PM every day on my Twitter account. I am now working on an MTA related Python app called "Express Or Local" that displays express or local train time, and will display user configured route settings. I also have been working on adding more JavaScript scripts to the "Visit Monaco" website.
September 7, 2018
With the help from some programmers on the #Python IRC channel, I have been able to run the Hockey Webscraper successfully. The only thing remaining for this project is to add team logos as another dictionary of .jpeg pictures within the same directory. I have also continued to make progress in "Automate the Boring Stuff with Python", and also have updated the "Visit Monaco" webpage with a few more scripts in the Stats webpage.
I have also been going back and forth on Beeware's Gitter page to get more help running the tests necessary for their open source project. I'm aiming to probably start learning GIT as well to help with this website.
August 31, 2018
I've been trying to figure out how to fix the "ftplib" Python module issue, and have been sending e-mails back and forth to the web hosting company to figure out the exact FTP directory I need to upload a link for the Hockey Webscraper. I've also ran a test suite for the Pybee open source project, and am going to run more specific tests for the project next week. I received the switches in the mail for the PODHD500 guitar pedal, so I'll post pics of how I fix it by soldering new switches within the next two weeks during a weekend. I also updated the Visit Monaco website with new scripts to include object orientated JavaScript programming as well.
August 24, 2018
I have been updating the Monaco website to include more scripts on the stats page. I am also becoming more active in Open Source projects such as BeeWare, and am working on running test scripts for this great open source project. I am also working on finishing the Hockey Webscraper to include an FTP link via the Twitter post that will be hosted from the webhosting provider's FTP service via Python's "ftplib" module, and using urllib3. I'm also going to be fixing the switches on my Line 6 PodHD500 multi-fx pedal this weekend, and will post related pics of the completed project.
August 17, 2018
I've been working on the Visit Monaco website, and to update the Hockey Webscraper to be placed on Twitter. The only issue at the moment is to account for Python's FTP module, and with the shared hosting server that this website is on. Once that is included, I can finally include Twitter posts with Excel .xlsx file attachments for the Hockey Webscraper. I also have been working on different Automating The Boring Stuff chapter projects which I will upload at a later time.
August 9, 2018
Uploaded Garden Menu Webscraper Twitter Bot, which basically scrapes the menu of a Brooklyn based restaurant, and reposts the link on Twitter for ease of use by customers. I also worked on the Monaco website this week as well.
August 6, 2018
I updated the Cat Of The Day Twitter Webscraper Bot to actually run at 1 pm every day! This took several attempts since the urllib module is very specific on how its methods are called. I am currently working on adding more features to the Monaco website, and am also working on making a menu webscraper Twitter bot that will actively scrape a Brooklyn based restaurant's PDF menu. I'm waiting for Twitter to approve this bot's account, but should be working soon.
August 3, 2018
I finally uploaded the results of the Hockey Webscraper, and am actively working on the fake website, "Visit Monaco, The Second Smallest Country In The World". I am also working on creating a "QC Auditor View" JavaScript project for work to help me and my fellow Quality team members while auditing our website to redisplay Ad ID's, and links to internal tools directly on each ad.
July 27, 2018
I am working on the "Visit Monaco, The Smallest Country In The World" website currently. I am making a lot of progress on the Hockey Webscraper at home, and am working on putting the results in an Excel .xlsv file so I can attach it to an e-mail, and send it to the user.
July 19, 2018
I finished the fake website, "The History Of MS Paint", and uploaded the results. I am still working on the Hockey Webscraper at home, and am attempting to use Selenium to automate tasks at work as well.
July 13, 2018
I am making progress on the Hockey Webscraper, but haven't posted the results yet. I created another fake website called "The History Of MS Paint" to demonstrate my skills learned from Chapters 1 & 2 for the HTML & JS books so far. I look forward to doing more project work before continuing in the actual books so that I can reinforce and demonstrate what I've learned so far.
July 9, 2018
Was incredibly sick for the last two weeks, but thankfully feel much better now. I have started working in the programming books at work to continue my progress, and will focus on the Hockey Webscraper first before revisiting the Song Of The Day Webscraper, since that will take more time to really find a solution via Repl.it
June 15, 2018
Still trying to find a capable Python based synth that will work in Repl.it to convert the MIDI sample to WAV for the Song Of The Day project. Made small progress this week due to sickness this week.
June 8, 2018
Updated overall website design, looks good so far. Still trying to make the Song Of The Day Webscraper actually work on its iFrame webpage. Cat Of The Day Webscraper still works, and I'm making progress on the Hockey Webscraper. I might shift focus to purely implementing the MIDI to Audio conversion for the Song Of The Day Webscraper though.
June 1, 2018
Updated the Song Of The Day Webscraper with my current version. It's still not working because I haven't figured out the MIDI to audio conversion process within the same tab. However, its getting closer since I'm using "FluidSynth" to do this, as well as the Midi2Audio Python library, and e-mailed the library's creator with questions.
May 25, 2018
Updated background color to light purple on all webpages. Fixed Python script for Cat Of The Day Webscraper. Still working on making Song Of The Day Webscraper functional without use of MIDI, and currently researching MIDI to WAV converters to allow the user to play it in browser. Will also update guitar section with actual step by step process on how I will fix my Fender Jaguar guitar's input jack with soldering since it's my first time doing so. Wish me luck!
May 18, 2018
Adjusted all web pages to include mobile based viewport meta tag to allow for easier viewing on mobile devices.
May 17, 2018
Adjusted styling on Python programming project webpages, as well as adjusted width of iFrame for scripts.
May 16, 2018
Added my Python programming projects, Song Of The Day Music Webscraper, and Cat Of The Day Twitter Webscraper to the Python projects section. Need to fix the errors in the Repl.it verison of the Music Webscraper.
May 15, 2018
Adjusted more styling on all Musimatic webpages to appear more consistent. Will think of cool ways to add JavaScript code as well.
May 14, 2018
Adjusted styling on Musimatic's various webpages to include bigger headers
May 11, 2018
Fixed script issues on Collar website for Spin To Win webpage, and adjusted styling consistently throughout the website. It's complete, and I like how it looks with Bootstrap. I tried adding a dropdown menu nav bar with Bootstrap, but this is not necessarily working out easily. Will try again next week.
May 4, 2018
Adjusted styling of old fake website, "Slings", to appear more consistent. Also updated "Collar" with a Profiles webpage this week.
April 27, 2018
Updated the website to include consistent styling, and uploaded the actual fake websites with their current state of progress. Looks good so far.